Closed zhanglichuan closed 5 years ago
初始化的代码贴一下
@property (nonatomic, strong) CHDMainTabBarContrllerConfig * tabBarControllerConfig;
self.window.rootViewController = self.tabBarControllerConfig.tabBarController;
- (CHDMainTabBarContrllerConfig *)tabBarControllerConfig {
if (!_tabBarControllerConfig) {
_tabBarControllerConfig = [[CHDMainTabBarContrllerConfig alloc] init];
}
return _tabBarControllerConfig;
}
#import <Foundation/Foundation.h>
#import <CYLTabBarController/CYLTabBarController.h>
@interface CHDMainTabBarContrllerConfig : NSObject
@property (nonatomic, readonly, strong) CYLTabBarController *tabBarController;
@end
#import "CHDMainTabBarContrllerConfig.h"
//#import <UINavigationController+FDFullscreenPopGesture.h>
#import "FirstMainVC.h"
#import "SecondMianVC.h"
#import "SXMMallVC.h"
#import "FourthVC.h"
//#import "CHDLoginVC.h" // 登录页面
@interface CHDMainTabBarContrllerConfig ()
<UITabBarControllerDelegate>
@property (nonatomic, readwrite, strong) CYLTabBarController *tabBarController;
@property (nonatomic, strong) FirstMainVC * firstVC;
@property (nonatomic, strong) BaseNavgationController * firstNav;
@property (nonatomic, strong) SecondMianVC * secondVC;
@property (nonatomic, strong) BaseNavgationController * secondNav;
@property (nonatomic, strong) SXMMallVC * thirdVC;
@property (nonatomic, strong) BaseNavgationController * thirdNav;
@property (nonatomic, strong) FourthVC * fourthVC;
@property (nonatomic, strong) BaseNavgationController * fourthNav;
@end
@implementation CHDMainTabBarContrllerConfig
#pragma mark - Action
/** 返回的控制器数组 */
- (NSArray *)viewControllers {
/*************** 首页 ******************/
self.firstVC = [[FirstMainVC alloc] init];
self.firstNav = [[BaseNavgationController alloc] initWithRootViewController:self.firstVC];
/*************** BCT ******************/
self.secondVC = [[SecondMianVC alloc] init];
self.secondNav = [[BaseNavgationController alloc] initWithRootViewController:self.secondVC];
/*************** 商城 ******************/
self.thirdVC = [[SXMMallVC alloc] init];
self.thirdNav = [[BaseNavgationController alloc] initWithRootViewController:self.thirdVC];
/*************** 我的 ******************/
self.fourthVC = [[FourthVC alloc] init];
self.fourthNav = [[BaseNavgationController alloc] initWithRootViewController:self.fourthVC];
NSArray *viewControllers = @[self.firstNav, self.secondNav, self.thirdNav, self.fourthNav];
return viewControllers;
}
#pragma mark - UITabBarControllerDelegate
/** TabBar的图片包括 选中 和 未选中 */
- (NSArray *)tabBarItemsAttributesForController {
NSDictionary *dict1 = @{CYLTabBarItemTitle : @"首页",
CYLTabBarItemImage : @"首页未选中",
CYLTabBarItemSelectedImage : @"首页选中"};
NSDictionary *dict2 = @{CYLTabBarItemTitle : @"商品管理",
CYLTabBarItemImage : @"商品管理未选中",
CYLTabBarItemSelectedImage : @"商品管理选中"};
NSDictionary *dict4 = @{CYLTabBarItemTitle : @"商城",
CYLTabBarItemImage : @"商城",
CYLTabBarItemSelectedImage : @"商城选中"};
NSDictionary *dict5 = @{CYLTabBarItemTitle : @"我的",
CYLTabBarItemImage : @"我的未选中",
CYLTabBarItemSelectedImage : @"我的-我的选中"};
NSArray *tabBarItemsAttributes = @[dict1, dict2, dict4, dict5];
return tabBarItemsAttributes;
}
/**
* 更多TabBar自定义设置:比如:tabBarItem 的选中和不选中文字和背景图片属性、tabbar 背景图片属性等等
*/
- (void)customizeTabBarAppearance:(CYLTabBarController *)tabBarController {
// 普通状态下的文字属性
NSMutableDictionary *normalAttrs = [NSMutableDictionary dictionary];
normalAttrs[NSForegroundColorAttributeName] = [UIColor grayColor];
// 选中状态下的文字属性
NSMutableDictionary *selectedAttrs = [NSMutableDictionary dictionary];
selectedAttrs[NSForegroundColorAttributeName] = HexColorInt32_t(AAD23E);
// 设置文字属性
UITabBarItem *tabBar = [UITabBarItem appearance];
[tabBar setTitleTextAttributes:normalAttrs forState:UIControlStateNormal];
[tabBar setTitleTextAttributes:selectedAttrs forState:UIControlStateSelected];
// 背景颜色
[[UITabBar appearance] setBarTintColor:HexColorInt32_t(ffffff)];
// 设置不透明
[[UITabBar appearance] setTranslucent:NO];
}
- (BOOL)tabBarController:(UITabBarController *)tabBarController shouldSelectViewController:(UIViewController *)viewController {
// BaseNavgationController * nav = (BaseNavgationController *)viewController;
// if ([nav.topViewController isKindOfClass:NSClassFromString(@"CHDTwoVC")] ||
// [nav.topViewController isKindOfClass:NSClassFromString(@"CHDThreeVC")] ||
// [nav.topViewController isKindOfClass:NSClassFromString(@"CHDFourVC")]) {
// if (!BTool.isUserLogin) {
// CHDLoginVC * login = [CHDLoginVC new];
// [tabBarController presentViewController:[[BaseNavgationController alloc] initWithRootViewController:login] animated:YES completion:nil];
// return NO;
// }
// }
return YES;
}
//- (void)tabBarController:(UITabBarController *)tabBarController didSelectControl:(UIControl *)control {
// UIView *animationView;
// if ([control cyl_isTabButton]) {
// animationView = [control cyl_tabImageView];
// }
//
// // 即使 PlusButton 也添加了点击事件,点击 PlusButton 后也会触发该代理方法。
// if ([control cyl_isPlusButton]) {
// UIButton *button = CYLExternPlusButton;
// animationView = button.imageView;
// }
// [self addScaleAnimationOnView:animationView repeatCount:1];
//}
//缩放动画
- (void)addScaleAnimationOnView:(UIView *)animationView repeatCount:(float)repeatCount {
//需要实现的帧动画,这里根据需求自定义
CAKeyframeAnimation *animation = [CAKeyframeAnimation animation];
animation.keyPath = @"transform.scale";
animation.values = @[@1.0,@1.2,@0.9,@1.1,@0.95,@1.02,@1.0];
animation.duration = 1;
animation.repeatCount = repeatCount;
animation.calculationMode = kCAAnimationCubic;
[animationView.layer addAnimation:animation forKey:nil];
}
#pragma mark - 懒加载
- (CYLTabBarController *)tabBarController {
if (!_tabBarController) {
UIEdgeInsets imageInsets = UIEdgeInsetsMake(-3, 0, 0, 0);
UIOffset titlePositionAdjustment = UIOffsetMake(0, -3);
_tabBarController = [CYLTabBarController tabBarControllerWithViewControllers:self.viewControllers tabBarItemsAttributes:self.tabBarItemsAttributesForController imageInsets:imageInsets titlePositionAdjustment:titlePositionAdjustment];
_tabBarController.delegate = self;
[self customizeTabBarAppearance:_tabBarController];
}
return _tabBarController;
}
@end
pod 'CYLTabBarController' # 1.25.0
我在测试了,在iOS10.0中,好像都有这样的情况
imageset设置有问题,四个值加起来要等于0,其它issue讨论过
好的,已经可以的,
top + bottom == 0
left+right == 0
才可以,谢谢
模拟器和真机都会这样,iPhone5、iOS10,iPhone6以上都不会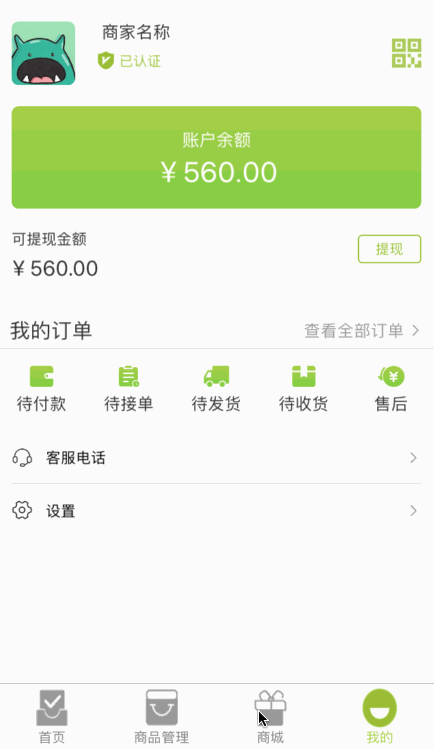