Closed intelviper closed 1 year ago
Solved this by adding below code in line #856
if (_scan_pattern==ZIGZAG)
bit_select = 7-bit_select;
This solved the flipped first row. And now all examples and code are working fine.
I even managed to use this library in https://github.com/donnersm/FFT_ESP32_Analyzer and made it to work with this.
I connected P10 1/4 Scan 3535 RGB LED Matrix as per connection diagram.
When I print text, the top half gets rotated in ZIGZAG Mode and is then pushed into display. Attached Pic/Pattern test video.
Any help would be much appreciated. Thanks!
Rules
Post a picture of the problem and describe what you expect to see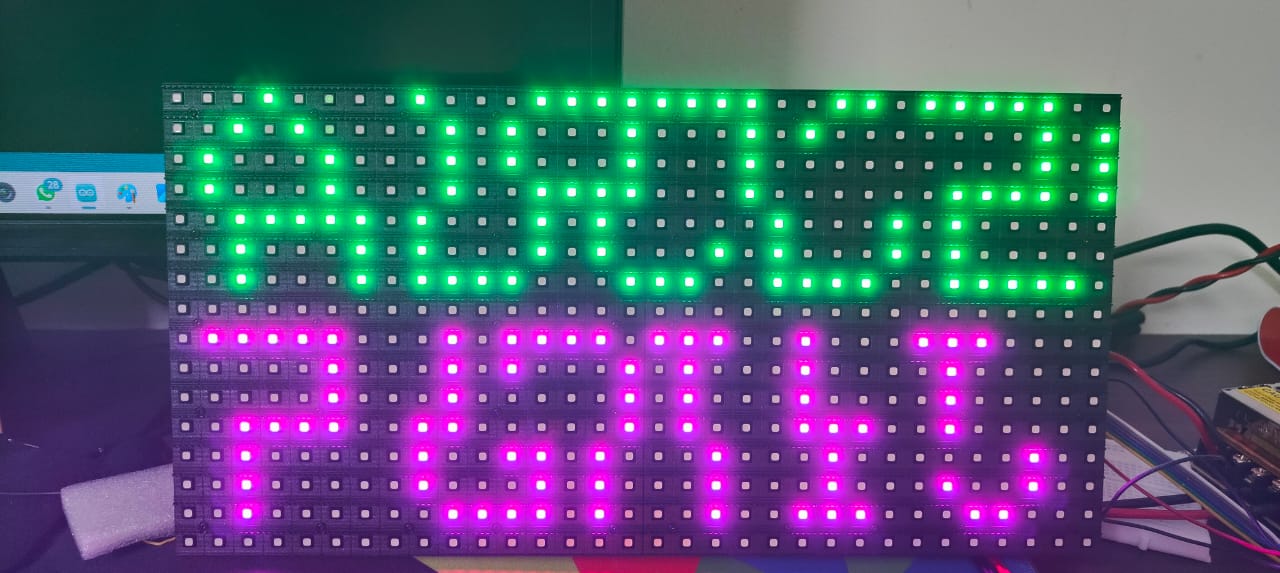
Run the pattern test and post the result as gif/video (need to have a bit more than one full RED/YELLOW/WHITE cycle)
https://user-images.githubusercontent.com/27574370/227803845-302df194-88a0-4af0-be6b-dc546c294ba2.mp4