Open scholtes opened 4 years ago
Using the CosmosDB client from the CosmosDB v3 SDK instead of the older DocumentClient would also solve this issue. This is requested in #610.
@brettsam , Any known workarounds here?
We cannot support this right now due to impact on breaking changes.
@brettsam , Tagging Brett for more context
Bindings use the V2 Cosmos DB SDK which only works with Newtonsoft.Json, supporting System.Text.Json is not possible unless a new version of the binding is created using the V3 Cosmos DB SDK, which is a breaking change.
It's a good feature for a new version, but cannot be implemented in the current one.
Only workaround would be to issue the query with a V3 SDK instance with custom serialization using System.Text.Json (which is possible). Remember to either have a static/singleton client or use Functions DI (https://github.com/Azure/azure-cosmos-dotnet-v3/blob/master/Microsoft.Azure.Cosmos.Samples/Usage/AzureFunctions/Startup.cs).
@ealsur I noticed this PR #717 that seems to upgrade to the V3 SDK, should be expect that if we are using the preview nuget package for the cosmos extension? I tried it recently and it didn't seem to respect system.text.json annotation, which I assume means we are still using Newtonsoft under the hood.
V3 SDK uses Newtonsoft.Json as default serialization engine, so it's the same behavior for the 4.0.0-preview package. But you can plug in/replace with any serializer you want. We don't have official docs yet but you can leverage the new serialization factory. In your Startup.cs file:
[assembly: FunctionsStartup(typeof(FunctionApp1.Startup))]
namespace FunctionApp1
{
public class Startup : FunctionsStartup
{
public override void Configure(IFunctionsHostBuilder builder)
{
builder.Services.AddSingleton<ICosmosDBSerializerFactory, MyCosmosDBSerializerFactory>();
}
}
public class MyCosmosDBSerializerFactory : ICosmosDBSerializerFactory
{
public CosmosSerializer CreateSerializer()
{
var options = new JsonSerializerOptions();
return new CosmosSystemTextJsonSerializer(options);
}
}
}
You can have any custom implementation of CosmosSerializer, for example, one for System.Text.Json: https://github.com/Azure/azure-cosmos-dotnet-v3/tree/master/Microsoft.Azure.Cosmos.Samples/Usage/SystemTextJson
Adoption of System.Text.Json is encouraged for applications targeting .NET Core 3+. The CosmosDBAsyncCollector output binding uses Newtonsoft as its JSON Serializer.
For Azure Functions on runtime version ~3 targeting .NET Core 3.1 it would be preferred if all of the bindings directly supported System.Text.Json (preferably by default).
Repro steps
Create an empty Cosmos DB database
example-db
collectionexample-collection
.Create an Azure Function App project targeting runtime ~3 and .NET Core 3.1.
Set
example-db-connection-string
in thelocal.settings.json
.Target Azure Functions runtime ~3 and .NET Core 3.1, then add a
FunctionExample.cs
to your project with the following code:Run the function host and allow
FunctionExample
to execute once.Inspect the document created by the function in
example-collection
.Expected behavior
Document is serialized by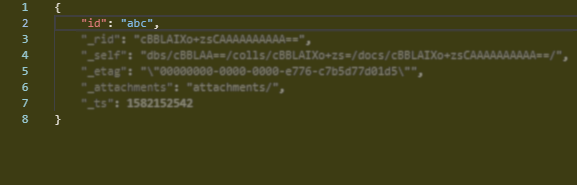
System.Text.Json
:Actual behavior
Document is serialized by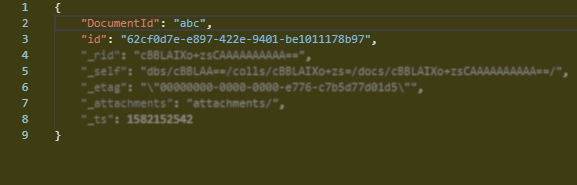
Newtonsoft
:Known workarounds
Related information
Relevant code:
Possibly other Azure Function bindings and triggers follow the same pattern (not investigated).