Open thetractor opened 3 years ago
Hello,
I believe this is not an issue, there is a bit of misunderstanding of how it works. Let me explain:
Basically what you are doing with this code router = APIRouter(route_class=versioned_api_route(1, 0))
is generating a new version, version 1 to be precise.
The same happen with this one router = APIRouter(route_class=versioned_api_route(2, 0))
, we create version 2.
The problem here is that you are specifying again version on the path e.g. "/users/v1"
so this URL will look like this: http://localhost:8000/v1/users/v1
When you are adding the new route /users/v2
, this is a completely different path as /users/v1
because remember, the versioning is already happening when we initialize APIRouter
When we create a new version all the routes from the past version will be replicated in the new version + the new routes with the new version
I am in the same situation. So there is no way to include in the new version all the routes of the new version + just the routes of the old version which are also referenced in the new one?
Up to now I ended up not to use fastapi_versioning at all, but creating separated APIRouter for the two versions.
@colibri17 To fix this I had to patch the library. In the file fastapi_versioning/versioning.py
I moved the line 50 to line 53 and it works flawlessly
Hello,
I have a sample app with two versions of the user endpoint. Version 1 has an endpoint and version 2 has an other endpoint. Now if I navigate to the docs version 1 shows all correct but version two shows both of the endpoints together (the endpoint from v1 and the endpoint from v2)
Here my code:
Here what I see in the docs when I navigate to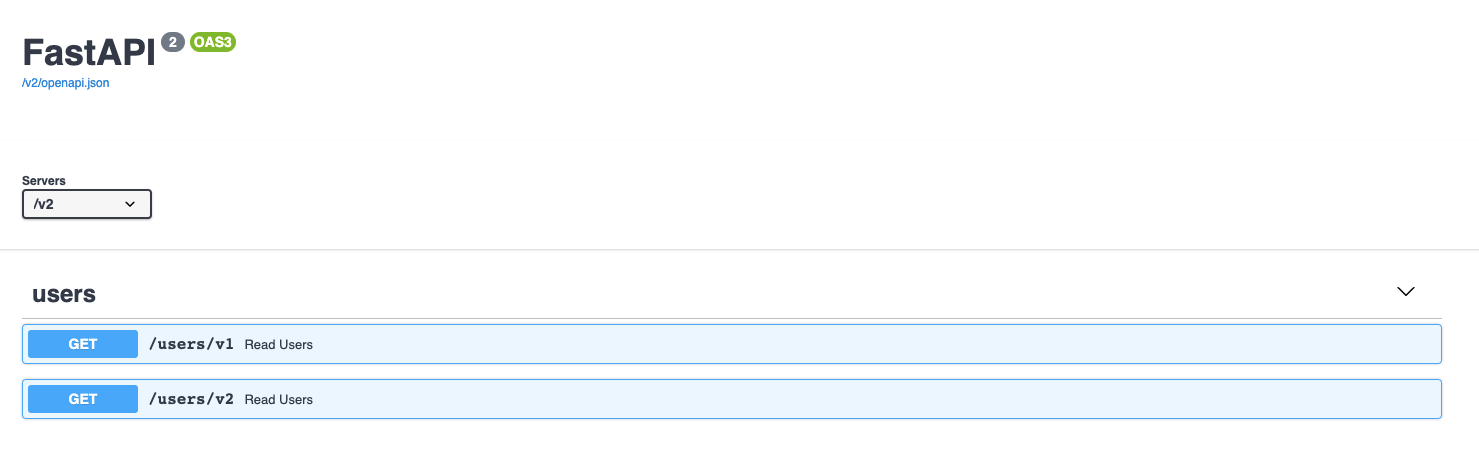
http://localhost:8000/v2/docs
I would expect the docs of version 2 to only show the endpoint defined in
app/v2/routers/users.py
and not a combination of both versions.Thanx for any help with this!