Closed allenmqcymp closed 5 years ago
Thank you for using cvui and reporting problems (particularly being your first time using it)! There is nothing wrong with your code, so this issue must be a cvui bug. I'll check on that.
Hi
There is some problem in coding.
please check below code
#include <opencv2/opencv.hpp>
#include "opencv2/imgproc/imgproc.hpp"
#define CVUI_IMPLEMENTATION
#include "cvui.h"
using namespace cv;
int main() {
// Create a frame where components will be rendered to.
cv::Mat frame = cv::Mat(250, 500, CV_8UC3);
cvui::init("test");
// create a trackbar
int bigN = 6;
bool checked = false;
while (true) {
// Fill the frame with a nice color
frame = cv::Scalar(49, 52, 49);
cvui::trackbar(frame, 110, 80, 250, &bigN, (int)2, (int)15);
cvui::checkbox(frame, 90, 50, "Example checkbox", &checked);
if (cvui::button(frame, 110, 150, "Find images!")) {
printf("clicked\n");
};
cvui::update("test");
// Update cvui stuff and show everything on the screen
cv::imshow("test", frame);
if (cv::waitKey(1) == 27) {
break;
}
}
return 0;
}
@prabhakarmp can you describe a bit better what is wrong with your code, please? The UI components are not working or anything different?
@Dovyski
int bigN = 6; bool checked = false;
These variables are declared as local variables which needs to be Global variable.
Since it is declared as local variables, UI results are not impacted because every time these variables getting updated with declared values.
Actually UI result will be there and it is valid till end of that cycle only. Suppose if we give big delay we can see the difference. And then in next cycle it will go to actual value what we had declared.
@prabhakarmp thanks! I do realize my mistake now. Closing this thread because the issue was due to my own bug and not CVUI's
Thank you for the help, @prabhakarmp! And I am glad @allenmqcymp 's code is working now. All good.
This is my first time using CVUI. I'm also a beginner to OpenCV as well as C++, so this "error" may just be my own stupidity. Regardless, I've had trouble getting started with CVUI and can't figure out why. All the items appear yet none of them respond to mouse clicks and drags.
CVUI Version: 2.7.0 OpenCV: 3.2.0 Running: Ubuntu 18.04.2 LTS x86-64
Here is the main.cpp file that essentially I'm trying to run. I build it using a makefile.
Here's the result: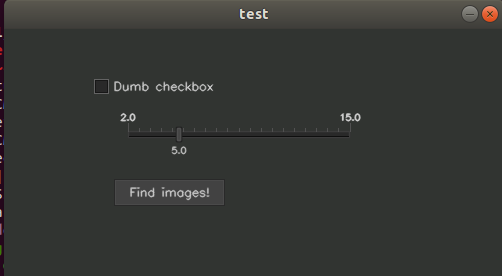
I can hover over the button and it changes shade - it doesn't respond to clicks though. The checkbox doesn't respond to clicks within the box. The trackbar does not move with mouse drag.
Thanks for your time Allen