Closed counselorbot[bot] closed 2 years ago
🛑 There was an error: Error: You forgot to add your 'HACKERVOICE_ENDPOINT' secret! 🛑
Go ahead and merge this branch to main
to move on. Great work finishing this section!
⚠️ If you receive a
Conflicts
error, simply pressResolve conflicts
and you should be good to merge!
Move on to the next issue.
Week 1 Step 4 ⬤⬤⬤⬤◯◯◯◯◯ | 🕐 Estimated completion: 35-45 minutes
Building our first function ⚡[HackerVoice]
✅ Tasks:
git pull
and create a new branch calledhackervoice
password
and returns it in the function's bodyHACKERVOICE_ENDPOINT
and commit the function's code in a file namedhackervoice/index.js
on thehackervoice
branchhackervoice
tomain
, and only merge when the bot approves your changes!**🚧 Test your Work
Option 1: Paste the function url directly in your browser and add the query parameters to the end of the url:
¶m_name=param_value
. Your inputted password value should appear.Option 2: Use Postman! Paste the function url and make a GET request. In the output box, you should receive the value you put in for the request parameter.
1: Create an Azure Account
Azure is Microsoft's cloud computing platform (similar to Google Cloud Platform and Amazon Web Services).
2: Set Up Azure Locally on VS Code
Function
❓ What should running the function look like?
Start the debugger by going to index.js and then pressing the F5 key. > Having trouble with Azure Tools? Try installing it [this way](https://docs.microsoft.com/en-us/azure/azure-functions/functions-run-local?tabs=macos%2Ccsharp%2Cbash#install-the-azure-functions-core-tools)Once you receive the localhost link in the terminal, follow it and notice the terminal log "Executing 'Functions.[Name of your function]'" indicating that you made a request to the function. 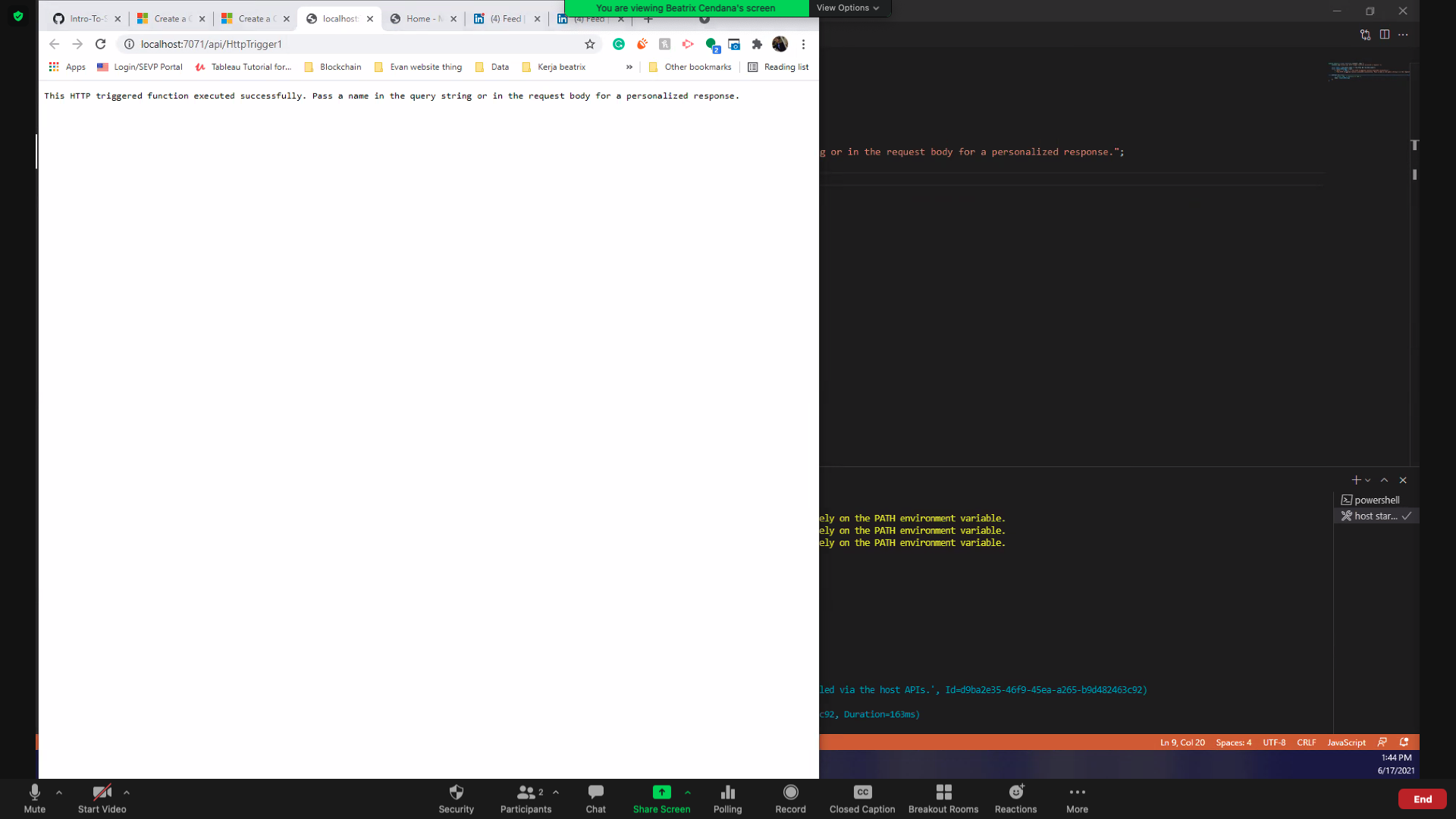
If the request is successfully made in Postman this is what it should look like: 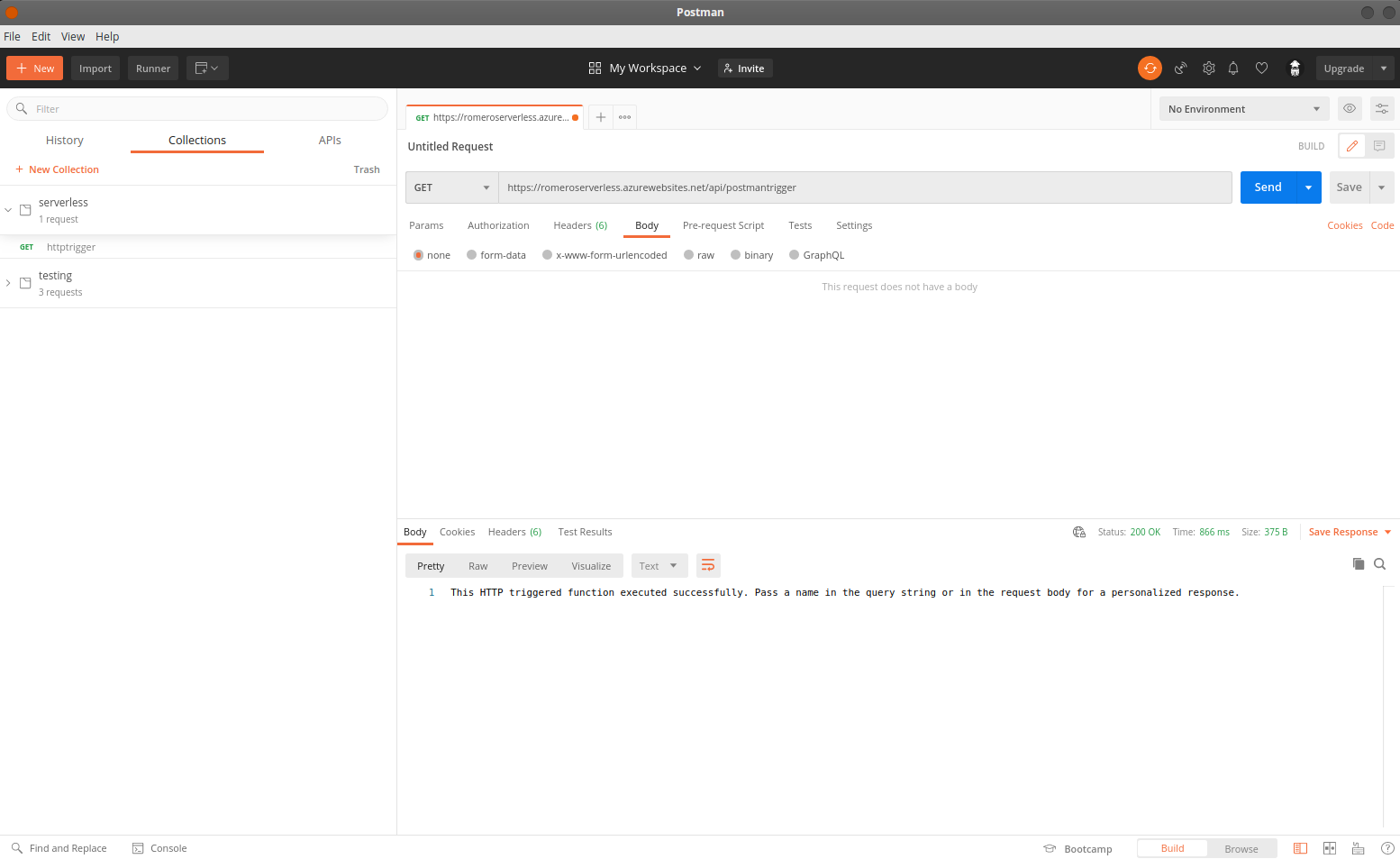 Once you deploy/publish the code to Azure successfully, you will get the function url in the Output of VS Code: 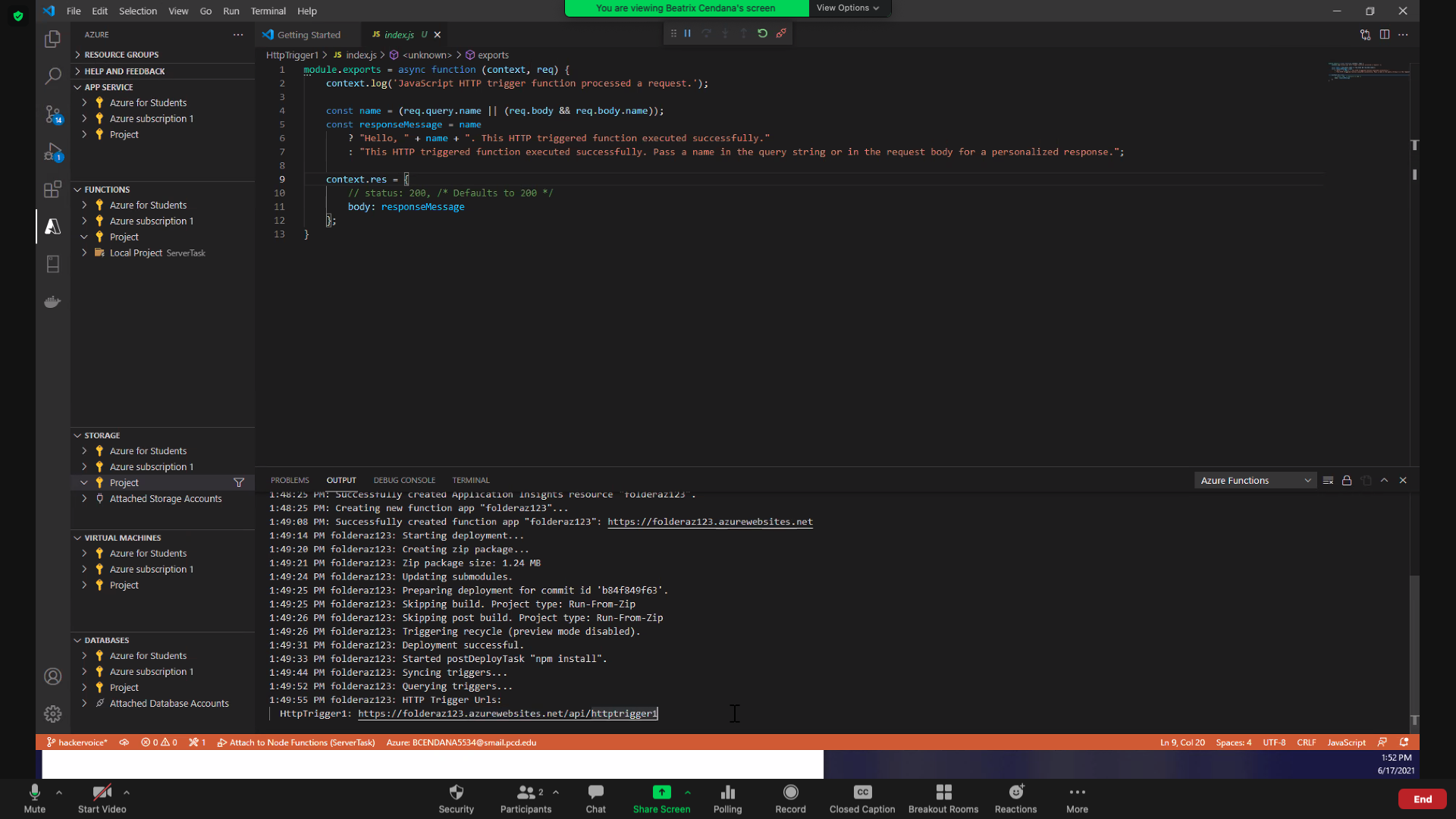
3: Create your own HTTP Trigger Function
You should see the HTTP Trigger Function template code Microsoft Azure starts you with when you first create your trigger.
❓ What is happening in index.js?
- Start of function definition: `module.exports = async function` - Print comment in Azure Console anytime the function is triggered: `context.log()` - Get parameter from request body: `const name` - Conditional (ternary) operator to print message if parameter exists (else print error message): ```javascript //condition: if name exists name //? is chosen if the condition evaluates to true ? "Hello, " + name + ". This HTTP triggered function executed successfully." //: is chosen if the condition evaluates to false : "This HTTP triggered function executed successfully. Pass a name in the query string or in the request body for a personalized response."; ``` - Results of that conditional ternary statement are assigned to `responseMessage` which is returned in the function body
Now, let's begin coding by modifying that template. First, you will need to retrieve content from a request parameter named
password
. Recall that parameters look like this in a full URL:❓ How to get content from a request parameter?
Request parameters are a way for an HTTP request to take in information! They are pretty much identical in purpose to why you would want a parameter for a function in a coding language. In this instance, we will need a parameter for the password. - Request parameters are a property of the `req` or request parameter of the module - The request has a `query` object that stores all of the parameters passed in - You don't need to specify what parameters the user needs to input into the HTTP request - You can acess any parameters that are sent in You would access a parameter by calling on the query like this: ```javascript
Finally, we have to return something to the users. In this case, we will be returning the value of the request parameter we just retrieved.
❓ How can I return something in the function body?
In Azure, `context` is an object that holds data about the response of the HTTP function. Read more about [Accessing the request and response](https://docs.microsoft.com/en-us/azure/azure-functions/functions-reference-node?tabs=v2#accessing-the-request-and-response). In our HTTP trigger function, we have defined context object with the body property: ```javascript context.res = { // status: 200, /* Defaults to 200 */ body: responseMessage }; ``` To return the password in body, simply replace `responseMessage` with `password`.
4: Test your Function with Postman
password
and give it any random valuepassword
parameter in the body5: Add Repository Secrets
❓ How do I add a respository secret?
[Here are some steps:](https://docs.github.com/en/actions/reference/encrypted-secrets#creating-encrypted-secrets-for-a-repository) 1. On GitHub, navigate to the main page of the repository. 2. Under your repository name, click `Settings`.  3. In the left sidebar, click Secrets. 4. Click New repository secret. 5. Type a name for your secret in the Name input box. 6. Enter the value for your secret. 7. Click Add secret.📹 Walkthrough Video