Closed jussiks closed 2 years ago
Inheriting from QDoubleSpinBox fixes the font size and button placement issues mentioned in #214. However, for some reason on Windows the arrow buttons appear smaller than in regular spin boxes (see picture below). I have not figured out the cause yet.
I think this is caused by the whole input field being shorter. Normal fields are 20 px tall, while ScientificSpinBox
fields are 16 px tall.
Of course we could disable scrolling altogether by monkey patching the event in the base class (QAbstractSpinBox.wheelEvent = lambda _, e: e.ignore()) but that seems rather questionable...
A monkey patch would be fine by me. Not the most elegant solution, but at least it would be applied globally, without the need to manually call a function for each dialog/widget etc.
I also noticed that when disabled, ScientificSpinBox
is a different shade of gray than the normal spinboxes:
This is a really minor issue, so if there's no easy solution to it, I don't think it's worth it to spend too much time trying to fix.
Other than these minor issues, looks good to me.
I think this is caused by the whole input field being shorter. Normal fields are 20 px tall, while
ScientificSpinBox
fields are 16 px tall.
I fixed this by setting the value of the spin box before setting its validator. Validator overrides the stylesheet of the element which somehow breaks things if the value has not yet been set.
A monkey patch would be fine by me. Not the most elegant solution, but at least it would be applied globally, without the need to manually call a function for each dialog/widget etc.
Added a function that disables scrolling. It gets called when Potku
instance is initialized.
I also noticed that when disabled,
ScientificSpinBox
is a different shade of gray than the normal spinboxes:
This is because the validator sets the background color to solid white. I am not sure how to fix this while still allowing the validator to change the color.
EDIT: I realized that my sizing "fix" did not actually work in the Request Settings dialog. It only worked for the reference density spin box in Global Settings dialog. I'll have to dig a bit deeper on this one.
b89f6c7 is a quick-and-dirty solution to the sizing problems on Windows.
A better solution could be to set all CSS rules in a single global style sheet when the application starts. We could then use attribute selectors to change only a single property like the background-color
instead of overriding the entire style sheet every time a value changes:
def main():
app = QtWidgets.QApplication(sys.argv)
css = """
QWidget[validation_status="acceptable"] {
background: #ffffff
}
QWidget[validation_status="intermediate"] {
background: #ebde34
}
QWidget[validation_status="invalid"] {
background: #f6989d
}
"""
app.setStyleSheet(css)
window = Potku()
window.show()
sys.exit(app.exec_())
def set_input_field_intermediate(input_field: QtWidgets.QWidget) -> None:
input_field.setProperty("validation_status", "intermediate")
input_field.style().polish(input_field)
This would make it easier to manage and customize the looks of the GUI. But this idea goes beyond the scope of this PR.
At least one more thing needs fixing: user should be able to type in zeroes after the last decimal place. This is currently not possible because trailing zeroes are immediately removed from the input. Changing the value from 1.0e+10
to 1.0001e+10
is a pain, because the user needs to first change the value to 1.01e+10
before they can fill in the rest of the zeroes.
This PR addresses the issue #214.
ScientificSpinBox
is now aQDoubleSpinBox
that implements its ownstepBy
andtextFromValue
methods to increase/decrease values and to format them into scientific notation.Inheriting from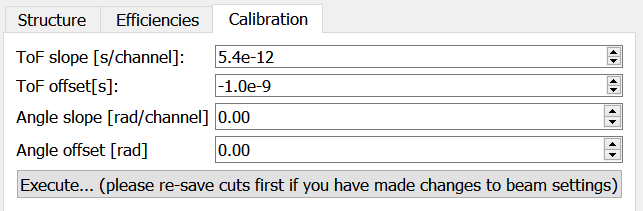
QDoubleSpinBox
fixes the font size and button placement issues mentioned in #214. However, for some reason on Windows the arrow buttons appear smaller than in regular spin boxes (see picture below). I have not figured out the cause yet.This change also makes ScientificSpinBoxes scrollable which was not actually desired. It seems that the only way to make a spin box unscrollable is to ignore the mouse wheel event:
This would be a simple addition to
ScientificSpinBox
but then we would once again have a spin box that behaves differently than other spin boxes. Of course we could disable scrolling altogether by monkey patching the event in the base class (QAbstractSpinBox.wheelEvent = lambda _, e: e.ignore()
) but that seems rather questionable...I removed the .ui file that we no longer need, and a Validator class that had been out-of-use for some time now.
I used hypothesis library to generate input values in some test cases. This library allows creating more robust property based tests (i.e. tests like "after n up steps and n down steps the value should of the spin box should stay the same") so I think it is a worthwhile addition to the project dependencies. Pipfile and Pipfile.lock have been updated. To install the new library, you need to run
pipenv install --dev
. I also updated README to include the --dev option when installing dependencies.Changes have not been tested on Mac.
Edit: forgot to mention pressing the PageUp key now increments the value by 10 steps (i.e. 1.234e10 => 2.234e10) and pressing PageDown decreases it by the same amount.