Closed mariaisid closed 1 year ago
Oo thank you @mariaisid for your issue. Does the demo works on mozilla? https://black-rock-0dc6b0d03.1.azurestaticapps.net/profile
I cannot reproduce it on it.
@mariaisid do you have any other serviceworkers registered? I had a similar issue with the CRA5 serviceworker and oidc serviceworker causing similar behaviour (only in Firefox).
@jafin that was the problem, thank you!
Issue and Steps to Reproduce
Firefox reloads page before redirecting to the authorization endpoint, it only happens in Firefox not in chromium based navigators.
Versions
6.15.2
Screenshots
firefox:
last network petitions before reloading:

chrome: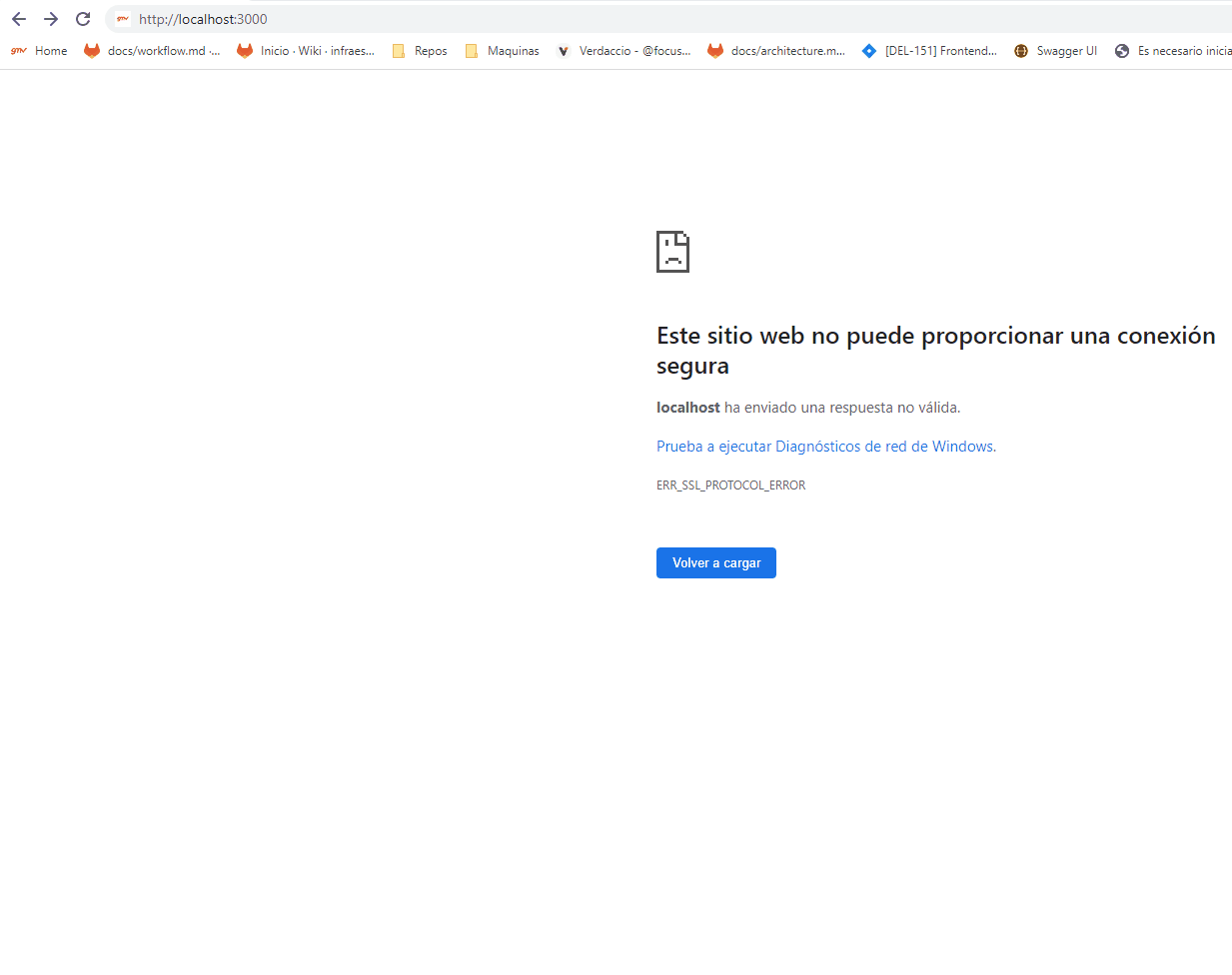
Expected
redirect to the authorization endpoint
Actual
reloads the page from the start
Additional Details