Closed mjs513 closed 1 year ago
As a follow up believe it related to a clock stretching issue with the BNO080: https://github.com/adafruit/Adafruit_CircuitPython_BNO08x/issues/33
Will try a i2c1 = bitbangio.I2C(board.I2C_SCL1, board.I2C_SDA1, timeout = 500) next time I have it set up
There are known problems with esp32-s3 in i2c as well, which we are tracking. But it's true, the BNO chips have some interesting I2C behavior as well.
Yeah. Was doing some research this morning and seems to be a known problem. Unfortunately also happening on the RP2040, Teensy Micromod and Teensy 4.1 as well.
Early on in the development of the Teensy 4 we had similar issues with the BNO055 and BNO080 and required a changed to I2C. For ref here was the fix = at least for the teensy in i2c: https://github.com/PaulStoffregen/Wire/pull/16/commits/08d870fc130b741e4fdee38798c64a28949c853d
Maybe a clue for you all to look at
Will re-test after pending S3 I2C fixes are added to ESP-IDF v4.4.x.
I retested with a BNO055 on Feather ESP32-S3 with the latest build, and it still fails. It works fine on ESP32-S2, and also with bitbangio.I2C()
. This may have the same root cause as #6311.
Pushing this forward to 8.x.x because I don't see an upstream fix in sight.
The BNO0xx sensors are known to violate the I2C bus protocol under certain conditions. I did some testing of the BNO055 here: https://github.com/adafruit/Adafruit_CircuitPython_BNO055/issues/115. I expect similar results with the BNO080. Perhaps we can make the library do some error recovery, but given that the BNO sensors themselves are flaky, I'm not sure we should characterize this as a bug that we expect the ESP-IDF to fix. The ultimate bug is in the BNO sensors themselves.
I will close this as "can't fix" in the core for now. If you disagree let me know. I will add some warnings to the BNO080 learn guide, and have opened this library issue: https://github.com/adafruit/Adafruit_CircuitPython_BNO08x/issues/41
I retested after we upgraded to ESP-IDF v5.1 in CircuitPython 9.0.0-alpha.2. BNO055 still does not work. This is not so surprising. I added warnings to the appropriate BNO guides a while ago.
CircuitPython version
Code/REPL
Behavior
When working but if I try to add a BNO080 (Adafruit or Sparkfun) it reboots the ESP32S3 and seems to hang. No error messages
Description
Using a I2c test board that we used for testing the Teens 4.1: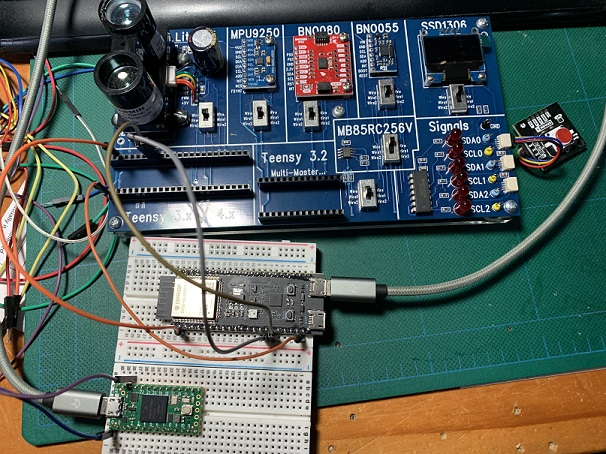
I attached the ESP32S3. All worked well until I tried to add the BNO080 to either I2c or I2c1 bus (Arduino verbiage Wire or Wire1). When I add the BNO080 it reboots the ESP32S3 and seems to hang Mu edit and I have to power cycle to get it running again. No error messages appear.
If I run the same script using CircuitPython on the T4.1 it picks up all devices including the BNO080. I do have a QT PY RP204 on order to test it with that as well.
Additional information
No response