Closed nsiatras closed 2 years ago
@nsiatras Result of polyline after intersection is probably a set of disconnected paths. Here is how to visit all paths and vertices in each path:
for (int ipath = 0; ipath < pLine.getPathCount(); ipath ++)
{
for (int i = pLine.getPathStart(ipath); i < pLine.getPathEnd(ipath); i++)
{
pLine.getPoint(i).getX();
pLine.getPoint(i).getY();
}
}
@stolstov Thank you very much for your reply
Edited: It works excellent and I can tell it is very fast! Thanks again!
@nsiatras If your intent to clip with axis-aligned rectangle (as clipping geometry for display on rectangular screen), then OperatorClip is more optimized for this.
Hello,
I am trying to clip a polyline. The input and result are as the following image. As you can see the result is not complete, it is exactly half.
Also see:
Before clipping: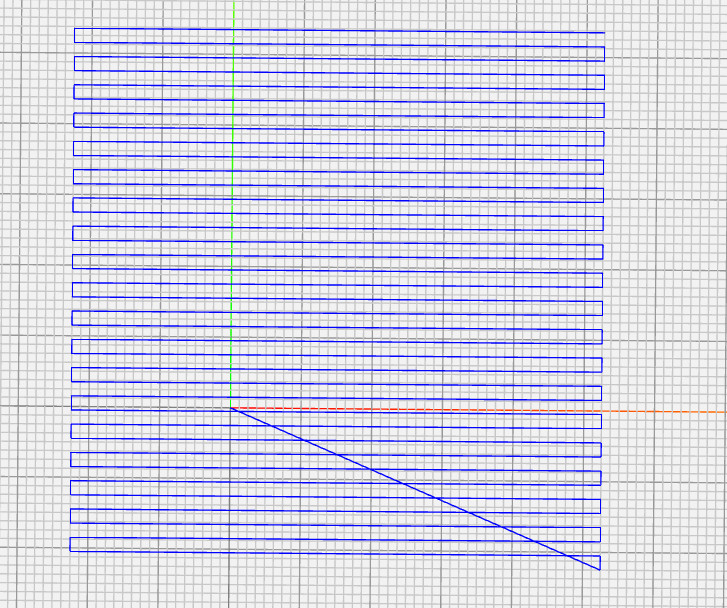
After Clipping: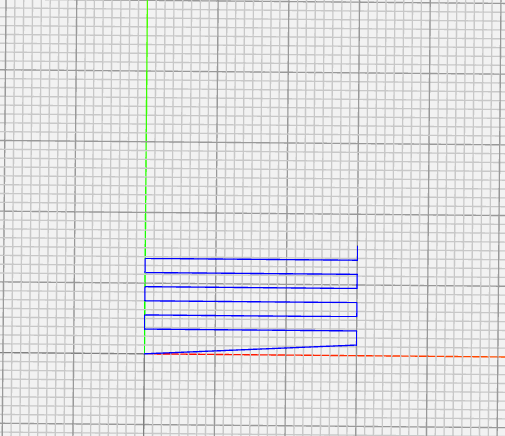
The code I am using is the following
All ideas are welcome