Open Christian-health opened 6 years ago
emit only the first item (or the first item that meets some condition) emitted by an Observable 仅发出由Observable发出的第一个项目(或第一个满足某些条件的项目)
If you are only interested in the first item emitted by an Observable, or the first item that meets some criteria, you can filter the Observable with the First operator. 如果您只对Observable发出的第一个项目感兴趣,或者符合某些条件的第一个项目,则可以使用First操作符过滤Observable。
In some implementations, First is not implemented as a filtering operator that returns an Observable, but as a blocking function that returns a particular item at such time as the source Observable emits that item. In those implementations, if you instead want a filtering operator, you may have better luck with Take(1) or ElementAt(0). 在某些实现中,First不是作为返回Observable的过滤运算符实现的,而是作为在源Observable发出该项的时候返回特定项的阻塞函数。 在这些实现中,如果您想要一个过滤操作符,那么您可能会更乐意携带Take(1)或ElementAt(0)。 In some implementations there is also a Single operator. It behaves similarly to First except that it waits until the source Observable terminates in order to guarantee that it only emits a single item (otherwise, rather than emitting that item, it terminates with an error). You can use this to not only take the first item from the source Observable but to also guarantee that there was only one item.
operate upon the emissions and notifications from an Observable 操作可观测的排放和通知
The Subscribe operator is the glue that connects an observer to an Observable. In order for an observer to see the items being emitted by an Observable, or to receive error or completed notifications from the Observable, it must first subscribe to that Observable with this operator. 订阅操作符是将观察者连接到Observable的粘合剂。 为了让观察者看到Observable发出的项目,或者从Observable接收错误或完成的通知,它必须首先使用该操作符订阅该Observable。 A typical implementation of the Subscribe operator may accept one to three methods (which then constitute the observer), or it may accept an object (sometimes called an Observer or Subscriber) that implements the interface which includes those three methods: 订阅运算符的典型实现可以接受一到三种方法(其然后构成观察者),或者它可以接受实现包括这三种方法的接口的对象(有时称为观察者或订阅者): onNext An Observable calls this method whenever the Observable emits an item. This method takes as a parameter the item emitted by the Observable. onNext 每当Observable发出一个项目时,Observable调用这个方法。该方法将Observable发出的项目作为参数。 onError An Observable calls this method to indicate that it has failed to generate the expected data or has encountered some other error. This stops the Observable and it will not make further calls to onNext or onCompleted. The onError method takes as its parameter an indication of what caused the error (sometimes an object like an Exception or Throwable, other times a simple string, depending on the implementation). onError 一个Observable调用此方法来表示它无法生成预期的数据或遇到其他错误。这将停止Observable,它不会进一步调用onNext或onCompleted。 onError方法作为其参数,指示导致错误的原因(有时像Exception或Throwable这样的对象,其他时候是简单的字符串,具体取决于实现)。 onCompleted An Observable calls this method after it has called onNext for the final time, if it has not encountered any errors. onCompleted 如果没有遇到任何错误,则可以在最后一次调用onNext之后调用此方法。 An Observable is called a “cold” Observable if it does not begin to emit items until an observer has subscribed to it; an Observable is called a “hot” Observable if it may begin emitting items at any time, and a subscriber may begin observing the sequence of emitted items at some point after its commencement, missing out on any items emitted previously to the time of the subscription. 一个Observable被称为“冷”可观察,如果它不开始发射项目,直到观察者订阅它;一个可观测值被称为“热”可观察,如果它可以随时开始发射项目,并且用户可以在其开始之后的某个时刻开始观察发射物体的序列,并忽略在预定时间之前发射的任何物品。
In RxJS, you can subscribe to an Observable in two ways: 在RxJS中,您可以通过两种方式订阅Observable: subscribe a single function to either the onNext, the onCompleted, or onError notifications from an Observable, with subscribeOnNext, subscribeOnCompleted, or subscribeOnError respectively subscribe by passing zero to three individual functions, or an object that implements those three functions, into either the subscribe or forEach operator (those operators behave identically). 可以使用 subscribeOnNext, subscribeOnCompleted, or subscribeOnError分别订阅一个Observable的 onNext, the onCompleted, onError 。 或者通过将零到三个单独的函数或实现这三个函数的对象订阅到subscribe或forEach操作符(这些操作符的行为相同)。
var source = Rx.Observable.range(0, 3)
var subscription = source.subscribeOnNext(
function (x) {
console.log('Next: %s', x);
});
Next: 0
Next: 1
Next: 2
var source = Rx.Observable.range(0, 3);
var subscription = source.subscribeOnCompleted(
function () {
console.log('Completed');
});
Completed
var source = Rx.Observable.throw(new Error());
var subscription = source.subscribeOnError(
function (err) {
console.log('Error: %s', err);
});
Error: Error
var observer = Rx.Observer.create(
function (x) { console.log('Next: %s', x); },
function (err) { console.log('Error: %s', err); },
function () { console.log('Completed'); });
var source = Rx.Observable.range(0, 3)
var subscription = source.subscribe(observer);
Next: 0
Next: 1
Next: 2
Completed
var source = Rx.Observable.range(0, 3)
var subscription = source.subscribe(
function (x) { console.log('Next: %s', x); },
function (err) { console.log('Error: %s', err); },
function () { console.log('Completed'); });
Next: 0
Next: 1
Next: 2
Completed
emit only the last item (or the last item that meets some condition) emitted by an Observable
只发出一个Observable发出的最后一个项目(或满足某些条件的最后一个项目)
If you are only interested in the last item emitted by an Observable, or the last item that meets some criteria, you can filter the Observable with the Last operator. 如果您只对Observable发出的最后一个项目或符合某些条件的最后一个项目感兴趣,则可以使用Last操作符过滤Observable。 In some implementations, Last is not implemented as a filtering operator that returns an Observable, but as a blocking function that returns a particular item when the source Observable terminates. In those implementations, if you instead want a filtering operator, you may have better luck with TakeLast(1). 在某些实现中,Last不是作为返回Observable的过滤运算符实现的,而是作为阻止函数,当源Observable终止时返回特定项。 在这些实现中,如果你想要一个过滤操作符,你可能会更好的运气与TakeLast(1)。 (如果Last作为运算符实现的那么就会返回一个Observable,但是如果作为阻止函数,那么就不会返回一个Observable所以这个时候,最好使用TakeLast(1)运算符,因为TakeLast会返回一个Observable)
RxJS implements the last operator. It optionally takes a predicate function as a parameter, in which case, rather than emitting the last item from the source Observable, the resulting Observable will emit the last item from the source Observable that satisfies the predicate. RxJS实现Last一个操作符。 它可以选择使用谓词函数作为参数,在这种情况下,而不是从源Observable发出最后一个项目,所得到的Observable将从源Observable中发出满足谓词的最后一个项目。 The predicate function itself takes three arguments: 谓词函数本身有三个参数:
//取出最后一个对2取余数为1的元素,所以是9
var source = Rx.Observable.range(0, 10)
.last(function (x, idx, obs) { return x % 2 === 1; });
var subscription = source.subscribe(
function (x) { console.log('Next: ' + x); },
function (err) { console.log('Error: ' + err); },
function () { console.log('Completed'); });
Next: 8
Completed
If the source Observable emits no items (or no items that match the predicate), last will terminate with a “Sequence contains no elements.” onError notification. 如果源Observable不发出任何项目(或没有匹配谓词的项目),最后将以“序列不包含元素”终止。onError通知。 If instead you want the Observable to emit a default value in such a case, you can pass a second parameter (named defaultValue) to last: 如果相反,您希望Observable在这种情况下发出默认值,则可以传递第二个参数(命名为defaultValue)来执行以下操作:
var source = Rx.Observable.range(0, 10)
.last(function (x, idx, obs) { return x > 42; }, 88 );
//如果没有满足谓词条件的数值,那么返回后面的默认值88
var subscription = source.subscribe(
function (x) { console.log('Next: ' + x); },
function (err) { console.log('Error: ' + err); },
function () { console.log('Completed'); });
Next: 88
Completed
本页
periodically gather items emitted by an Observable into bundles and emit these bundles rather than emitting the items one at a time 定期将Observable发射的项目收集到捆绑包中,并发布这些捆绑包,而不是一次发送一个项目
The Buffer operator transforms an Observable that emits items into an Observable that emits buffered collections of those items. There are a number of variants in the various language-specific implementations of Buffer that differ in how they choose which items go in which buffers.
Buffer运算符将原来的Observable发出的item组装成为一个个的Buffer,并产生一个新的Observable,然后通过这个新的Observable发出变成Buffer的item集合, 缓冲区的各种语言特定实现中有许多变体,
还有就是可以设置buffer生成的规则,也就是设置一个buffer中有什么样子的元素。
Note that if the source Observable issues an onError notification, Buffer will pass on this notification immediately without first emitting the buffer it is in the process of assembling, even if that buffer contains items that were emitted by the source Observable before it issued the error notification.
请注意,如果源Observable发出onError通知,Buffer将立即传递此通知,而不会首先发出正在组装的缓冲区,即使该缓冲区包含源Observable在发出错误通知之前发出的项目
The Window operator is similar to Buffer but collects items into separate Observables rather than into data structures before reemitting them.
Window操作符和buffer操作符号类似,但是收集items到一个单独的Observable而不是到一个数据结构中在重新发送他们之前。
buffer(bufferBoundaries) monitors an Observable, bufferBoundaries. Each time that Observable emits an item, it creates a new collection to begin collecting items emitted by the source Observable and emits the previous collection.
buffer(bufferBoundaries 缓冲区边界)监视一个Observable,bufferBoundaries。 每当Observable发出一个项目时,它将创建一个新的集合,开始收集源Observable发出的项目并发出先前的集合。
When it subscribes to the source Observable, buffer(bufferClosingSelector) begins to collect its emissions into a collection, and it also calls bufferClosingSelector to generate a second Observable. When this second Observable emits an item, buffer emits the current collection and repeats this process: beginning a new collection and calling bufferClosingSelector to create a new Observable to monitor. It will do this until the source Observable terminates.
当它订阅源Observable时,buffer(bufferClosingSelector)开始将其排放收集到一个集合中,并且还调用bufferClosingSelector来生成第二个Observable。 当这个第二个Observable发出一个项目时,buffer会发出当前的集合并重复这个过程:开始一个新的集合并调用bufferClosingSelector来创建一个新的Observable来监视。 它将这样做,直到源Observable终止。
(就是说整个的过程中有两个流,第一个流是用来发出真实数据的buffer流,并且在这个流中把数据组成一个buffer,而不是单独一个一个的发出,第二个流是一个用来发出信号的流,当这个发出信号的流发出信号时候,第一个流就开发向外面发出一个一个小的item的buffer集合)
buffer(bufferOpenings, bufferClosingSelector) monitors an Observable, bufferOpenings, that emits BufferOpening objects. Each time it observes such an emitted item, it creates a new collection to begin collecting items emitted by the source Observable and it passes the bufferOpenings Observable into the bufferClosingSelector function. That function returns an Observable. buffer monitors that Observable and when it detects an emitted item from it, it emits the current collection and begins a new one.
buffer(bufferOpenings, bufferClosingSelector) 监控一个Observable,bufferOpenings这个东西就是一个流,这个流发出了一个BufferOpening对象。每次它(应该指的是buffer运算符)观察一个item的发出,它
出创建一个新的集合用来收集源Observable并且传递一个bufferOpenings流到一个bufferClosingSelector函数中,这个函数会返回一个新的流,并且buffer运算符监控这个函数返回的Observable当buffer运算符探测到有元素从这个函数返回的流中发射出来,那么buffer运算符就把收集到一起的item发射出去,然后再次创建一个新的集合。
bufferWithCount(count) emits non-overlapping buffers, each of which contains at most count items from the source Observable (the final emitted buffer may contain fewer than count items).
bufferWithCount(count)发出不重叠的缓冲区,每个缓冲区最多包含来自源Observable的最多计数项(最终发出的缓冲区可能包含少于计数项)。
bufferWithCount(count, skip) creates a new buffer starting with the first emitted item from the source Observable, and a new one for every skip items thereafter, and fills each buffer with count items: the initial item and count-1 subsequent ones, emitting each buffer when it is complete. Depending on the values of count and skip these buffers may overlap (multiple buffers may contain the same item), or they may have gaps (where items emitted by the source Observable are not represented in any buffer).
bufferWithCount()的第一个参数声明一次打包需要的元素数量:
var source = Rx.Observable.timer(0,1000); //序列:0 1 2 3 4 ...
var target = source.bufferWithCount(3); //序列: [0,1,2] [3,4,5] ...
可选的第二个参数声明打包的步进数量,默认情况下,这个值等于第一个参数:
var source = Rx.Observable.timer(0,1000); //序列:0 1 2 3 4 ...
var target = source.bufferWithCount(3,1); //序列: [0,1,2] [1 2 3] [2 3 4] ...
// 注意最后这一句,这里的是 序列: [0,1,2] [1 2 3] [2 3 4] ...
bufferWithTime(timeSpan) emits a new collection of items periodically, every timeSpan milliseconds, containing all items emitted by the source Observable since the previous bundle emission or, in the case of the first bundle, since the subscription to the source Observable. There is also a version of this variant of the operator that takes a Scheduler as a parameter and uses it to govern the timespan; by default this variant uses the timeout scheduler.
bufferWithTime(timeSpan) 周期性的发射一个新的集合,每隔timeSpan时间,发射出在这个时间间隔内,从源Observable中发出的所有的item。从订阅源Observable开始的第一个bundle, 还有一个版本的操作符的这个变体,它将一个Scheduler作为一个参数,并用它来管理时间段; 默认情况下,此变体使用超时调度程序。
bufferWithTime(timeSpan, timeShift) creates a new collection of items every timeShift milliseconds, and fills this bundle with every item emitted by the source Observable from that time until timeSpan milliseconds has passed since the collection’s creation, before emitting this collection as its own emission. If timeSpan is longer than timeShift, the emitted bundles will represent time periods that overlap and so they may contain duplicate items. There is also a version of this variant of the operator that takes a Scheduler as a parameter and uses it to govern the timespan; by default this variant uses the timeout scheduler.
bufferWithTimeOrCount(timeSpan, count) emits a new collection of items for every count items emitted by the source Observable, or, if timeSpan milliseconds have elapsed since its last collection emission, it emits a collection of however many items the source Observable has emitted in that span, even if this is fewer than count. There is also a version of this variant of the operator that takes a Scheduler as a parameter and uses it to govern the timespan; by default this variant uses the timeout scheduler.
bufferWithTimeOrCount(timeSpan, count)运算符只要源Observable发射出了count个item之后,就发射一个新的集合。或者从上次发射到现在timeSpan时间过去了,那么就将当前的buffer发射出去,当然,可能当前的buffer中的元素个数没有达到count个。还有一个版本的操作符的这个变体,它将一个Scheduler作为一个参数,并用它来管理时间段; 默认情况下,此变体使用超时调度程序。
transform the items emitted by an Observable by applying a function to each item
通过对每个项目应用函数来转换Observable发出的项目
RxJS implements this operator as map or select (the two are synonymous). In addition to the transforming function, you may pass this operator an optional second parameter that will become the “this” context in which the transforming function will execute.
RxJS将此运算符实现为map或select(两者是同义词)。 除了变换函数之外,您可以将此运算符传递给可选的第二个参数,这将成为转换函数执行的“this”上下文。
The transforming function gets three parameters:
这个函数有三个参数
// Using a value
var md = Rx.Observable.fromEvent(document, 'mousedown').map(true);
var mu = Rx.Observable.fromEvent(document, 'mouseup').map(false);
// Using a function var source = Rx.Observable.range(1, 3) .select(function (x, idx, obs) { return x * x; });
var subscription = source.subscribe( function (x) { console.log('Next: ' + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); }); Next: 1 Next: 4 Next: 9 Completed
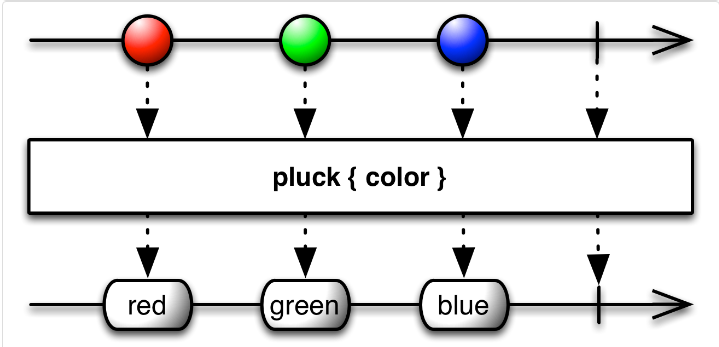
There is also an operator called pluck which is a simpler version of this operator. It transforms the elements emitted by the source Observable by extracting a single named property from those elements and emitting that property in their place.
还有一个名为pluck的操作符,这个操作符是一个更简单的版本。 它通过从这些元素中提取单个命名属性并在其中放置该属性来转换源Observable发出的元素。
var source = Rx.Observable .fromArray([ { value: 0 }, { value: 1 }, { value: 2 } ]) .pluck('value');
var subscription = source.subscribe( function (x) { console.log('Next: ' + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); }); Next: 0 Next: 1 Next: 2 Completed
blocking (To) / toArray / toMap / toSet
https://github.com/Christian-health/StudyNote2017/issues/11 这个页面上有 上面所有操作符号的详细解释