Closed douglascomet closed 8 months ago
Thanks for the report!
I added a simple test script to the bug report
fwiw, I was able to get around this issue by adding gaps programatically and skipping empty tracks
# Filter empty tracks because
# if the top track is empty then it will create an empty track after flattening
video_tracks = list(filter(None, timeline.video_tracks()))
rate = timeline.global_start_time.rate
end_frame = timeline.duration().to_frames() - 1
end_time = otio.opentime.from_frames(end_frame, rate)
# Iterate through video tracks and determine which do not end with a child and append a gap
for index, track in enumerate(video_tracks):
result = track.child_at_time(end_time)
if result:
msg = 'Skipping adding gap to the track because' \
' it has a child at end time of the timeline.'
print(msg)
continue
# Get time range of last child in track
# Tracks are essentially lists of children reading left to right based on their
# sequential order in the track
source_range = track.range_of_child(track.children_if()[-1])
print('Track {}\'s last child\'s source_range {}'.format(index, source_range))
# The gap will be inserted after the last child's duration,
# so calculate that frame by adding the last child's start frame and its duration
gap_start_frame = source_range.start_time.to_frames() + source_range.duration.to_frames()
print('Start frame for the gap to be created {}'.format(gap_start_frame))
# Determine time range from start and end frame for the gap
duration = end_frame - gap_start_frame + 1
start_time = otio.opentime.RationalTime(gap_start_frame, rate)
duration_time = otio.opentime.RationalTime(duration, rate)
gap_source_range = otio.opentime.TimeRange(start_time=start_time, duration=duration_time)
print('Time Range of gap: {}'.format(gap_source_range))
# Create gap and append to the track
gap = otio.schema.Gap(source_range=gap_source_range)
track.append(gap)
one_track = otio.algorithms.flatten_stack(video_tracks)
Came across this problem myself, where a "lower" track that was the last clip on the timeline and was missing from the result of otio.core.flatten_stack
, but your workaround @douglascomet did work for me, thank you!
Fixed by https://github.com/AcademySoftwareFoundation/OpenTimelineIO/pull/1703
@douglascomet can you double-check that this fix works for your case?
Bug Report
Repro test
C++:
Python:
If the stack is flipped (such that the track with two clips is above the track with one, then it functions as expected.
(original bug report follows below)
Incorrect Functionality and General Questions
When using
flatten_stack
if a video track does not end with a clip or gap above another video track that does the child from the bottom video track will be omitted from the returned timeline.To Reproduce
Expected Behavior
flatten_stack
should insert a gap prior to flattening or account for this scenario when flattening so the clip on the bottom track is accounted for and included in the returned timelineScreenshots
Initial results, the top image from otioview is the original timeline and the bottom image from otioview is the result of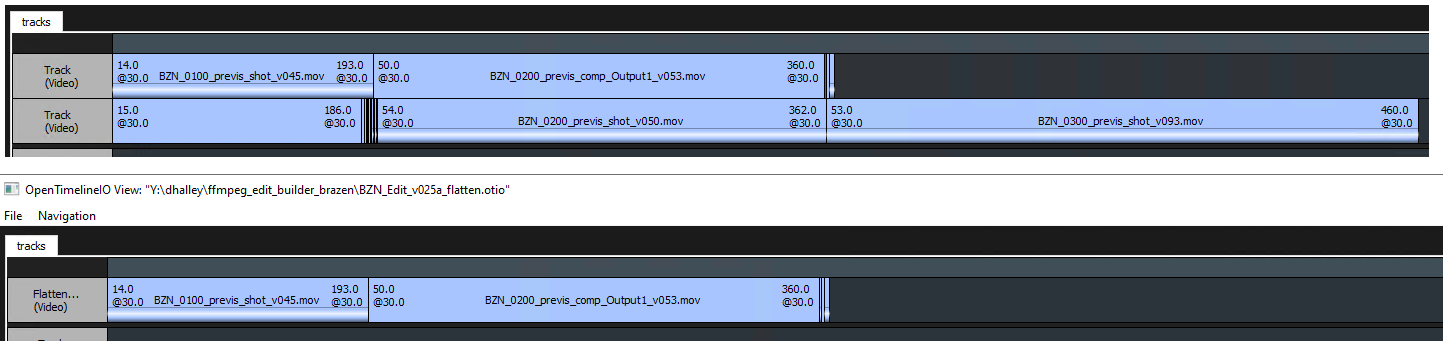
flatten_stack
Tested this by inserting a new clip into the above track and re-ran
flatten_stack
and got the same result where the end of the bottom clip is being omitted from the returned timeline.