Closed Que2701 closed 3 years ago
Is there a record in sessionStorage for the user's session? If so, then as far as oidc-client is concerned the user is logged in.
@brockallen yes there is ( unfortunately for me ), the issue is persisting the user object from identity server into the angular app layer.
Then that will be lost when the user hits refresh or you have page transitions
@brockallen okay I hear you, so what I did; I just went to sessionStorage
and retrieved the user from there and no more endless loop
isLoggedIn(): boolean{
this.user = JSON.parse(sessionStorage.getItem("oidc.user")) as User;
return this.user != null && !this.user.expired;
}
I have an angular 10 app running on localhost:4200. In my app-routing.module.ts I have configured root path to load the AdminComponent, so if you hit localhost:4200 it will try to load AdminComponent but before it does; I have a authguardservice that checks to see if the user is logged in and if not; it redirects the user to identity server (where he gets a login screen to get the token).
I do not have control on the identity server provider, it is hosted by another company
The connection is to IdentityServer4 with code flow and PKCE.
I researched quiet a bit on this issue, and a lot of people faced the same issue. however I could not find a solution that works for me. probably because I am new to angular and oidc? https://github.com/damienbod/angular-auth-oidc-client/issues/180 https://github.com/damienbod/angular-auth-oidc-client/issues/829 https://stackoverflow.com/questions/57574867/oidc-client-infinite-loop-when-calling-signinredirect
Here is my setup
app-routing.module.ts
auth-guard.service.ts
auth.service.ts
When I run the app on localhost:4200 I immediately get redirected to identity server, which is good. Punch in email and password and then identity server redirects to signin-callback.html page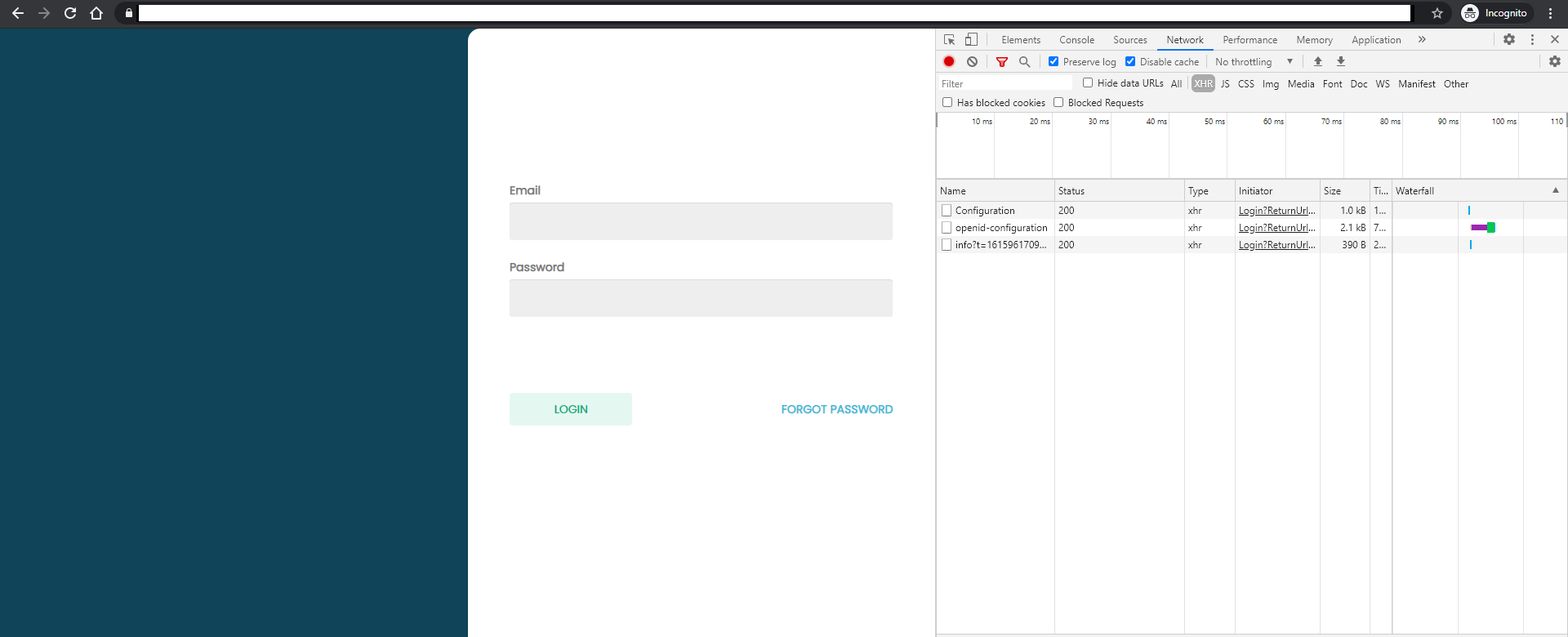
The user object has a id_token value as you can see here in the snipped below. and this is also good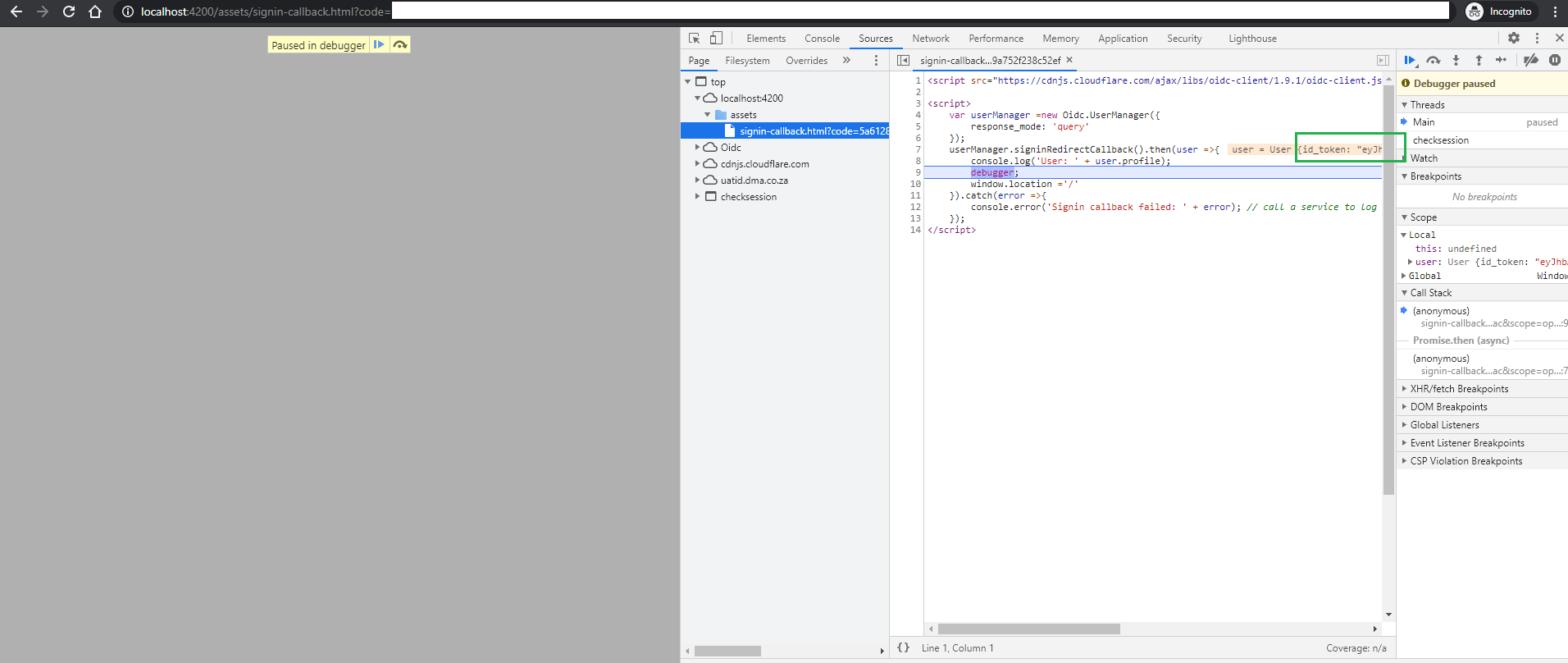
And in the network tab of the developer tools, I can see the token is there in the response section , refer to snipped below. so all good so far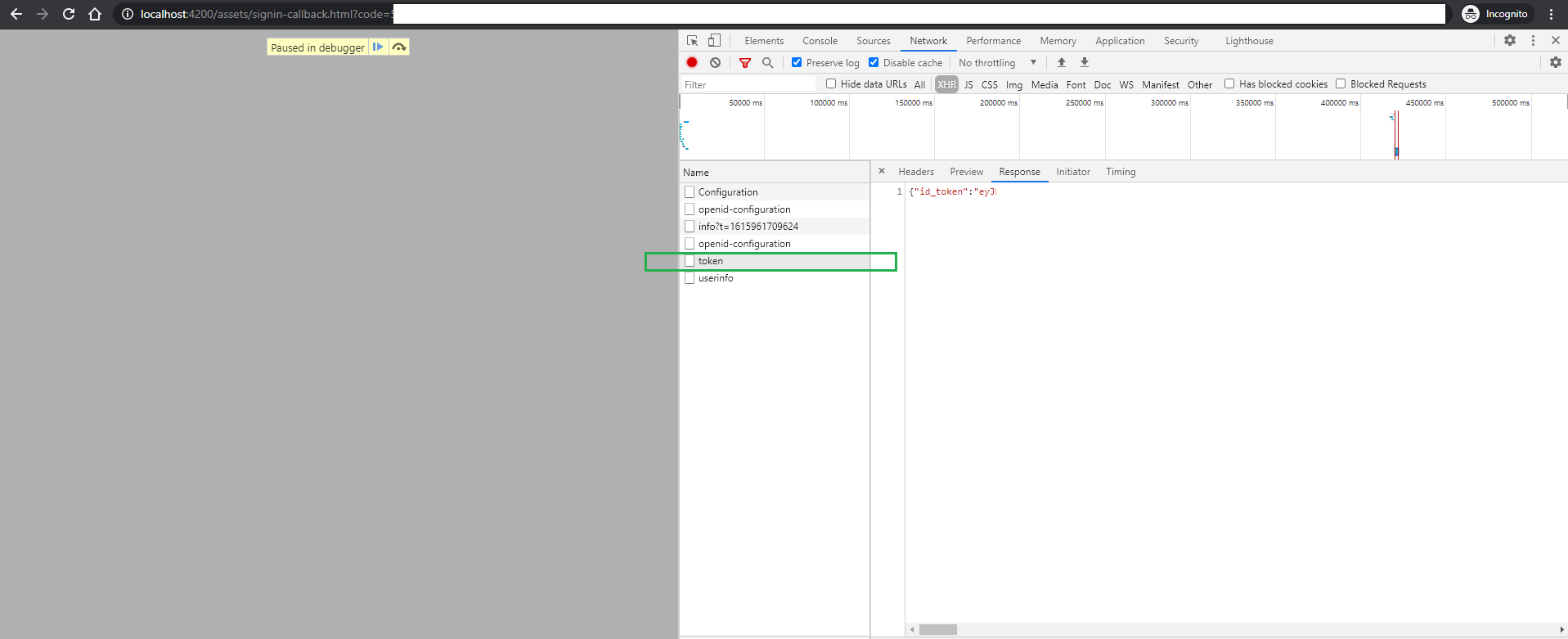
Also in my auth.service.ts constructor the identity server user gets assigned to the property user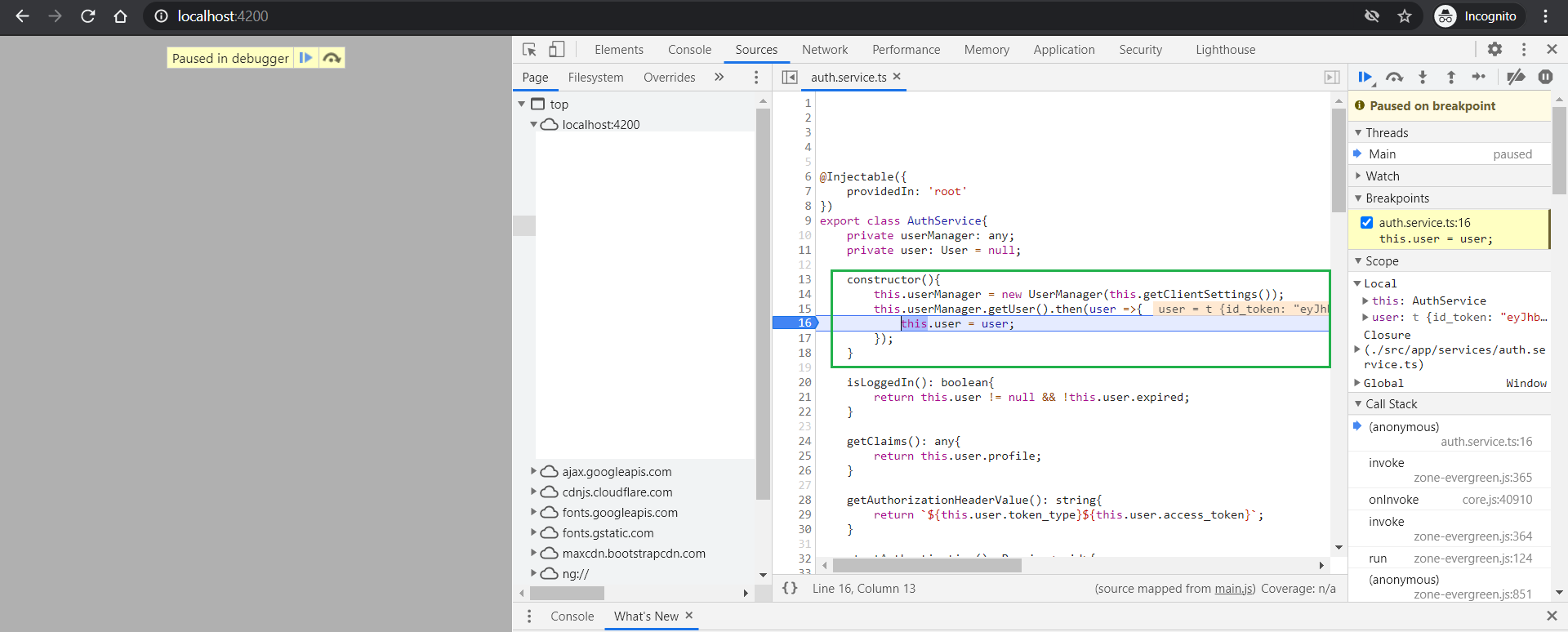
HERE's THE ISSUE: After identity server authenticates and sends us back a user, the signin-callback.html page then routes to "/admin" and here, the authguardservice runs again and tries to check if user is logged in, by calling the isLoggedIn() method of the authservice . (as you can see below)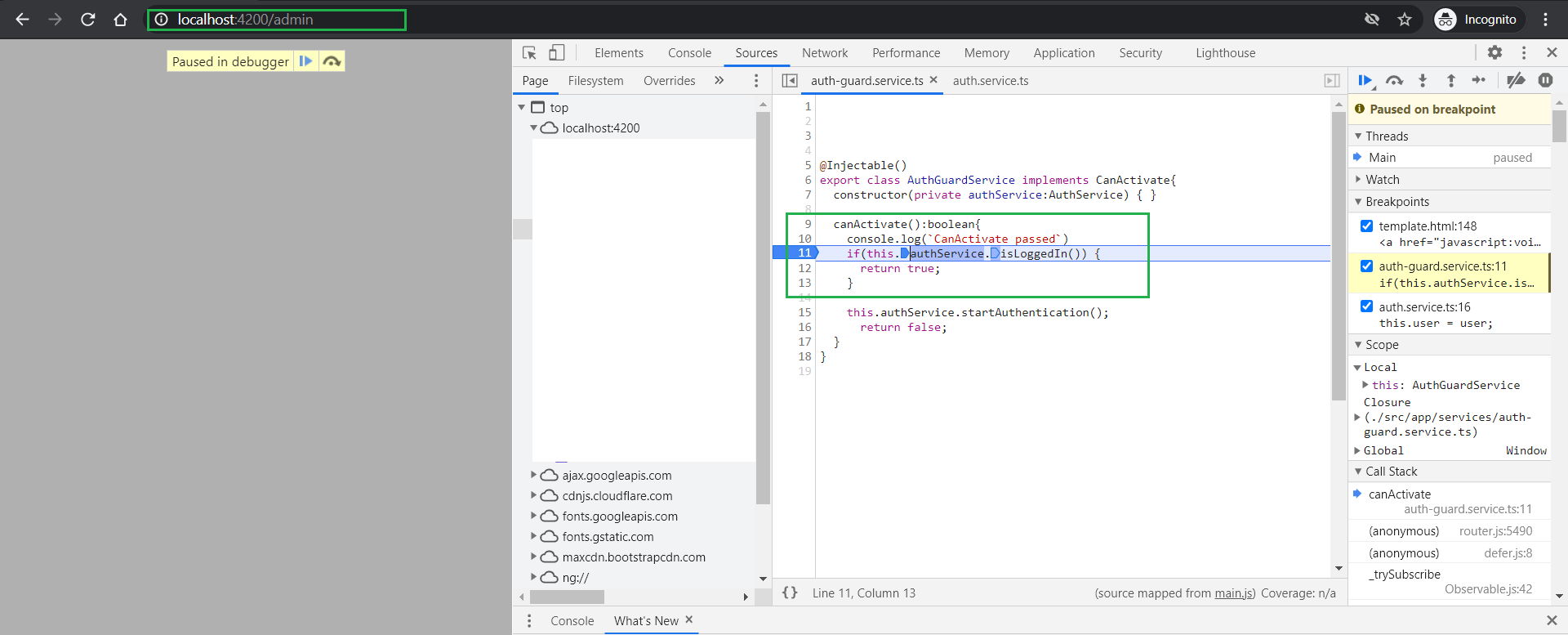
The only thing that
isLoggedIn()
method does, is check if theuser
object is null or not. and my surprise it is null :( even though we've recently set it's value in the constructor of the authservice