Closed Mukund-Tandon closed 1 year ago
the solution is the author of package jwt
change import to from collections.abc import Mapping
for python 3.10
P.S: i checked it seems they updated the package now this package need to bump PyJWT version @mattupstate
@Mukund-Tandon, same here
Same
same, any solutions?
I ran into this problem as well. I was using Python version 3.10.4 at the time.
I saw some other people having the same issue on Stackoverflow: https://stackoverflow.com/questions/69381312/in-vs-code-importerror-cannot-import-name-mapping-from-collections
The top rated solution (credit to John R Perry and Geremia) was to change any instance of:
from collections import Mapping
to
from collections.abc import Mapping
.
This may not be backwards compatible to older versions, so I think one should handle it via a try except block ( I copied some code from the urllib3 and Flask-RESTful library that had code which handled this ImportError case ).
try:
from collections.abc import Mapping
except ImportError:
from collections import Mapping
Credits to @Aazerra for having the answer before I found this thread.
EDIT 11/28/2022:
On further inspection, this package is referencing python package jwt: https://github.com/jpadilla/pyjwt. They have already fixed this problem, so the dependecy for pyjwt needs to be updated to address the issue for this package.
It is recommended to update pyjwt
to 1.7.1
The pyjwt version of the directly downloaded flask-jwt is 1.4.1 Will trigger:
ImportError: cannot import name 'Mapping' from 'collections'
If you use the latest pyjwt Will trigger:
return jsonify({'access_token': access_token.decode('utf-8')})
AttributeError: 'str' object has no attribute 'decode'
I solved this by installing a new version of pyJWT as @tdxs8848 mentioned in his answerd.
pip install PyJWT --upgrade
For flask-jwt
it would probably, I'll suggest to upgrade this dependency and changing the requirements.txt
I'm still facing the same issue? Is this issue resolved yet? I can still see an older version of PyJWT being used in the requirements.txt https://github.com/mattupstate/flask-jwt/blob/c27084114e258863b82753fc574a362cd6c62fcd/requirements.txt#L2
Also, I don't know why this PR suggesting the change was closed without merging it in? https://github.com/mattupstate/flask-jwt/pull/151
What's the best way to solve this now?
Using Python 3.10
Complete error-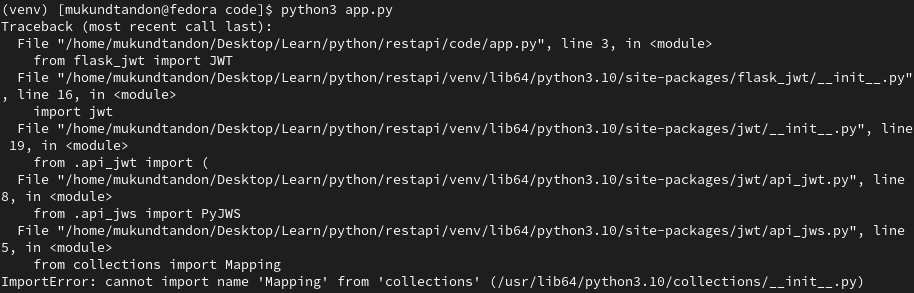
app.py -