Closed Finnegan1441 closed 6 years ago
I figured it out. Changed code to:
carouselLayoutManager.addOnItemSelectionListener(new CarouselLayoutManager.OnCenterItemSelectionListener() {
@Override
public void onCenterItemChanged(int adapterPosition) {
pageIndicatorView.setSelection(adapterPosition);
creditsCarouselAdapter.currentPosition = adapterPosition;
itsCarouselAdapter.notifyDataSetChanged();
}
});
and the onBindView Holder method in CarouselAdapater to:
public int currentPosition = 0;
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
holder.imageView.setImageResource(imageslist.get(position));
viewArrayList[position] = holder;
if (position == currentPosition){
holder.itemView.setAlpha(1.0f);
}else {
holder.itemView.setAlpha(0.5f);
}
}
Closing Issue.
Im trying to make it so the center items alpha is 1.0 and the items next to it are set to 0.5f like below:
However the very first item in the carousel remains unchanged like this: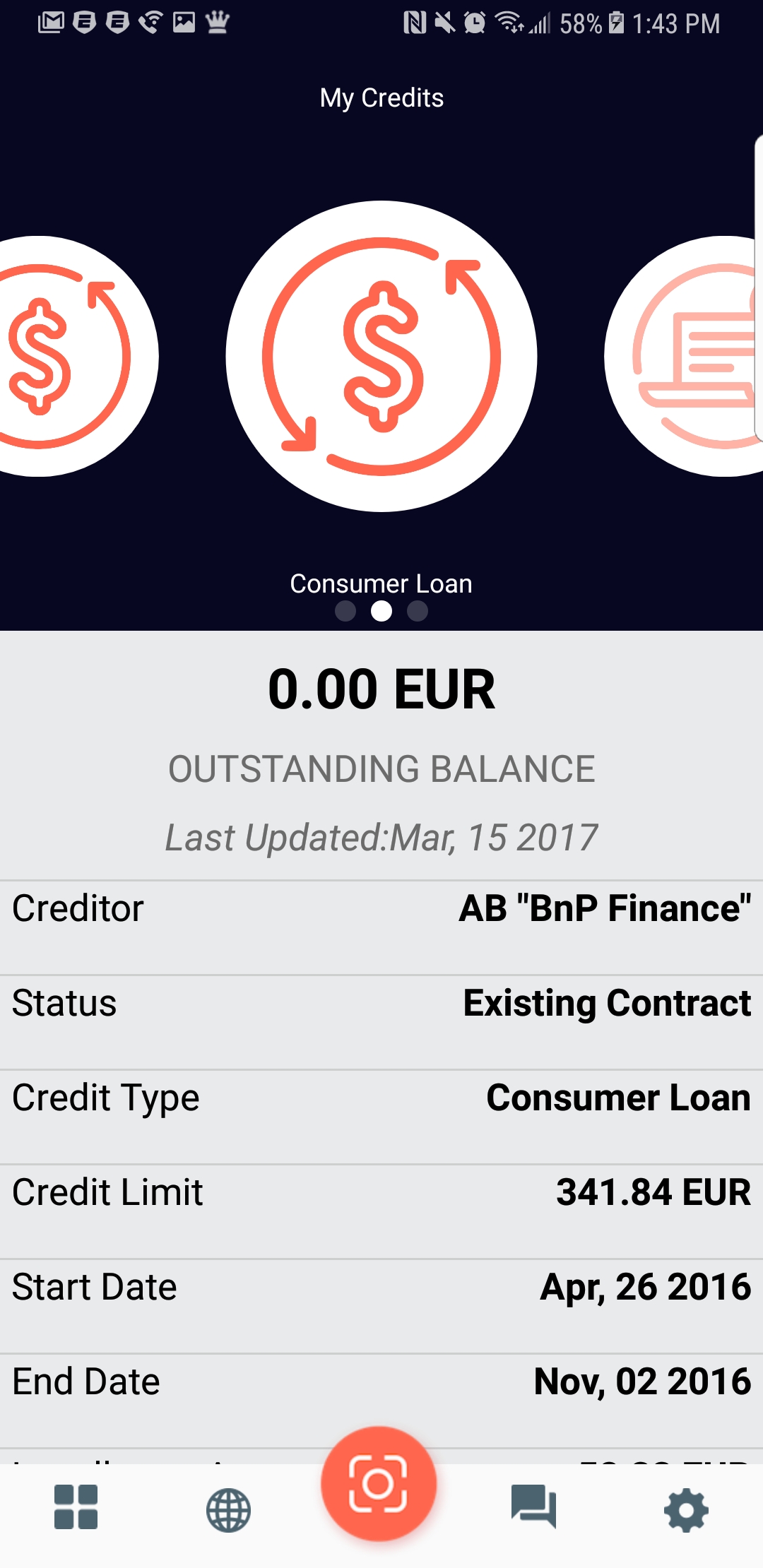
Here is the code I am using:
and here is the code for Carousel Adapter where I create a list of viewholders:
Note I have also tried this with the carouselView.getChildAt() method.