Open Helaas opened 3 years ago
Revisiting this, I notice I didn't make very clear when "SQLSTATE=PAERR SQLCODE=8012" occurs. When the temp storage reaches 1200 - 1400 MB, usually the job becomes unresponsive, but other times when a query (usually 'SET PATH = *LIBL'") is executed on the first statement after new dbconn() "SQLSTATE=PAERR SQLCODE=8012" occurs. After that point, every query will fail with this error until the job is restarted.
:wave: Hi! This issue has been marked stale due to inactivity. If no further activity occurs, it will automatically be closed.
Describe the bug When a bunch (north of 5000) queries have run inside the job, the job appears to run out of memory and refuses to create new connections until restarted. Temporary storage of the job also keeps increasing throughout the day. I can only suspect a memory leak.
To Reproduce Steps to reproduce the behavior: I have a back-end server implemented with Express.js that runs the entire day and is restarted at night. Normally it works fine, but a couple of days a month, everybody in the company uses the tool it powers at the same time, and it can receive 10 hits per second. During those busy days, it looks like the job eventually runs out of temporary storage. This appears to be caused by the statements (or the connections?) allocating memory and never deallocating? If this is caused by poor coding from my side, I would like to apologize for wasting your time, I usually stick to RPGLE :-).
I have also included a function that uses idb-pconnector, as there the problem seems to be much worse. This could provide useful clues when figuring out what happens I guess.
When ran using idb-connector [await executeStatement(sSql)]: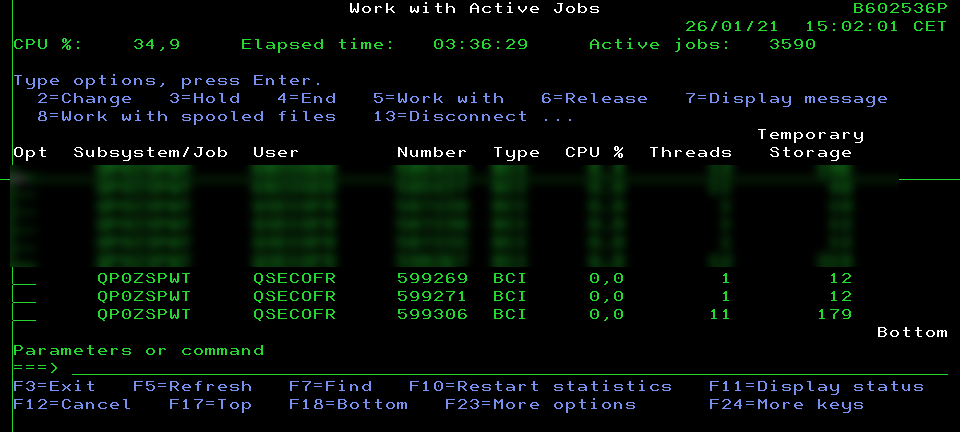
When ran using idb-pconnector [await executeStatementConnectorP(sSql)]:
My implementation with pconnector uses about 30% more temp storage after completing 1000 statements and runs slightly slower.
When a node job reaches 1200 - 1400 MB of temp storage, it tends to crash on our machine. Is this caused by poor coding on my side, or is this some sort of memory leak?
Expected behavior All memory cleaned up after completing a query.
Screenshots See above.
-- idb-connector@1.2.10 deduped
-- itoolkit@1.0.0 `-- idb-connector@1.2.10 dedupedThank you for your time 👍