Open driggeraaliya94 opened 2 years ago
Hi @driggeraaliya94 Do you have any logs from ML backend?
Hi @KonstantinKorotaev,
Thank you for quick turn around. Below is the error which is displayed on terminal.
WARNING: This is a development server. Do not use it in a production deployment. [2022-08-22 10:33:35,419] [INFO] [werkzeug::_log::225] * Running on http://192.168.0.168:9090/ (Press CTRL+C to quit) [2022-08-22 10:34:08,865] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:08] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:08,876] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:08,876] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:08,876] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:08,876] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:08,877] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:08] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:33,940] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:33] "POST /webhook HTTP/1.1" 201 - [2022-08-22 10:34:34,024] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:34] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:34,030] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:34,031] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:34,031] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:34,031] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:34,031] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:34] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:34,231] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:34] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:34,235] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:34,235] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:34,235] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:34,235] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:34,235] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:34] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:34,248] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:34,248] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:34,248] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:34,248] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:34,248] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:34] "POST /predict HTTP/1.1" 200 - [2022-08-22 10:34:34,657] [ERROR] [label_studio_ml.model::get_result::58] Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 56, in get_result job_result = self.get_result_from_job_id(model_version) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 110, in get_result_from_job_id assert isinstance(result, dict) AssertionError [2022-08-22 10:34:36,458] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:36] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:36,460] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:36,461] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:36,461] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:36,461] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:36,461] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:36] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:36,476] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:36,476] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:36,476] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:36,476] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:36,477] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:36] "POST /predict HTTP/1.1" 200 - [2022-08-22 10:34:39,824] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:39,824] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:39,824] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:39,824] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:39,825] [ERROR] [label_studio_ml.exceptions::exception_f::53] Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/exceptions.py", line 39, in exception_f return f(*args, kwargs) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/api.py", line 70, in _train job = _manager.train(annotations, project, label_config, params) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 682, in train job_result = cls.train_script_wrapper( File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 638, in train_script_wrapper train_output = m.model.fit(data_stream, workdir, **train_kwargs) File "/home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/image_backend_simple/model.py", line 60, in fit raise KeyError(f'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled') KeyError: 'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled'
Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/exceptions.py", line 39, in exception_f return f(*args, kwargs) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/api.py", line 70, in _train job = _manager.train(annotations, project, label_config, params) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 682, in train job_result = cls.train_script_wrapper( File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 638, in train_script_wrapper train_output = m.model.fit(data_stream, workdir, **train_kwargs) File "/home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/image_backend_simple/model.py", line 60, in fit raise KeyError(f'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled') KeyError: 'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled'
[2022-08-22 10:34:39,825] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:39] "POST /train HTTP/1.1" 500 - [2022-08-22 10:34:39,855] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:39] "POST /webhook HTTP/1.1" 201 - [2022-08-22 10:34:40,006] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:40] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:40,009] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:40,009] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:40,009] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:40,009] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:40,009] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:40,010] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:40] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:40,027] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:40,027] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:40,027] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:40,027] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:40,027] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:40,027] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:40] "POST /predict HTTP/1.1" 200 - [2022-08-22 10:34:40,499] [ERROR] [label_studio_ml.model::get_result::58] Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 56, in get_result job_result = self.get_result_from_job_id(model_version) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 110, in get_result_from_job_id assert isinstance(result, dict) AssertionError [2022-08-22 10:34:40,549] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:40] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:40,553] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:40,553] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:40,553] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:40,553] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:40,554] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:40,554] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:40] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:40,564] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:40,564] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:40,564] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:40,564] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:40,564] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:40,564] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:40] "POST /predict HTTP/1.1" 200 - 4 labeled tasks downloaded for project 17 We'll return this as dummy prediction example for every new task: [] [2022-08-22 10:34:44,791] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:44] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:44,794] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:44,794] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:44,794] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:44,794] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:44,794] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:44,794] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:44] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:44,802] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:44,802] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:44,802] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:44,802] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:44,802] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:44,803] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:44] "POST /predict HTTP/1.1" 200 - [2022-08-22 10:34:48,281] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:48,281] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:48,281] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:48,281] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:48,281] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:48,282] [ERROR] [label_studio_ml.exceptions::exception_f::53] Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/exceptions.py", line 39, in exception_f return f(*args, kwargs) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/api.py", line 70, in _train job = _manager.train(annotations, project, label_config, params) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 682, in train job_result = cls.train_script_wrapper( File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 638, in train_script_wrapper train_output = m.model.fit(data_stream, workdir, **train_kwargs) File "/home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/image_backend_simple/model.py", line 60, in fit raise KeyError(f'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled') KeyError: 'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled'
Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/exceptions.py", line 39, in exception_f return f(*args, kwargs) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/api.py", line 70, in _train job = _manager.train(annotations, project, label_config, params) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 682, in train job_result = cls.train_script_wrapper( File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 638, in train_script_wrapper train_output = m.model.fit(data_stream, workdir, **train_kwargs) File "/home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/image_backend_simple/model.py", line 60, in fit raise KeyError(f'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled') KeyError: 'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled'
[2022-08-22 10:34:48,282] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "POST /train HTTP/1.1" 500 - [2022-08-22 10:34:48,318] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "POST /webhook HTTP/1.1" 201 - [2022-08-22 10:34:48,424] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:48,427] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:48,427] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:48,427] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:48,427] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:48,427] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:48,427] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:48,427] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:48,446] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:48,446] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:48,446] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:48,446] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:48,446] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:48,446] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:48,446] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "POST /predict HTTP/1.1" 200 - [2022-08-22 10:34:48,891] [ERROR] [label_studio_ml.model::get_result::58] Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 56, in get_result job_result = self.get_result_from_job_id(model_version) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 110, in get_result_from_job_id assert isinstance(result, dict) AssertionError [2022-08-22 10:34:48,941] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:48,944] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:48,944] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:48,944] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:48,944] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:48,944] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:48,944] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:48,944] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:48,963] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:48,963] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:48,963] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:48,963] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:48,963] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:48,963] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist Return output prediction: [] [2022-08-22 10:34:48,963] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:48] "POST /predict HTTP/1.1" 200 - 5 labeled tasks downloaded for project 17 We'll return this as dummy prediction example for every new task: [ { "value": { "choices": [ "Boeing" ] }, "id": "bYTVwy37cP", "from_name": "choice", "to_name": "image", "type": "choices", "origin": "manual" } ] [2022-08-22 10:34:52,031] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:52,089] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:52,125] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:52,126] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:52,126] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:52,126] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:52,126] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:52,126] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:52,126] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:52,160] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:52,160] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:52,160] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:52,160] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:52,160] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:52,160] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:52,160] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:52,184] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:52,208] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:52,218] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:52,218] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:52,218] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:52,218] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:52,218] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:52,218] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:52,219] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:52,224] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:52,228] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:52,228] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:52,228] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:52,228] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:52,228] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:52,228] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:52,229] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:52,248] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:52,249] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:52,249] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:52,249] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:52,249] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:52,249] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:52,249] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:52] "POST /setup HTTP/1.1" 200 - [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:55,442] [ERROR] [label_studio_ml.exceptions::exception_f::53] Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/exceptions.py", line 39, in exception_f return f(*args, kwargs) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/api.py", line 70, in _train job = _manager.train(annotations, project, label_config, params) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 682, in train job_result = cls.train_script_wrapper( File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 638, in train_script_wrapper train_output = m.model.fit(data_stream, workdir, **train_kwargs) File "/home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/image_backend_simple/model.py", line 60, in fit raise KeyError(f'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled') KeyError: 'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled'
Traceback (most recent call last): File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/exceptions.py", line 39, in exception_f return f(*args, kwargs) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/api.py", line 70, in _train job = _manager.train(annotations, project, label_config, params) File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 682, in train job_result = cls.train_script_wrapper( File "/home/ubuntu/.local/lib/python3.10/site-packages/label_studio_ml/model.py", line 638, in train_script_wrapper train_output = m.model.fit(data_stream, workdir, **train_kwargs) File "/home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/image_backend_simple/model.py", line 60, in fit raise KeyError(f'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled') KeyError: 'Project is not identified. Go to Project Settings -> Webhooks, and ensure you have "Send Payload" enabled'
[2022-08-22 10:34:55,443] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:55] "POST /train HTTP/1.1" 500 - [2022-08-22 10:34:55,479] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:55] "GET /health HTTP/1.1" 200 - [2022-08-22 10:34:55,484] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144695/job_result.json doesn't exist [2022-08-22 10:34:55,485] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144688/job_result.json doesn't exist [2022-08-22 10:34:55,485] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1661144679/job_result.json doesn't exist [2022-08-22 10:34:55,485] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660906281/job_result.json doesn't exist [2022-08-22 10:34:55,485] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660904993/job_result.json doesn't exist [2022-08-22 10:34:55,485] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902606/job_result.json doesn't exist [2022-08-22 10:34:55,485] [ERROR] [label_studio_ml.model::_get_latest_job_result_from_workdir::438] The latest job result file /home/ubuntu/Documents/ml_backend_19aug/label-studio-ml-backend/././image_backend_simple/17.1660722702/1660902588/job_result.json doesn't exist [2022-08-22 10:34:55,485] [INFO] [werkzeug::_log::225] 192.168.0.168 - - [22/Aug/2022 10:34:55] "POST /setup HTTP/1.1" 200 -
I see that /setup is returning 200 OK in your case. Are you sure that this log is from ML backend you are trying to connect?
Additional question: Do you use active learning?
By the way, if your training ended with error - you can clean your project folder with empty results. Just delete folder like this: ./image_backend_simple/17.1660722702/1660902588/ where you don't have any job_result file.
Hi,
I am getting a validation error when I try to connect my model.py to the label interface for image classification. Can anyone please help me with the issue.
This is my model.py file.
and below is my label interface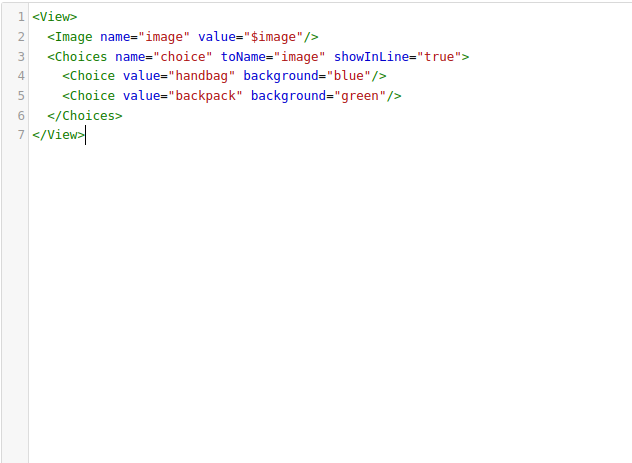
I am getting below validation error while trying to connect.