Open BodoMinea opened 3 years ago
Hello! Did you ever get this issue resolved? I have a very similar problem I think. I have a single 1/16 P5 64x32 panel which works fine using an Arduino Mega 2560 following the guide on https://github.com/adafruit/RGB-matrix-Panel. But I want chaining of multiple panels so I have wired it to a D1 mini clone as per instructions on https://github.com/2dom/PxMatrix. The pattern test runs mostly ok but the "pixeltime" test has serious ghosting. AFAIK the wiring is correct. Any ideas?
Hi, the mentioned matrix works properly with another and different wiring - try using this https://github.com/pixelmatix/SmartMatrix
The wiring should be documented as a comment in the corresponding example sketches or you can check out some more details in this project - https://esp32.com/viewtopic.php?f=17&t=3188 or on the library wiki http://docs.pixelmatix.com/SmartMatrix/library.html
You can also check out some discussions on the library forum - https://community.pixelmatix.com/ I got multiple types of non standard panels with the help of folks over there.
Good luck with your project!
Hi everyone,
I have this panel - https://www.aliexpress.com/item/32998526924.html bought from Aliexpress that I can easily control with an Arduino Mega 2560 following the guide on Adafruit and also using their library - https://learn.adafruit.com/32x16-32x32-rgb-led-matrix/connecting-with-jumper-wires / https://github.com/adafruit/RGB-matrix-Panel
It works with their default configuration and examples, but only one 64x32 matrix (no chaining).
I am also able to correctly control it with the default settings in @hzeller´s https://github.com/hzeller/rpi-rgb-led-matrix With that, chaining also works (even with 6 of them).
Now, here are my results with PxMatrix.
1. Post your code The code is probably NOT the issue. I can use it with other LED matrix panels I have (a P4 64x32 for example), but anyway.
2. Post a picture of the problem and describe what you expect to see With the code above, I currently see this: (and the scrolling text shows up as white and very noisy).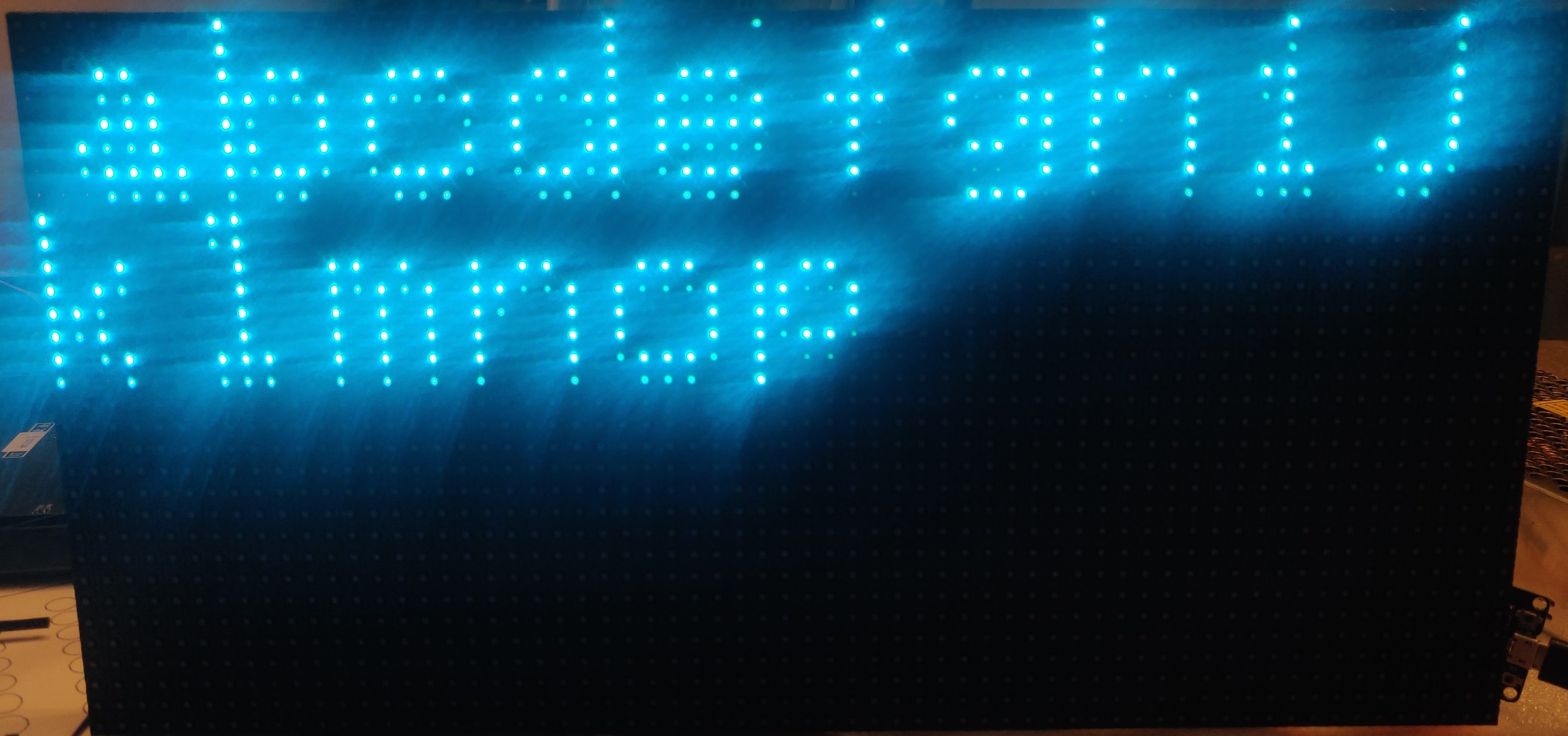
If I uncomment the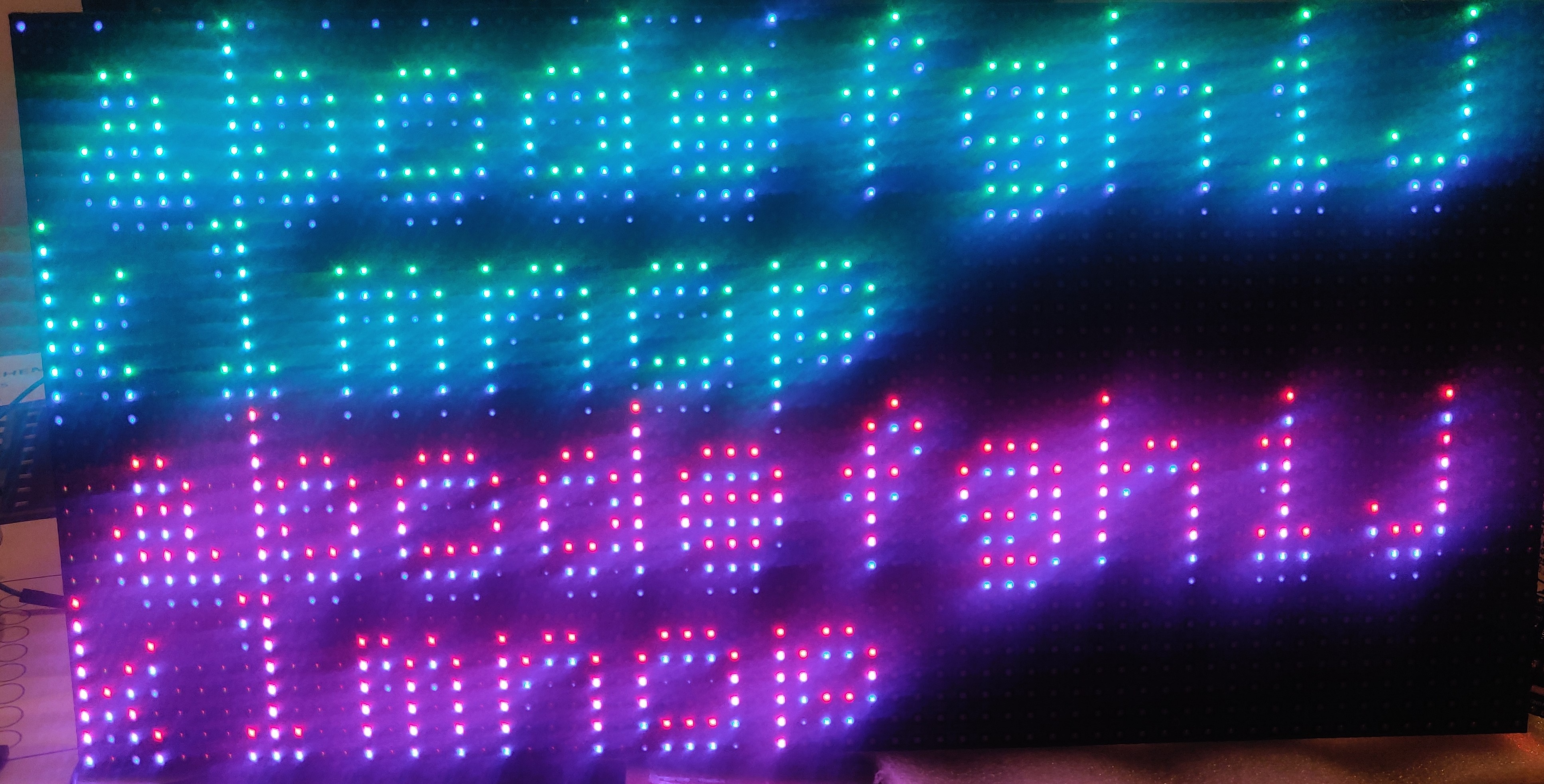
display.setPanelsWidth(2);
and chain another panel (ESP32 to Panel Input of matrix 1, from there to panel output of matrix 2 and a ribbon connecting all pins of panel output 1 to panel input 2), this is the result - still only the first (ESP32 directly connected) panel lights up, and it displays like this:Seller instructions say it is a 1/16 scan module, but I tested to see how it looks with 1/8 just to be sure (no chaining, only one module):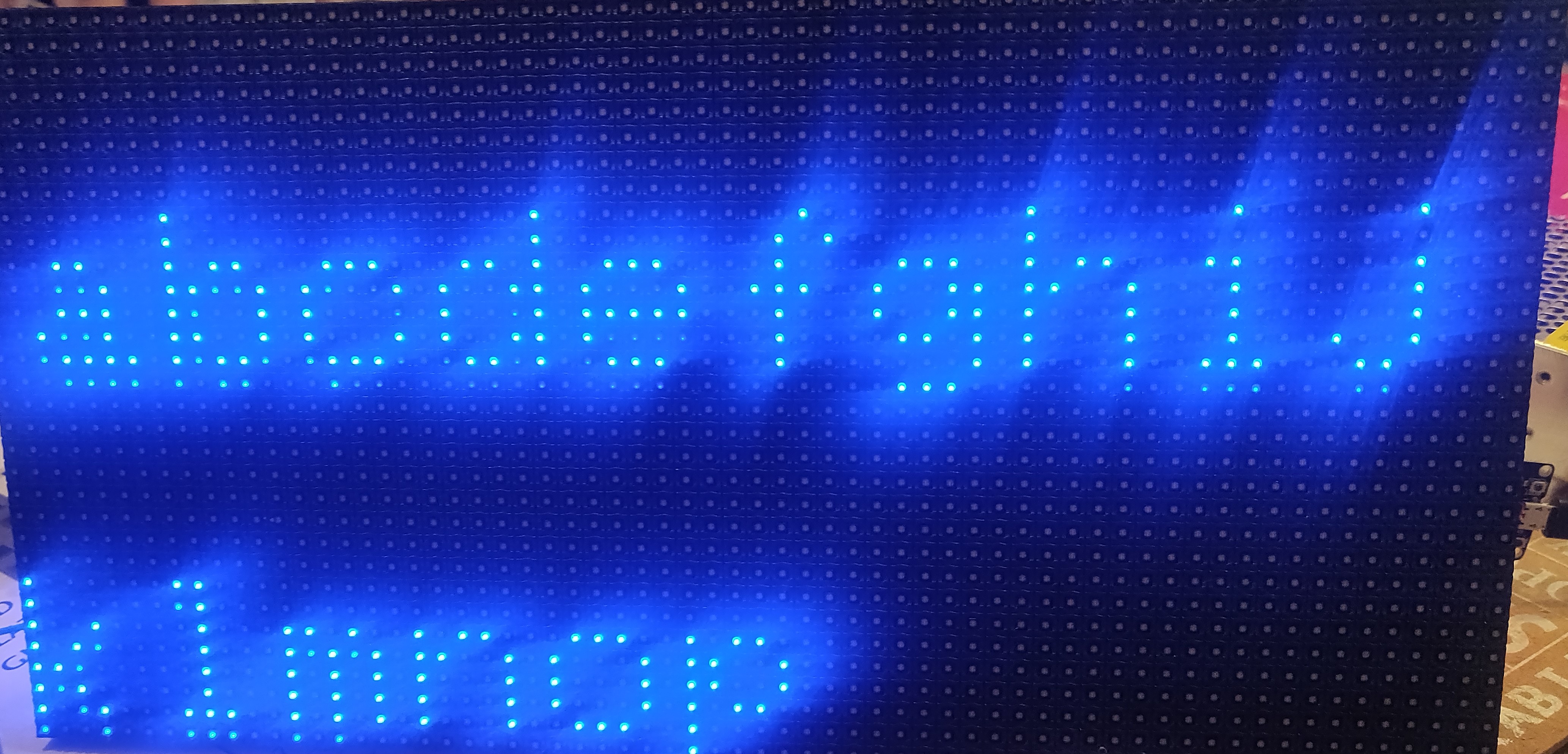
3. Run the pattern test and post the result as gif/video (need to have a bit more than one full RED/YELLOW/WHITE cycle)
1/16 pattern test (it loops after WHITE) - please note that the GIF skips some frames, but the pattern test fully runs, smoothly. Here it also doesn't have the noise issues I get on the pixeltime sketch:
1/8 pattern test (it stops/locks up after WHITE):
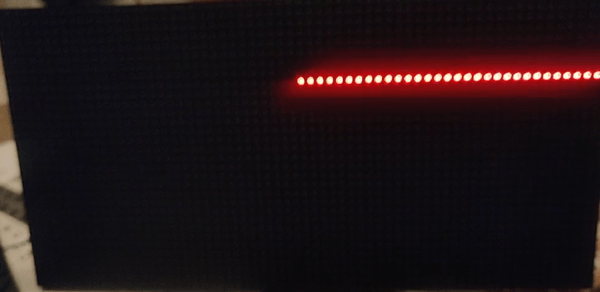
During both tests only one module is connected.
4. State what version of PxMatrix you are running and what MicroController you use Latest PxMatrix with ESP-WROOM-32. The wiring is the standard one from the project homepage, with some of the PI wires hooked up to the PO and the rest to the ESP32 via jumper cables. I have tried swapping out cables, boards, re-setting-up and recompiling everything on ESP8266, the results are the same, can't get it to display correctly.
Any ideas are appreciated. Happy holidays!