Closed mameolan closed 2 years ago
@maxkatz6 what was the solution? I'm trying to make PSAvalonia asynchronous by running avalonia inside of a powershell runspace, but I'm getting a black window
Going to try to run it without using the runspace to see if that makes a difference.
It's also possible that I'm using the wrong rendering libraries for my platform (I'm having to install the dependencies manually at the moment). I'm running on Win x64
EDIT:
I'm downloading the packages from nuget as .zip files then loading the assemblies using this manifest:
{
"System.ComponentModel.Annotations" : {
"target" : "net461", //name of the folder within package/lib/ to search for assemblies
"assemblies" : [ "*.dll" ] //glob patter of assemblies to look for in the file above
},
"JetBrains.Annotations" : {
"target" : "net20",
"assemblies" : [ "*.dll" ]
},
"Avalonia.Remote.Protocol" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"System.Buffers" : {
"target" : "net461",
"assemblies" : [ "*.dll" ]
},
"System.Numerics.Vectors" : {
"target" : "net46",
"assemblies" : [ "*.dll" ]
},
"System.Runtime.CompilerServices.Unsafe" : {
"target" : "net461",
"assemblies" : [ "*.dll" ]
},
"System.Runtime.InteropServices.WindowsRuntime" : {
"target" : "net45",
"assemblies" : [ "*.dll" ]
},
"System.Threading.Tasks.Extensions" : {
"target" : "net461",
"assemblies" : [ "*.dll" ]
},
"System.Reactive" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"System.Memory" : {
"target" : "net461",
"assemblies" : [ "*.dll" ]
},
"System.Runtime.InteropServices.RuntimeInformation" : {
"target" : "net45",
"assemblies" : [ "*.dll" ]
},
"System.ValueTuple" : {
"target" : "net461",
"assemblies" : [ "*.dll" ]
},
"Avalonia" : {
"target" : "net461",
"assemblies" : [ "*.dll" ]
},
"Avalonia.Native" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"HarfBuzzSharp.NativeAssets.Win32" : {
"natives" : [ "*.dll" ] //glob of the native .dll to load as found in package/runtimes/[platform]/native/
// These get copied over to a natives folder in the root of my repo so that they are all in the same place
// - Just the native .dlls are copied to that natives folder. The managed .dlls are loaded in-place from within the extracted nuget package zip file
},
"HarfBuzzSharp" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"SkiaSharp.NativeAssets.Win32" : {
"natives" : [ "*.dll" ]
},
"SkiaSharp" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"Avalonia.Skia" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"System.Drawing.Common" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"Avalonia.Win32" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"Avalonia.Desktop" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
},
"Avalonia.Markup.Xaml.Loader" : {
"target" : "netstandard2.0",
"assemblies" : [ "*.dll" ]
}
}
Here's the powershell script for running Avalonia Asynchronously (This doesn't use PSAvalonia, but I'm planning on porting it) to resolve https://github.com/ironmansoftware/psavalonia/issues/6
# Create new runspace
$Runspace = [Management.Automation.Runspaces.RunspaceFactory]::CreateRunspace( $Host )
# Initialize the runspace object
# - Some Windows Forms and WPF controls are only STA
$Runspace.ApartmentState = 'STA'
$Runspace.ThreadOptions = 'ReuseThread'
$Runspace.Open() | Out-Null
# Add the window tracker to the Runspace environment
# - Makes the windows usable inside the runspace
$Runspace.SessionStateProxy.SetVariable( 'Avalonia_Wins', $Avalonia_Wins ) | Out-Null
# - To access the application object from inside the runspace:
# - use [Avalonia.Application]::Current
# Create a new PowerShell object (a Thread)
$PowerShellRunspace = [System.Management.Automation.PowerShell]::Create()
# Initialize the PowerShell object with the runspace
$PowerShellRunspace.Runspace = $Runspace
$PowerShellRunspace.AddScript({
# Set platform-specific services on the main loop
$AppBuilder = [Avalonia.AppBuilder]::Configure[Avalonia.Application]()
$AppBuilder = [Avalonia.AppBuilderDesktopExtensions]::UsePlatformDetect( $AppBuilder )
# Set the lifetime for the applicaiton
$LifeTime = [Avalonia.Controls.ApplicationLifetimes.ClassicDesktopStyleApplicationLifetime]::new()
$LifeTime.ShutdownMode = [Avalonia.Controls.ShutdownMode]::OnExplicitShutdown
$AppBuilder = $AppBuilder.SetupWithLifetime( $LifeTime )
# Set the Avalonia main loop
$App = $AppBuilder.Instance
$Window = [Avalonia.Markup.Xaml.AvaloniaRuntimeXamlLoader]::Parse[Avalonia.Controls.Window](@"
<Window xmlns="https://github.com/avaloniaui"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="800" d:DesignHeight="450"
Width="800" Height="450"
Title="Test">
<StackPanel>
<Button Width="160" Name="button">My Button</Button>
<TextBox HorizontalAlignment="Left" Margin="12,12,0,0" Name="txtDemo" VerticalAlignment="Top" Width="500" Height="25" />
</StackPanel>
</Window>
"@)
$Window.Show()
# Set application lifetime via cancellation token (required for cross-platform dev)
$CancellationTokenSource = [System.Threading.CancellationTokenSource]::new()
# Run the main loop
[Avalonia.Controls.DesktopApplicationExtensions]::Run( $App, $CancellationTokenSource.Token )
}) | Out-Null
$PowerShellRunspace.BeginInvoke() | Out-Null
Well I think I notice an issue with my dependencies. From the build files of PSavalonia, it looks like my implementation of Avalonia in PowerShell is missing Avalonia.Angle.Windows.Natives
This missing dependency seems to be the culprit. According to https://github.com/AvaloniaUI/angle/, that native library is the implementation of Google's Angle in Avalonia. I'm shocked that Avalonia isn't erroring out when this library is missing.
Though, timeline-wise there could be another issue. The last fix for Avalonia.Angle that should have affected my imp was in February of this year: https://github.com/AvaloniaUI/Avalonia/pull/10204
This issue was closed on June 18th of last year, so whatever solution was implemented here was not resolved by that pull request, but rather by something else
Ok, fixing the dependency, fixed that problem, but... new problem, my window is transparent :/
From an initial search, it looks like the problem is caused by missing an application theme
https://github.com/AvaloniaUI/Avalonia/search?q=invisible+window&type=discussions
Will need to figure out how to add the theme dynamically using powershell...
EDIT: this may be a good starting point: https://github.com/AvaloniaUI/Avalonia/issues/2824
Ok. translating this into powershell, and using the sources that are used in Application.Styles
for PSAvalonia, I got Avalonia to work in my own implementation of Avalonia in PowerShell:
Screenshot of it working now:
@smartguy1196 Care to share a solution? Sorry for pinging you here but I'm interested in what you have done for your own Avalonia PowerShell implementation and your PSAvalonia fork has disabled issues and discussions.
@ALIENQuake I'm working on it. The fork was just to get PSAvalonia to upgrade from 0.8.x to 0.10.x
My imp will be hosted from its own repo, as it was built from the ground up, and doesn't just support Avalonia, but WPF, and WinForms. I used PSAvalonia as a reference when I would come across bugs in my imp. I'll have the repo uploaded before the end of the week
My implementation runs Avalonia/WPF/WinForm asynchronously in pure PowerShell instead of synchronously in pure C# like PSAvalonia does. Writing your own Async Imp is actually pretty simple to setup, especially if you are just looking to use WPF/WinForms, but I'll have mine up shortly.
You setup the Application instance inside of a PowerShell Runspace. While in the runspace, share the System.Window.Threading.Dispatcher Object for the runspace with the the host runspace (I use a hashtable stored on a sessionproxy variable).
Then call the Dispatcher from the host runspace thread to run code on the application's runspace/thread.
@ALIENQuake see https://github.com/pinuke/PWSH-AGUI/blob/main/samples/simple-window-creation/sample.ps1
https://github.com/pinuke/PWSH-AGUI (Powershell Asynchronous Graphical User Interface) is very much in a pre-release state right now, as there is still work to be done.
The actual scripting bit is pretty much done. I still have to add code to support closing/disposing of all of the resources, but the code for runspace, application, and window creation is already written, and I would like to do a bit of rewriting to format the script as a powershell module.
Forgive the file-splitting (I prefer to practice IoC in every language when possible). I do plan to serialize and simplify the entire script so that I can educate others on how to write something similar. For now, to navigate the code, clone with:
git clone https://github.com/pinuke/PWSH-AGUI
and use ctrl+shift+f to find definitions for cmdlets and the like.
I'm very active on the project, as I intend on using this repository within my other projects. So, don't be startled by a lack of a README.md.
@pinuke Very nice! Thanks! I will look at it and try to provide feedback directly inside repo.
@ALIENQuake one issue you may run into, is my dependency loader (PowerLoader) is currently hard coded for win-x64.
I have plans to make it smarter by handling NuGet APIs, but as of right now, PWSH-AGUI is held back by PowerLoader's hard-coded dependency on win-x64
Hello,
thank you for your project. I play around with Animations and get some drawing problems (black screen and render issues) on windows 7. Here is the playgrond project: AvaloniaAnimationTest.zip. I used this repo LoadingIndicators.WPF for the animation tests. I also recognize that some effects in Avalonia are different to Wpf (ScaleTransform, TranslateTransform,...).
Screenshot: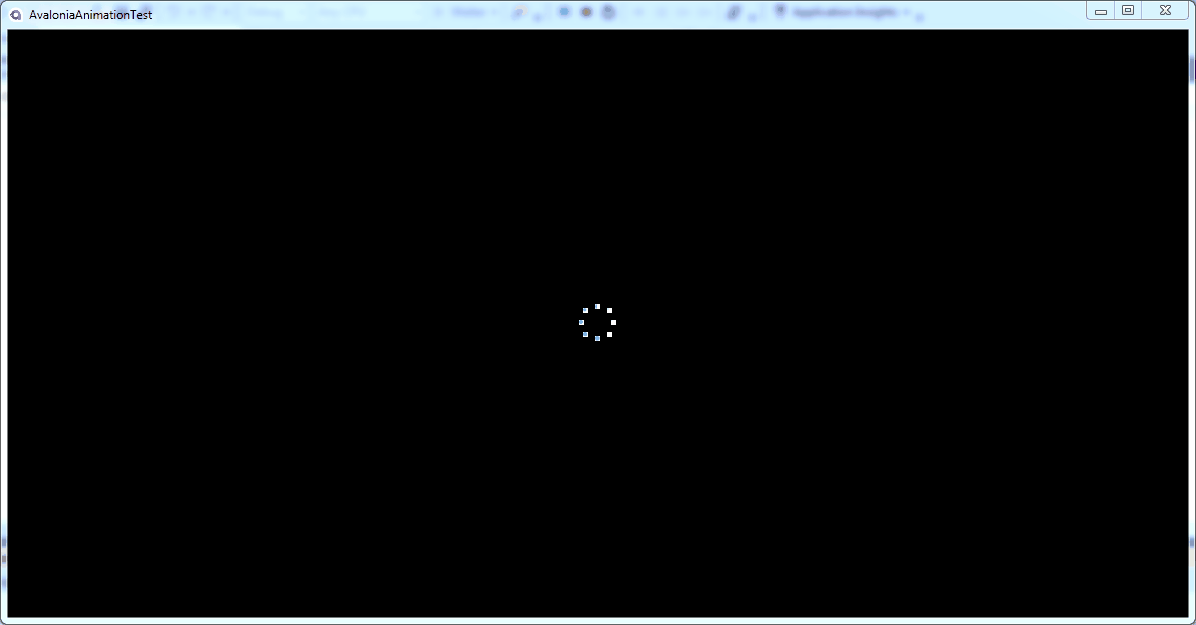
Regards