Closed counselorbot[bot] closed 2 years ago
🛑 There was an error: Error: You forgot to add your 'SONGREC_ENDPOINT' secret! 🛑
Go ahead and merge this branch to main
to move on. Great work finishing this section!
⚠️ If you receive a
Conflicts
error, simply pressResolve conflicts
and you should be good to merge!
Week 2 Step 6 ⬤⬤⬤⬤⬤⬤◯◯ | 🕐 Estimated completion: 10-15 minutes
Pose! Song Recommendation with Age Recognition
✅ Task:
Create a new Azure Function that parses a Twilio request body and returns the image url.
song4u
qs
song4u
that acts as a Twilio webhooksong4u/index.js
on thesong4u
branch and add your function url to a repository secret namedSONGREC_ENDPOINT
song4u
tomain
, and only merge the pull request when the bot approves your changes!🚧 Test Your Work
To test your work, try texting a jpg image to your Twilio number. You should receive a link back that contains the image.
❓ How do I find my Twilio number?
See your [phone numbers](https://www.twilio.com/console/phone-numbers/incoming).1: Creating a Twilio Account
In this project, the user will text an image to a Twilio number and an Azure function will analyze the image. The Face API will determine the age of the person in the image. We need to create a Twilio account first so that the user can text an image to your Twilio number.
Sign up for a free Twilio trial account here.
:question: What's the process like for signing up for Twilio?
- When you sign up, you'll be asked to verify your personal phone number. This helps Twilio verify your identity and also allows you to send test messages to your phone from your Twilio account while in trial mode. - Once you verify your number, you'll be asked a series of questions to customize your experience. - Once you finish the onboarding flow, you'll arrive at your project dashboard in the Twilio Console. This is where you'll be able to access your Account SID, authentication token, find a Twilio phone number, and more. Learn more about it [here](https://www.twilio.com/docs/usage/tutorials/how-to-use-your-free-trial-account)!After we get our account set up, we need to get a phone number, which is free from your trial account.
❓ Where can I get a phone number?
1. [Create a new project](https://support.twilio.com/hc/en-us/articles/360011177133-View-and-Create-New-Projects-in-Twilio-Console) on Twilio. 2. [Add a brand new number](https://www.twilio.com/docs/usage/tutorials/how-to-use-your-free-trial-account#get-your-first-twilio-phone-number) for your Twilio project, which will be used to send texts to users. Save this to use for later.Before you move on, be sure to create a new Azure function!
2: Configuring the Azure Function
Our new Azure function will act as a Twilio webhook.
You will need to place your function's URL into the settings on your Twilio account.
Do you like the command line more? Try using the Twilio CLI instead!
❓ How do I configure it?
1. Go to the [Twilio Console's Numbers page](https://www.twilio.com/console/phone-numbers/incoming) 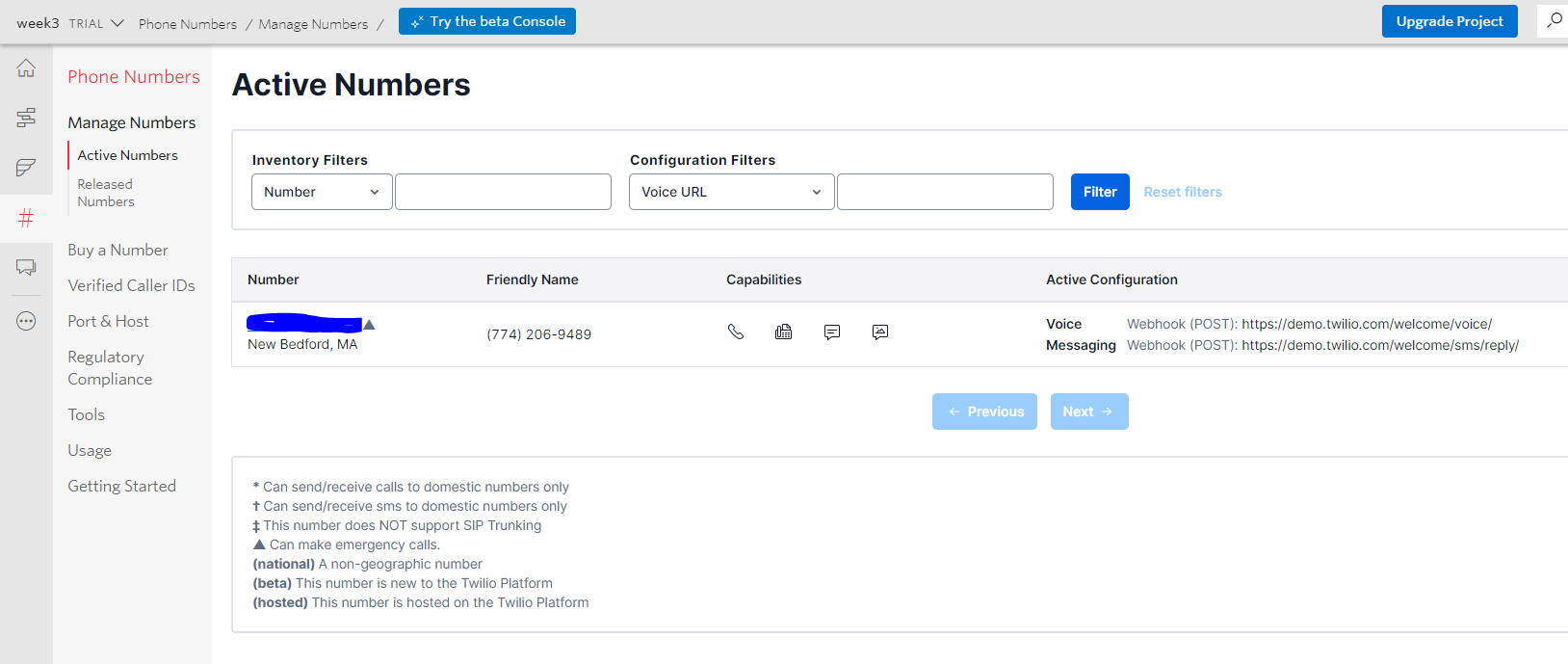 2. Click on the phone number you'd like to modify 3. Scroll down to the Messaging section and the "A MESSAGE COMES IN" option. 4. Paste in your Azure Function URL. Make sure to click `Save` afterwards!! 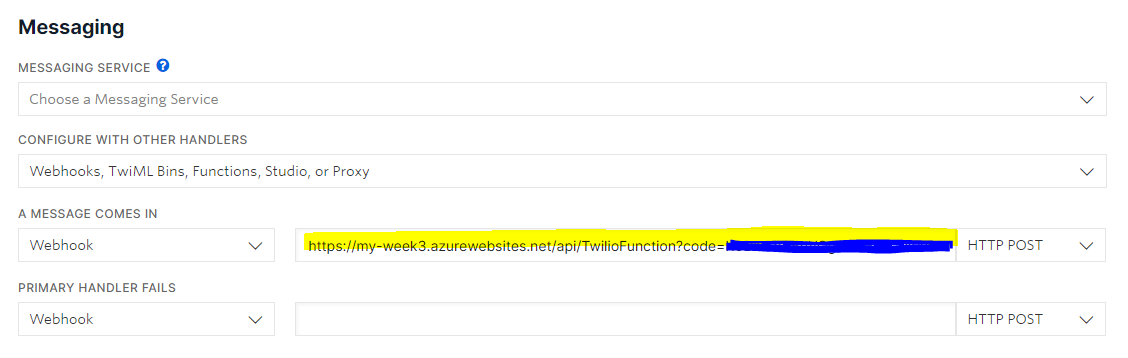3. Coding the Azure Function
Test the webhook out!
If you want more insight into how a webhook actually works, try this:
:exclamation: Log the request body in your console using `context.log(req.body)` and text your Twilio number.
Using this code: ```js module.exports = async function (context, req) { const reqbody = req.body context.log(reqbody) context.res = { // status: 200, /* Defaults to 200 */ body: reqbody }; } ``` You might get something like this: ``` ToCountry=US&MediaContentType0=image%2Fjpeg&ToState=MI&SmsMessageSid=MM0fe83458b74a1f626eb0da4685ab28b5&NumMedia=1...... ```In order to parse the parameters from those values you just saw, we need an npm package.
❓ How do you install qs
We need to install the npm package `qs`. This package parses **query strings**, and we will use it to parse the SMS sent by the user to the Twilio number so we can access the image sent in the text. > 💡 You only need to use `npm init -y` if you are installing npm packages for the first time on an Function App! As we did when we installed `parse-multipart`, we need to enter `npm init -y` (which initializes the package.json files) before we can install `qs`:Now, to use
qs
, first don't forget to initialize it!Then, let's parse the request body, which is held in
req.body
.From this outputted object, we'll find its
MediaUrl0
attribute and return it.:question: How do I return the output?
```js context.res = { body: queryObject.MediaUrl0 }; ```📹 Walkthrough Video