In the context of a framework, a component’s lifecycle is a collection of phases a component goes through from the time it is appended to the DOM and then rendered by the browser (often called mounting) to the time that it is removed from the DOM (often called unmounting).
Each framework names these lifecycle phases differently, and not all give developers access to the same phases.
All of the frameworks follow the same general model: they allow developers to perform certain actions when the component mounts, when it renders, when it unmounts, and at many phases in between these.
The render phase is the most crucial to understand, because it is repeated the most times as your user interacts with your application. It's run every time the browser needs to render something new, whether that new information is an addition to what's in the browser, a deletion, or an edit of what’s there.
This diagram of a React component's lifecycle offers a general overview of the concept.
Mounting
The constructor is only called when an instance of the component is created and inserted in the DOM. The component gets instantiated. That process is called mounting of the component.
constructor(props)
It is called when the component gets initialized.
You can set an initial component state and bind class methods during that lifecycle method.
constructor(props) {
super(props);
this.state = {date: new Date()};
}
render()
This lifecycle method is mandatory and returns the elements as an output of the component.
The method should be pure and therefore shouldn’t modify the component state.
It gets an input as props and state and returns an element.
componentDidMount()
It is called only once when the component mounted.
That’s the perfect time to do an asynchronous request to fetch data from an API.
The fetched data would get stored in the internal component state to display it in the render() lifecycle method.
Updating
What about the update lifecycle of a component that happens when the state or the props change?
render()
This lifecycle method is mandatory and returns the elements as an output of the component.
The method should be pure and therefore shouldn’t modify the component state.
It gets an input as props and state and returns an element.
componentDidUpdate(prevProps, prevState)
The life cycle method is immediately invoked after the render() method.
You can use it as opportunity to perform DOM operations or to perform further asynchronous requests.
Unmounting
componentWillUnmount()
It is called before you destroy your component.
You can use the lifecycle method to perform any clean up tasks.
What is Component's LifeCycle
Reference: LifeCycle
mounting
) to the time that it is removed from the DOM (often calledunmounting
).render phase
is the most crucial to understand, because it is repeated the most times as your user interacts with your application. It's run every time the browser needs to render something new, whether that new information is an addition to what's in the browser, a deletion, or an edit of what’s there.This diagram of a React component's lifecycle offers a general overview of the concept.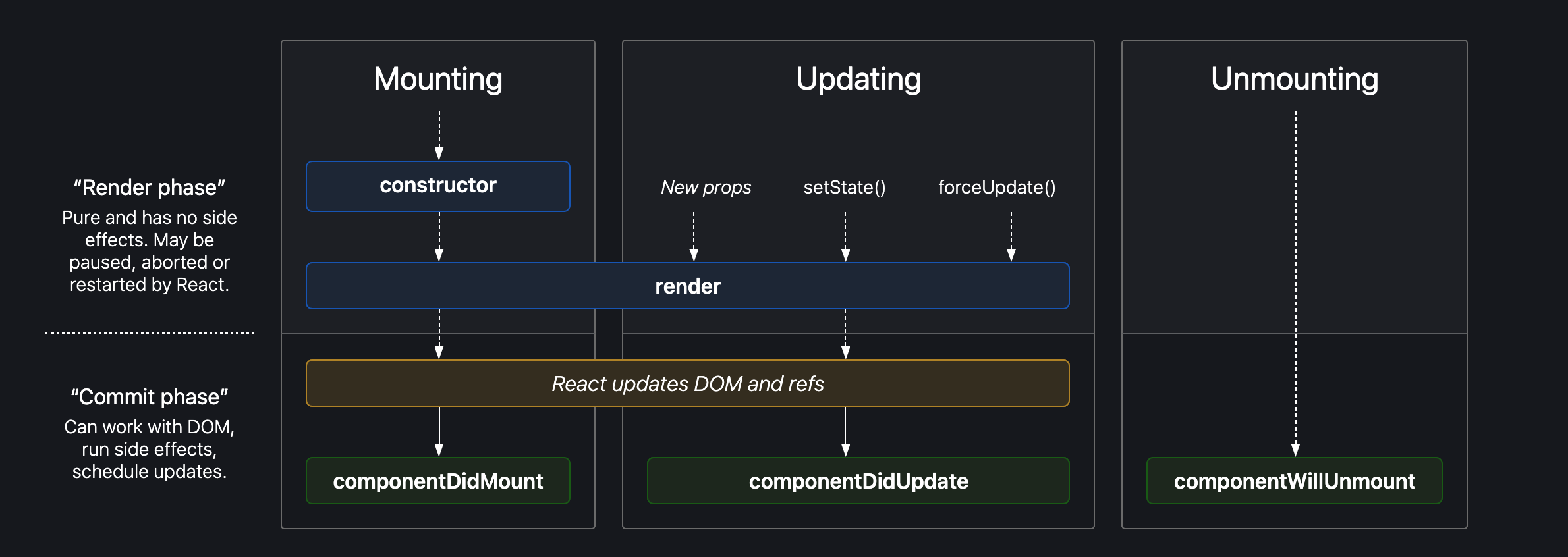
Mounting
The constructor is only called when an instance of the component is created and inserted in the DOM. The component gets instantiated. That process is called mounting of the component.
constructor(props)
render()
state
.props
andstate
and returns an element.componentDidMount()
Updating
What about the update lifecycle of a component that happens when the state or the props change?
render()
state
.props
andstate
and returns an element.componentDidUpdate(prevProps, prevState)
render()
method.Unmounting
componentWillUnmount()