i got an issue while developing a code, which creates a 3D model of weaves (see figure)
Within my code i create multiple splines (each representing one fiber). After that, i create two loops of which each should create all fibers for one direction. (Two loops because there are two directions). Unfortunately sometimes the sweep doesn't work and gives the following error:
__File "D:\textileassembly_error.py", line 118, in
textile_assembly()
File "D:\textileassembly_error.py", line 73, in init
self.warp_cq_splines,self.weft_cq_splines= self.create_cadquerry_splines()
File "D:\textileassembly_error.py", line 94, in create_cadquerry_splines
weft_cq_splines.append(self.make_fiber(self.points_weft[i],self.cross_section,[0,1,0]))
File "D:\textileassembly_error.py", line 85, in makefiber
sweep=r.sweep(path)
File "C:\Users\Stefan\anaconda3\lib\site-packages\cadquery\cq.py", line 2848, in sweep
r = self._sweep(
File "C:\Users\Stefan\anaconda3\lib\site-packages\cadquery\cq.py", line 3244, in _sweep
thisObj = Solid.sweep(
File "C:\Users\Stefan\anaconda3\lib\site-packages\cadquery\occimpl\shapes.py", line 2585, in sweep
builder.MakeSolid()
Standard_NoSuchObject: NCollectionIndexedDataMap::FindFromKey
I created a file of my code which creates this error:
`# -- coding: utf-8 --
"""
"""
import cadquery as cq
import datetime
import numpy as np
class cross_section:
def __init__(self, width, height, form,):
self.width = width
self.height = height
self.form = form
#form property in sub classes?
@property
def form(self):
return self._form
@form.setter
def form(self,value):
if value == 'elliptical' or value == 'racetrack' or value == 'lenticular':
self._form = value
else:
raise ValueError ("This chosen cross section form is not available.")
@property
def width(self):
return self._width
@width.setter
def width(self,value):
if value <= 0:
raise ValueError ("The width must be greater than 0.")
self._width=value
@property
def height(self):
return self._height
@height.setter
def height(self,value):
if value <= 0:
raise ValueError ("The height must be greater than 0.")
self.height=value
class ellipse(cross_section):
# a = Weave.roving.width/2
# b = Weave.roving.height/2
def __init__(self,width,height):
super().__init__(width,height,form = 'elliptical')
self.a = width/2
self.b = height/2
self.points = self.create_cs_points(360)
def create_cs_points(self,amount_of_coordinate_points):
num_points =360
angles = np.linspace(0,360,num_points)
x_cs = self.a*np.cos(np.radians(angles))
y_cs = self.b*np.sin(np.radians(angles))
points =[]
for i in range (0,num_points,1):
points.append((x_cs[i],y_cs[i]))
return (points)
class textile_assembly:
def init(self):
self.weave=weave
self.distance_between_fibers = 6
self.cross_section = ellipse(width=5,height=1)
self.points_weft=[[[0, 0, -0.5], [0, 3.0, -0.5], [0, 6.0, -0.5], [0, 9.0, -0.5], [0, 12.0, -0.5]], [[6.0, 0, 0.5], [6.0, 3.0, 0.5], [6.0, 6.0, 0.5], [6.0, 9.0, 0.5], [6.0, 12.0, 0.5]]]
self.points_warp=[[[0, 0, 0.5], [3.0, 0, 0.5], [6.0, 0, 0], [9.0, 0, -0.5], [12.0, 0, -0.5]], [[0, 6.0, 0.5], [3.0, 6.0, 0.5], [6.0, 6.0, 0], [9.0, 6.0, -0.5], [12.0, 6.0, -0.5]]]
self.warp_cq_splines,self.weft_cq_splines= self.create_cadquerry_splines()
self.create_cadquerry_assembly()
def make_fiber(self,points,cross_section,direction):
if direction[0] != 0:
plane ="YZ"
elif direction[1] != 0:
plane="XZ"
elif direction[2] != 0:
plane="XY"
r = cq.Workplane(plane).spline(cross_section.points).close()
path= cq.Workplane().spline(points, makeWire=True)
sweep_=r.sweep(path)
return(sweep_)
def create_cadquerry_splines(self):
print(self.points_weft)
print(self.points_warp)
weft_cq_splines =[]
for i in range(2):
print("Weft i:",i)
weft_cq_splines.append(self.make_fiber(self.points_weft[i],self.cross_section,[0,1,0]))
weft_cq_splines[i] = weft_cq_splines[i].translate([i*self.distance_between_fibers+0.5*5+0.5*1,0,0])
warp_cq_splines = []
for i in range(2):
print("Warp i:",i)
warp_cq_splines.append(self.make_fiber(self.points_warp[i],self.cross_section,[1,0,0]))
warp_cq_splines[i] = warp_cq_splines[i].translate([0,i*self.distance_between_fibers+0.5*5+0.5*1,0])
return(warp_cq_splines,weft_cq_splines)
def create_cadquerry_assembly(self):
assembly =cq.Assembly()
for i in range(2):
assembly.add(self.warp_cq_splines[i], name="WarpFiber"+str(i))
for i in range(2):
assembly.add(self.weft_cq_splines[i], name="WeftFiber"+str(i))
now = datetime.datetime.now().strftime("%Y%m%d_%H%M%S")
assembly.save("assembly"+now+".step")
# self.export_assembly(assembly)
# print("Saved")
return(assembly)
textile_assembly()
`
Can someone tell me what i do wrong and/or how to solve this issue?
Hi there,
i got an issue while developing a code, which creates a 3D model of weaves (see figure)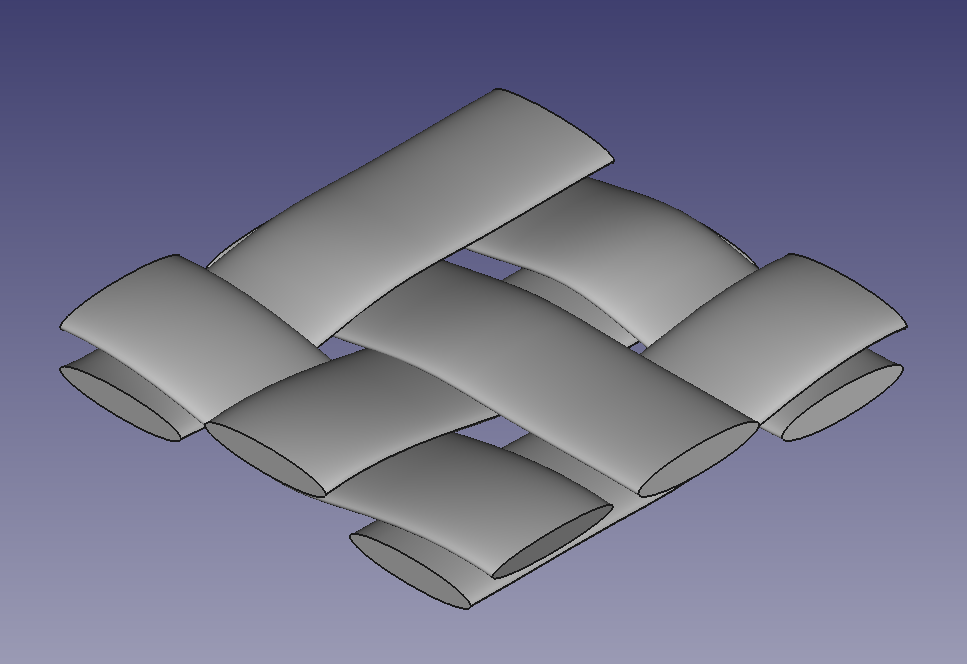
Within my code i create multiple splines (each representing one fiber). After that, i create two loops of which each should create all fibers for one direction. (Two loops because there are two directions). Unfortunately sometimes the sweep doesn't work and gives the following error:
__File "D:\textileassembly_error.py", line 118, in
textile_assembly()
File "D:\textileassembly_error.py", line 73, in init
self.warp_cq_splines,self.weft_cq_splines= self.create_cadquerry_splines()
File "D:\textileassembly_error.py", line 94, in create_cadquerry_splines
weft_cq_splines.append(self.make_fiber(self.points_weft[i],self.cross_section,[0,1,0]))
File "D:\textileassembly_error.py", line 85, in makefiber
sweep=r.sweep(path)
File "C:\Users\Stefan\anaconda3\lib\site-packages\cadquery\cq.py", line 2848, in sweep
r = self._sweep(
File "C:\Users\Stefan\anaconda3\lib\site-packages\cadquery\cq.py", line 3244, in _sweep
thisObj = Solid.sweep(
File "C:\Users\Stefan\anaconda3\lib\site-packages\cadquery\occimpl\shapes.py", line 2585, in sweep
builder.MakeSolid()
Standard_NoSuchObject: NCollectionIndexedDataMap::FindFromKey
I created a file of my code which creates this error:
`# -- coding: utf-8 -- """ """ import cadquery as cq import datetime import numpy as np
class cross_section:
class ellipse(cross_section):
class textile_assembly: def init(self):
self.weave=weave
textile_assembly() `
Can someone tell me what i do wrong and/or how to solve this issue?