Open travisrroy opened 1 year ago
Well, I can see that the panel is sending RF device statuses with the status of 0x84 and since your bit mask is set to 0x80, it sees it as an open. Do you have a status with a close event so we can determine what the correct bit mask should be.
I think the heartbeat that rf devices send every 60 to 90 minutes is causing the issue. I'll check my code in how it handles the mask.
I've pushed a small update on dev. Just an update to vistalarm.h that has it ignoring RF heartbeat status messages for zone updates. See if that addresses your issue. I'd still like to see a close RF status as well to see if there isnt anything else at play here.
Ok, so I haven't updated yet, but I collected these logs. I'm not sure if they have any specific close events in them.
Door 1
Event 1
Event 2 (I believe this is me actually opening the door)
Event 3
Door 2
Ok, I see the issue. You should be using 0x20 as the mask and not 0x80. With rf sensors there are 4 possible loops that can be used and the corresponding masks would be 0x80, 0x40, 0x20 and 0x10. Your's has two loops defined. 0x80 is always on and 0x20 is what determine the open/close status (giving a on value of 0xa0 on and 0x80 off). I don't know the logic at this time as to how the loops are selected. The rf serial lookup is still experimental at this time. So change the mask value (3rd entry of each record) of each sensor to 0x20.
Ok. It looks like changing the mask fixed the issue. One question about the rf serial lookup is would I figure out the mask for motion sensors and smoke detectors?
Only way is to trigger then reset them and see what the value changes to in the RFX line in the log. It's a guessing game as I don't know the logic that determines it. I don't have enough data from other users to compare. I also do not have any rf sensors set up so can't test myself. I'm only working off a bench test system as far as the vista is concerned.
Ok, I tested the smoke detector. B0 when triggered and 00 when disarmed. Not sure how to determine the mask for that. Still waiting/trying to trigger the motion detector.
Edit: For documentation sake, I believe the smoke detector is a 5806W3.
Ok, so the PIR motion produces an 80 when triggered and 00 when cleared.
ok, Great. From what I see you can use 0x80 mask for the PIR and smoke detectors as they all set bit 7 (0x80) on and off. For the door sensors, you use 0x20 for the mask as bit 7 (0x80) of the status seems to always be on but bit 5 (0x20) is the one that toggles to indicate open/close status. Interesting though that the smoke sensor set bit 7, 5 and 4 on for active and 0 for idle. You only need to test for one of those bits so might as well use bit 7(0x80)
So setting the proper mask worked great. When I updated to the latest commit (75d02a6), I got a warning in the compiler and the doors were no longer working in HA. I downgraded back to 21fa8b5 and it works great again.
FYI, i've changed the way the RF devices are entered in the yaml to make it easier. It will use whatever info you have setup from program *56 for loop# and zone#. No need to worry about masks. Just enter the serial:loop#:zone#. You can see this document for default loop numbers for various devices: https://advancedsecurityllc.com/wp-content/uploads/5800%20Wireless%20Device%20List.pdf
Problem
I updated to the latest dev branch since I had some issues with the ESP32 disconnecting randomly after unexpected power loss and now I have a new problem. Basically, each door sensor will report to home assistant that it has been opened when it has not every 72 minutes or so since the last time it falsely reported it. Maybe something to do with
rfSerialLookup
? I do like this new feature though.Platform
Vista-20P, 6150RF, 6x 5816WMWH, ESP32, Home Assistant 2023.2.3
Config
Logs
I managed to catch these 3 logs from ESPHome.
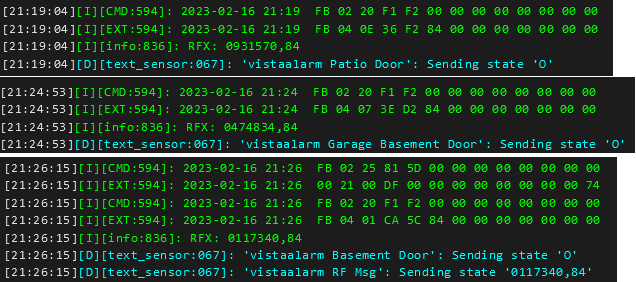