Closed palanglois closed 4 years ago
The find_optimal_alpha()
is based on the regularized alpha shape. In the regularized alpha shapes, k-simplicies (vertices, edges, faces) which belong to the alpha shape but do not have an incident (k+1)-simplex (respectively edges, faces, cells) in the alpha shape are ignored. Therefore the optimal alpha happens later than what you expected here.
What you are interested in is more like a general alpha shapes, such that faces in the alpha complex are not removed even if there is no incident cells. You will then get something like:
This is generated using the 3D alpha shapes CGAL demo, which has a useful slider for alpha value. I modified it to draw faces that are singular in addition to the regular ones (see https://doc.cgal.org/latest/Alpha_shapes_3/classCGAL_1_1Alpha__shape__3.html#ae887e9ecdaca28790f6ccdd73b84e40c) and hand picked a sensible alpha value.
I am not exactly sure how you could deduce which alpha value best fits your need for reconstruction purposes. The CGAL package Scale space reconstruction seems to be doing what you would like to do. Maybe @sgiraudot can expand on that as I am not very familiar with surface reconstruction.
The alpha shape mesher of Scale Space relies on a scale parameter, similarly to the Alpha Shape themselves in CGAL, so it won't help.
My advice would be to use something like CGAL::compute_average_spacing()
on the point cloud to have an estimation on the spacing between points, and then use a small factor (x2 or x3) or this value for alpha.
Hi,
Issue Details
I try to make an alpha shape approximation of a mesh. In order to do so, I used the following steps:
Here are the representations I get at each step.
Original mesh: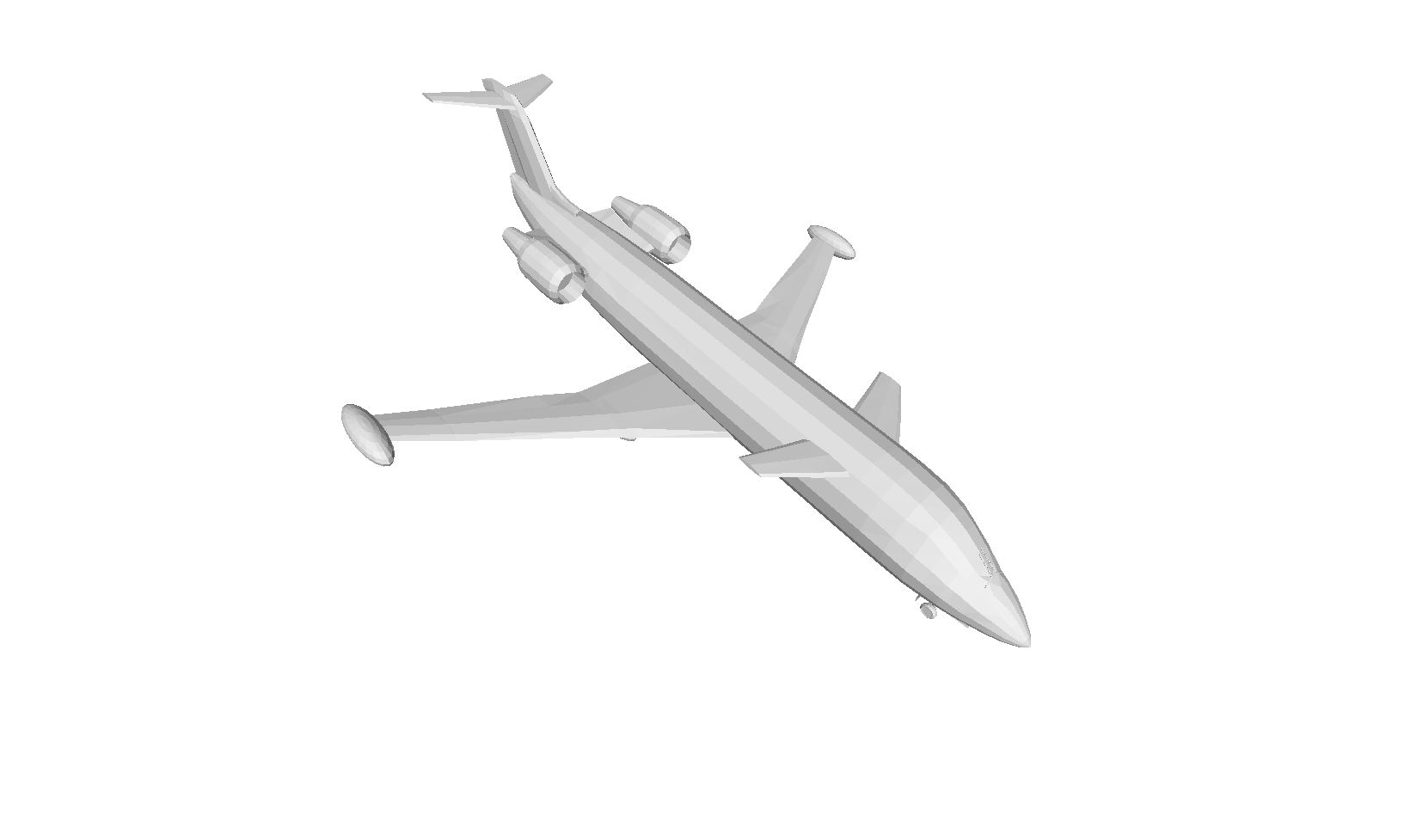
Sampled point cloud: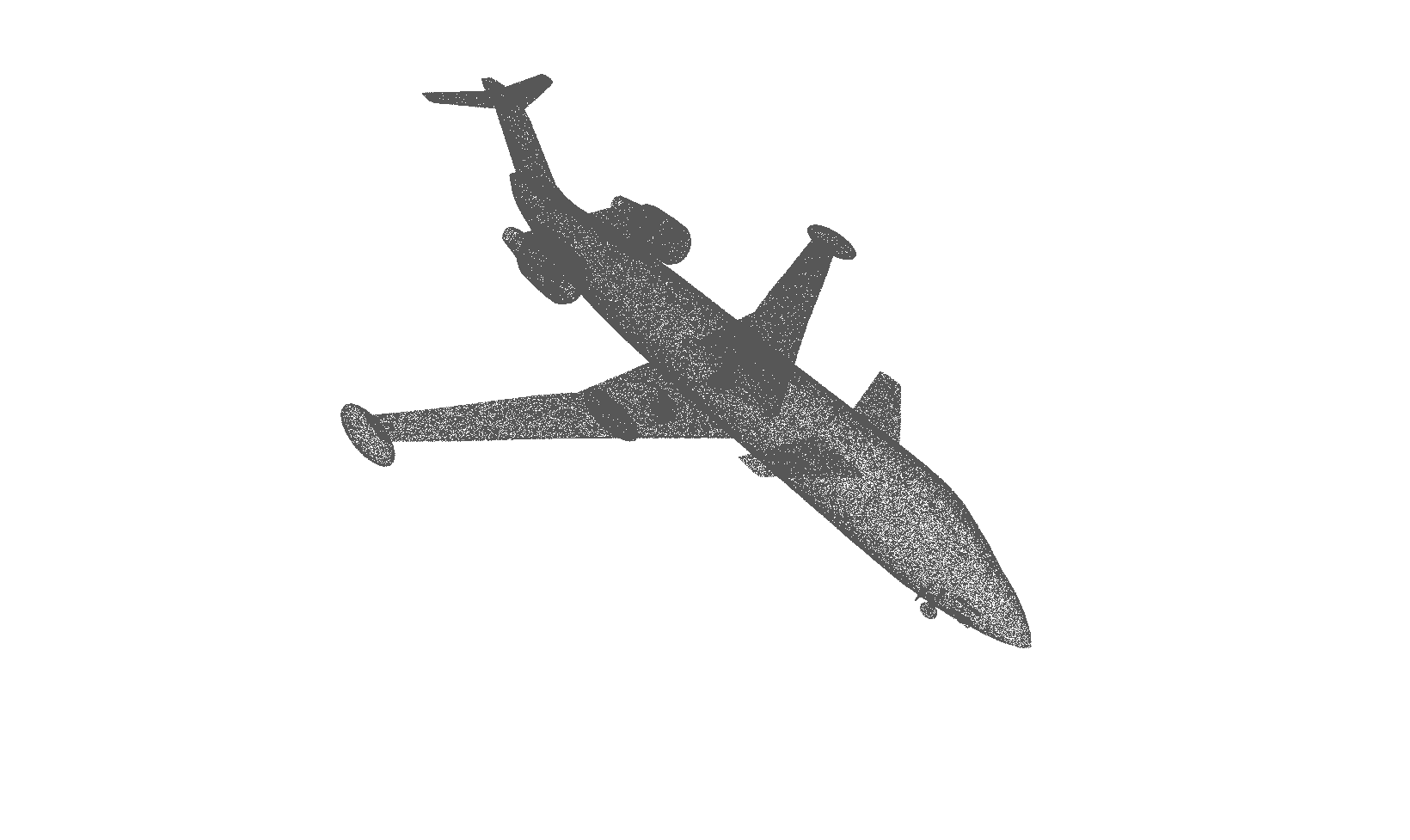
Alpha shape: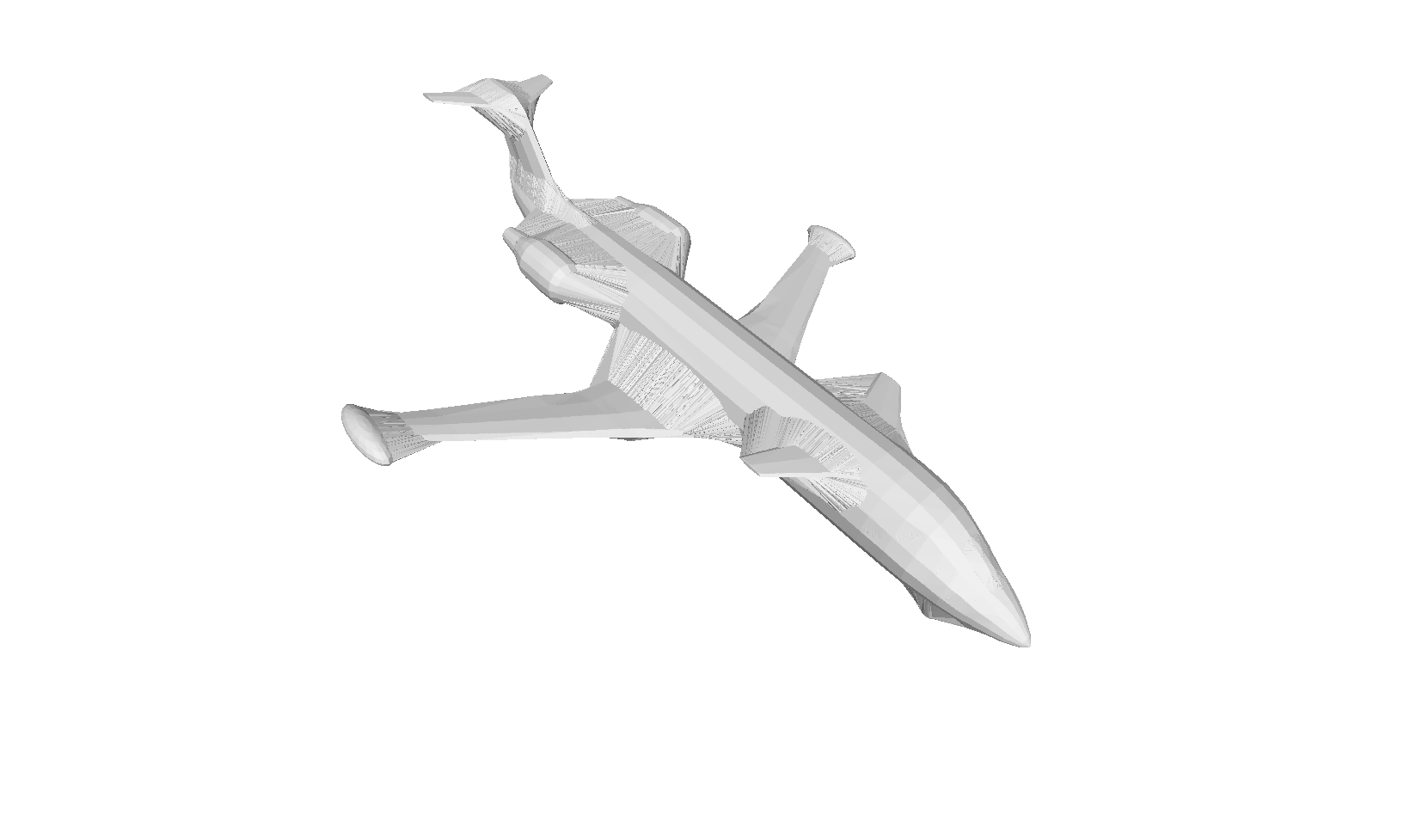
As you can see, despite the dense sampling, I get a very raw approximation in the concave parts of my mesh. Is this result normal ? I expected the alpha shape to almost perfectly fit the original mesh.
Source Code
The mesh I'm using: model.obj
CMakeLists.txt:
main.cpp
Environment