Open hkchengrex opened 4 years ago
I like the output of 0.4 more though, guess I will compute the centroid again in numpy for now.
Thanks for the report. I'll have a look on the version you mentioned. (it may take time though, because of the ongoing issue in my country😭)
Stay safe! I am in Hong Kong so 👀
Hello @Algy As I understand the problem with centroid is still available. Can you advise me a fast solution to calculate it by myself? Currently I'm using regionprops from skimage, but it's too slow
Hello @Algy ,
Thanks for this library, it is really fast and does the job very well.
I think that I met an issue that could be connected to the centroid one. If I show the image obtained by coloring every pixel of original image with the color of the assigned cluster I get the colors all shuffled up. I think that the indices of the clusters do not match the indices in the assignment matrix.
PS I checked the color space and it is not a problem of color conversion.
Any progress on the problem? I encounter this problem too.
You can bypass the problem by reassigning the index at very little cost, would still be faster if fixed in the C++ code probably.
for entry in slic.slic_model.clusters:
y, x = entry['yx']
idx = assignment[int(y),int(x)]
x_centroid_map[assignment==idx] = x
y_centroid_map[assignment==idx] = y
Thank you for this great piece of work!
I spotted an issue in the latest version (0.4.0), that the centroid information returned by
slic.slic_model.clusters
is incorrect.Minimal working example:
I would expect the (x, y) centroid map to look like this: X:
Y:
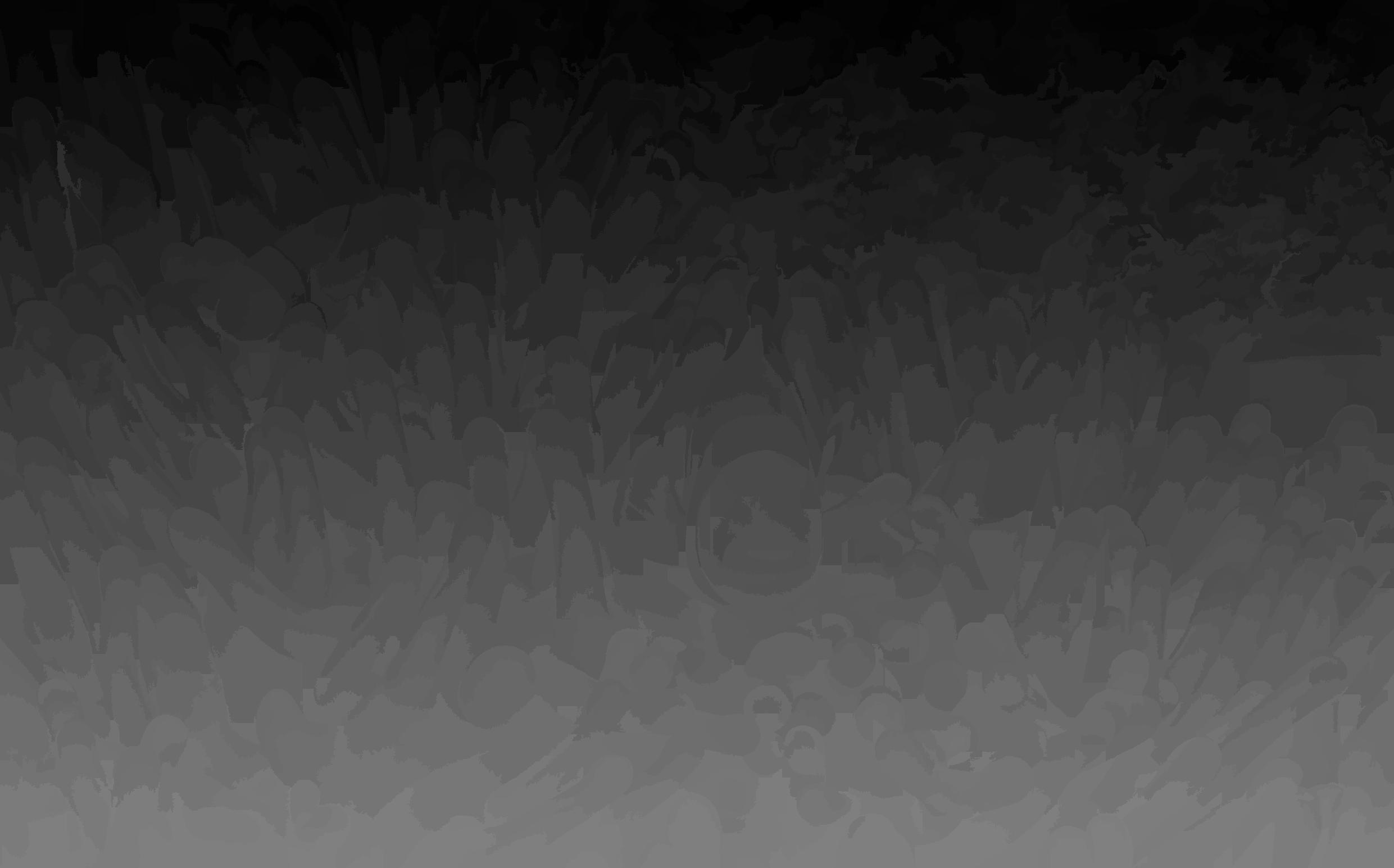
But it gives me this for X:
and Y is fine.
Note that this works in version 0.3.5 (which I used to generate the "expected output")!
Hope this helps :smile: