Closed BarRae closed 4 years ago
I'm not sure I fully understood:
"but using this it seems like my Z is equal to the depth to the object and that is not what I need"
Looking at the code, it seems correct.
If it computationally feasible, I would recommend using rs2::align
to bring the two frames into single viewport. This should simplify the problem significantly (we have two examples on alignment - align and align-advanced)
The thing I meant is like this: I am going to send the X, Y, Z coordinates to a robotic arm, so it can pick up the marble. For this I need the X, Y, Z like in the sketch I drew.
I tried to get this using rs2_deproject_pixel_to_point(berrycord, &depth_intrin, dpt_tgt_pixel, distance);
, but this gives me a Z which is equal to the depth value.
Or am I misunderstanding what get_distance()
gives me, is that value already the Z value?
Hi @BarRae
get_distance
is providing distance from the camera plane, not camera origin, so I do believe it is the Z value you are looking for.
That said, I do think the application can be simplified using the following pseudocode:
pipe.start()
align align_to(RS2_STREAM_COLOR);
while(auto fs = pipe.wait_for_frames())
{
fs = align.process(fs);
auto color_frame = fs.get_color_frame();
auto depth_frame = fs.get_depth_frame();
auto dpt_tgt_pixel = find_center_using_opencv(color_frame);
auto distance = depth_frame.get_distance(dpt_tgt_pixel);
rs2_deproject_pixel_to_point(berrycord, &depth_intrin, dpt_tgt_pixel, distance);
}
berrycord[2]
will hold distance from camera plane.
berrycord[0]
and berrycord[1]
will hold X and Y in relation to the color sensor. If you initialize align with RS2_STREAM_DEPTH
they will hold X and Y offsets in relation to the depth sensor (left infrared camera).
Thank you @dorodnic
This was the information I was looking for. I will probably do some testing with the camera today to check if the coordinates that the program gives me are correct enough. I will also consider your code advice.
Again, thank you.
Hello all,
I am currently working on a vision system to detect blue marbles. I am able to detect 1 marble from each picture. I now want to know the X,Y,Z coordinates of this marble. Here are is the image I use for detection: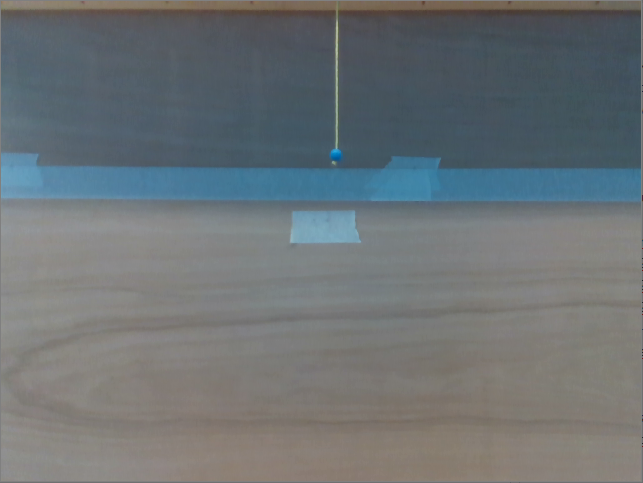
Here is the output with the detected marble: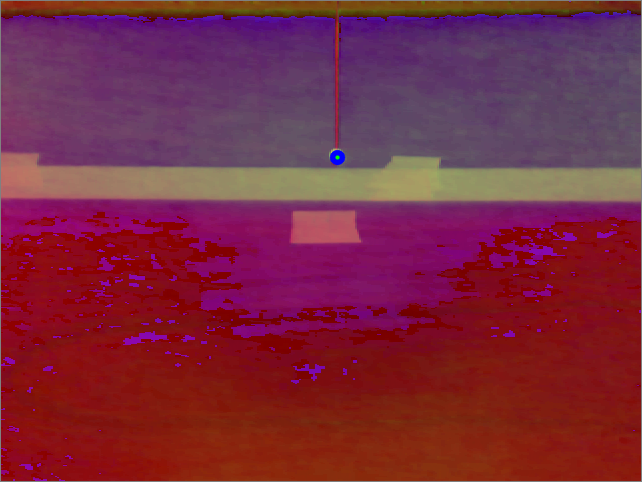
And here is my console output:
In my code I get the X,Y of the centre of the marble in the image and the distance to the marble.
The thing what I now need is The X, Y, Z coordinate of the centre of the marble. I tried doing this by using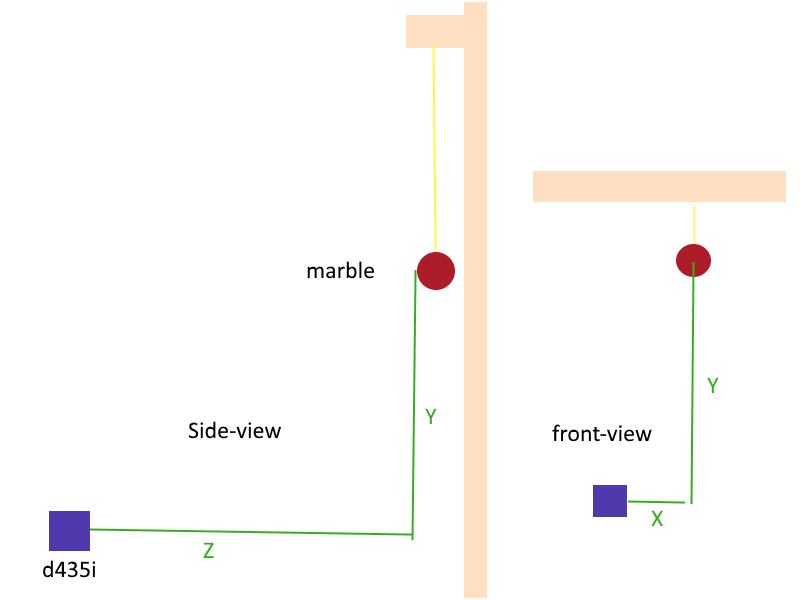
rs2_deproject_pixel_to_point(berrycord, &depth_intrin, dpt_tgt_pixel, distance);
but using this it seems like my Z is equal to the depth to the object and that is not what I need. See illustration below for the value of X, Y and Z that I would like to get:Would anyone be able to help me with this? Last week I though I got it but now i'm seriously doubting the correctness of my Z value
This is the code I currently use for this: