Closed Im-Oab closed 3 years ago
That's very strange 🤔
The artifacts you're seeing look like they could be a result of stuff overlapping/bleeding into eachother in the font atlas, but that's not meant to be possible - everything gets laid out with a 1px border to avoid that:
Which font are you using? If it's a free one, I can possibly try to replicate the issue on my end.
I use D-DINCondensed font.
I've not been able to replicate the issue yet, but I did find a small problem with the font atlas code (it wasn't including the padding I previously mentioned after a texture resize took place). I've pushed a fix for that issue to the main
branch - could you try running your code with that and see if it fixes your problem (or at least improves it)?
[dependencies]
tetra = { git = "https://github.com/17cupsofcoffee/tetra", branch = "main" }
If not, some other questions that might help me replicate the issue:
Canvas
/ScreenScaler
, or directly to the screen?16.0, 16.0
) or is it offset by fractional pixels (e.g. 16.5, 16.5
)?It's not fixed when I changed to the main branch but when I use ceil() with the position that passes to DrawParam. This issue disappears.
Thank you. :)
No problem! The text rendering code does some subpixel/anti-aliased rendering stuff which can look a bit weird if you draw off the pixel grid, but I've never seen it cause artifacts like this before.
Summary: continuous changing text using set_content() has a chance to cause glitching on display text. (acttached images)
Steps to reproduce:
Expected behavior:
Additional info:
tetra 0.5.7, OSX Catalina
Screenshots: // This happen after call set_content() several times.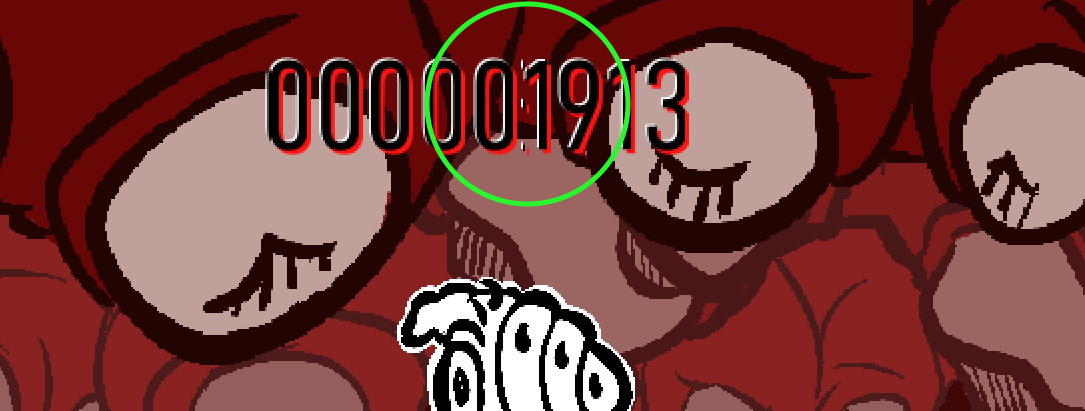
// This text call set_content() once but show it later. Between setup and the time it draws on screen. The game call set_content() on another text object several times.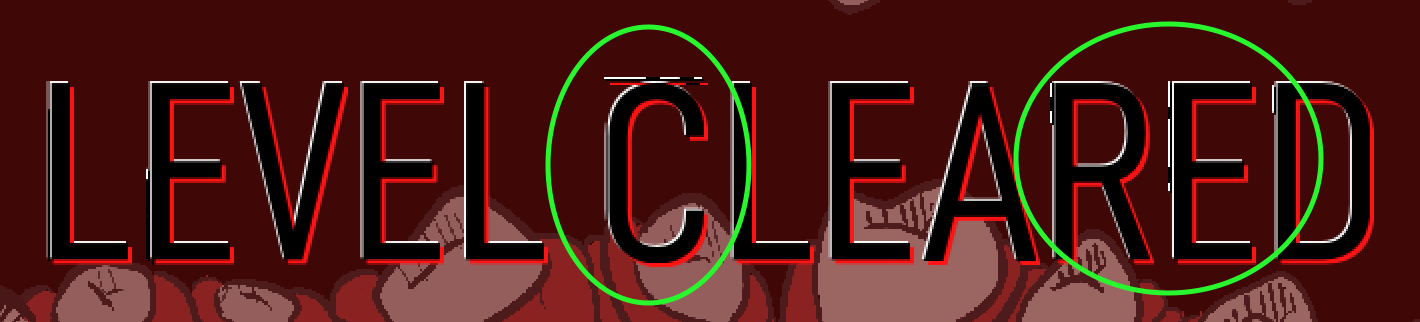
Codes: `
`