Closed lawsonxwl closed 2 years ago
Can you please try with --method scorecam or --method ablationcam and post the results?
Grad-cam seems to give bad results for the swin-transformer.
The result is very sensitive to the target_layer choice.
For the swin_base_patch4_window7_224
model,
This target_layer seems to give reasonable results, give it a try:
target_layer = model.layers[-1].blocks[-1].norm2
Closing the issue for now, please re-open if the suggestion above doesn't help.
My code: `if name == 'main': """ python swinT_example.py -image-path
Example usage of using cam-methods on a SwinTransformers network.
My heatmap:
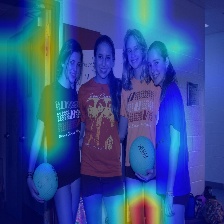
Is there something wrong with my code?