Open dmitrykudinov opened 3 years ago
I think this is the "expected" behavior. In the original publication you see: Since the proxies can be seen as approximate faces of the final mesh, we must put vertices at the intersection of the proxies. Thus, we create an anchor vertex at every original vertex where three or more regions meet. In order to account for every region, we then check whether each region boundary has at least three anchor vertices attached to it; if not, we simply add anchor vertices accordingly as it will guarantee the presence of at least one polygon per region. The spatial position of these anchor vertices is determined as follows: foreach neighboring proxy of an anchor, we compute the projection of the associated vertex from which the anchor was created onto the proxy(i.e.,its ideal position for this proxy); we then simply average these projections.
You have such an issue because you have several sheets at the corner with many self-intersections that the package try to approximate:
Thank you for reviewing the case. Do you think CGAL::Polygon_mesh_processing::smooth_mesh()
, as a preprocessing step to VSA, may help here? Sorry, cannot check it myself easily - for some reason, having a hard time making Ceres/Eigen being properly picked up on my ubuntu 16.04.
A bit more context here: we are evaluating CGAL's VSA implementation as an alternative to our in-house solution, potentially acquiring a commercial CGAL license.
In general, the self-overlapping in our input meshes cannot be completely avoided as they are generated by a neural network. We need a robust and efficient solution allowing to reduce the number of vertices through fitting approximating planar proxies, yet without the shoot-offs like in the above case.
Actually, figured out the Ceres issue and tried smooth_mesh()
- no luck here either. I guess one way to get rid of self-intersection could be through resampling and mesh reconstruction from point cloud as a preprocessing before VSA. Or is there a better CGAL-based way to get rid of self-intersections?
Please use the following template to help us solving your issue.
Issue Details
When applying
VSA::approximate_triangle_mesh()
on a triangular mesh, I'm getting some of the resulting vertices shooting off the original geometry. I tried usingPMP::orient_polygon_soup()
as a preprocessing step, but it made no difference.Before VSA: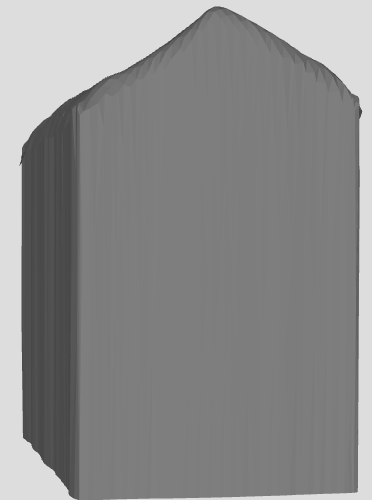
After (look at the far-left wall/corner) :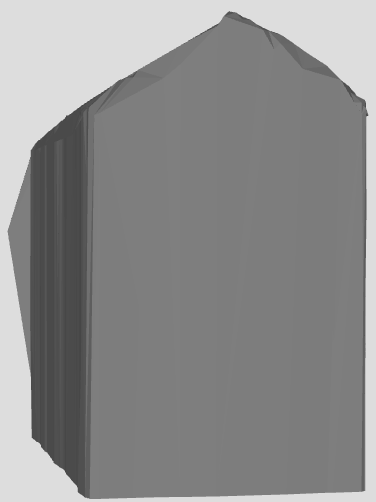
Source OBJ can be found here
Source Code
Environment