Closed ghost closed 3 years ago
Sorry don't know. Did you ever figure this out?
This issue is related to this https://stackoverflow.com/questions/65669259/keep-getting-session-out-with-oidc-js-client-and-identity-server-4-authorization But I have not found the solution for it.
the endsession endpoint is due to a call to signoutRedirect -- so find out where your code is calling that?
Account controller
Startup.cs
Using Identity server 4 with OIDC JS client in every 10 seconds the application reloads, in the network tabs I can see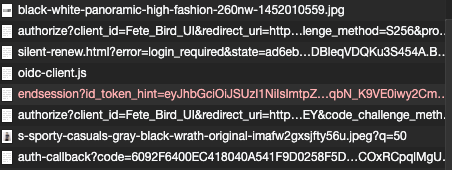
The end session URL is always been canceled and application reload frequently
Request URL: https://falconidentityserver.azurewebsites.net/connect/endsession?id_token_hint=eyJhbGciOiJSUzI1NiIsImtpZCI6IjU1RDY5MTZFODVCOUNENTgwRjQ0RTMzNzREMjZFOUFCIiwidHlwIjoiSldUIn0.eyJuYmYiOjE2MTAzNzU0NjQsImV4cCI6MTYxMDM3NTc2NCwiaXNzIjoiaHR0cHM6Ly9mYWxjb25pZGVudGl0eXNlcnZlci5henVyZXdlYnNpdGVzLm5ldCIsImF1ZCI6IkZldGVfQmlyZF9VSSIsImlhdCI6MTYxMDM3NTQ2NCwiYXRfaGFzaCI6IlVDaUt3LXBBdi14aFVINFRZVXk1a1EiLCJzX2hhc2giOiI5clc3UUY5ZEtqRXdubE9lTWpRTTVBIiwic2lkIjoiQjYwNEJBNEU5MTEyOURCQjYzNTJFOEJDNDZFQUM5QkUiLCJzdWIiOiJlNDEzMTIwYS0yYWEzLTQzZTktYTQ1MC1lZWU2NzBjY2EzMjEiLCJhdXRoX3RpbWUiOjE2MTAzNzU0NTEsImlkcCI6ImxvY2FsIiwiYW1yIjpbInB3ZCJdfQ.lmfq1ZJ2ukkrZ-FPjtCPaEsBYM0HXCAF496dNGMH0WP-SBFbbSllLSPGcavpruzzA0n-JQmkshtEqhyMvk5-c81dQLgjblrQ-X5QrzGoRd6fXDMnWwR0dlm2ZC2TcPOcBJXdaW1nfjLChxxrQljHMNLBr1tAmPfTfx0kaG7uvoXg1iY1aNmBsFUs4erdYs24Wd0JPtGzlHrGc3wyXk7aJNS77Ocu9SHUTL5XLsecOPMX0CVqeAX0ibRT6b-VuJ6u0egMKzR6yS8vCx4DNdHIScuX-zyMvisDePiCwqbN_K9VE0iwy2CmNfMKmioPX-aora7V1qZwCdj2-Lp-OSVVwg
Identity server client setting
OIDC client setting
I don't know what I am missing and where is the issue. Can anyone help me with the this issue
I am doing silent reniew and it is working fine as you can see in the network tab.
OIDC usermanager logs