Closed asmwarrior closed 5 months ago
As always, the DXF documentation establishes the format of the code-value pairs in a DXF entity, but leaves it up to implementation to decide the meaning of the values. I have seen all sorts of different interpretations of SOLID entities, all the way up to 3D triangular meshes.
I have chosen a very simple interpretation that serves my current purposes, visualizing the results of a 2d packing engine used to cut plywood panels from larger plywood sheets ( https://github.com/JamesBremner/pack2 ) I have added code documentation describing this interpretation ( bc51ec0bf00ae295d6c3bb260bfd2b236f3fdc9e )
What is your purpose for using DXF-Viewer?
If you wish to contribute a different SOLID entity parser, perhaps along with a different drawing method, please let me know and I will add some infrastructure to switch between parsers.
I think we do need all four points to have a universal result for SOLID. Because it looks like SOLID is designed to parse with three vertices(triangle) as a stride and a index list of [0,1, 2, 1, 2, 3]. See the image below
And as the doc describes,
Fourth corner. If only three corners are entered to define the SOLID, then the fourth corner coordinate is the same as the third.
So maybe there's even no need to switch parser between rectangle and triangle.
I think we do need all four points to have a universal result for SOLID.
No such thing is possible. There are many different interpretations of the DXF format out there. You will have noticed that when you use different viewers for a DXF file, some will render it correctly and others will be confused. Each generator and viewer pair need to decide and agree on what they want to do. There is no such thing as a universal DXF Viewer capable of correctly rendering every DXF file.
What is your purpose for using DXF-Viewer?
Mostly, interests.
I used the DXF_Viewer in a wxWidgets based project to show a 2D DXF file. The project does not need to show the SOLID entity. But as I see you have added this SOLID support, I think we should make the SOLID support feature rich. I have tested the DXF_Viewer to show the test dxf file in https://github.com/JamesBremner/DXF_Viewer/blob/master/dxf/HFP1JZ.dxf
,and I see that HFP1JZ.dxf contains triangle arrows which is SOLID type, but current implementation of the DXF_Viewer does not support it.
If you wish to contribute a different SOLID entity parser, perhaps along with a different drawing method, please let me know and I will add some infrastructure to switch between parsers.
I think at least 3 points and 4 points SOLID 2D entity is needed, and hope I can contribute to it.
OK, I will add infrastructure to enable different SOLID parsers.
I have left you to actually implement the parsers!
This is the result if I open the solid.dxf in this issue and with my pull request https://github.com/JamesBremner/DXF_Viewer/pull/25
I have added your solid.dxf to the repo, renamed solidtid23.dxf
Are you working on this?
Are you working on this?
Not yet, but I will do it in the next days. I'm considering the condition that a solid may have either:
So, I may need a counter to remember how many points we have parsed.
Please do not do it that way.
IfmyParser == eParser::solid_2point
then 2 point solids are expected, if myParser == eParser::solid_4point
the 4 point solids are expected.
Add a case to https://github.com/JamesBremner/DXF_Viewer/blob/abefc7a8be3220f3fd8425a7072d08af06411b25/src/Solid.cpp#L52
Please do not do it that way.
If
myParser == eParser::solid_2point
then 2 point solids are expected, ifmyParser == eParser::solid_4point
the 4 point solids are expected.Add a case to
OK, pull request is created, see here: https://github.com/JamesBremner/DXF_Viewer/pull/26
I have refactored the SOLID parser slightly to make it clearer what the while loop was doing ( since I confused myself over that! ) and updated the documentation
Perhaps you could add documentation for your 4-point parser?
I have refactored the SOLID parser slightly to make it clearer what the while loop was doing ( since I confused myself over that! ) and updated the documentation
Perhaps you could add documentation for your 4-point parser?
See this pull request: document the 4-point parser of the solid by asmwarrior · Pull Request #27 · JamesBremner/DXF_Viewer
Is there a reason this issue is still open?
Is there a reason this issue is still open?
Hi, thanks. I'm still using this library. I think this feature request can be closed. Because it is implemented.
Good to know you still find this useful. It seems a relatively mundane app but apparently gets more users than anything else I have.
One thing I like to mention is that when I export dxf file from autocad or other tool, this library can not handle dxf files contains splines. This is a big issue I see those years.
Please open an issue for this and provide a small, simple example of a DXF file that fails. Ensure that you do NOT have SPLINE with fit AND control points.
Hi, I see recently, reading the solid type of DXF is introduced.
I just did some test and found some issues here.
First, I create a dxf file for test by using the python's
ezdxf
library. Here is the code:I open the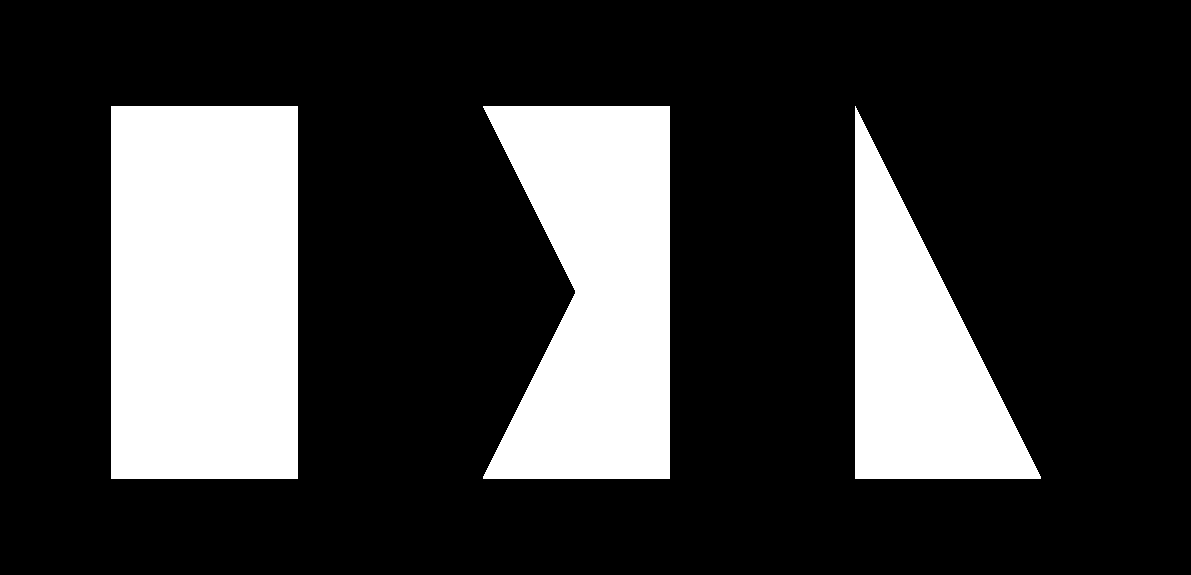
solid.dxf
under the liberCAD software, and shows such result image:You can see, the image shows three elements. For a normal rectangle(the first element), we should take the 4 points like:
(x, y), (x + width, y), (x, y + height), (x + width, y + height)
sequence.If we pass the point sequence like
(x, y), (x + width, y), (x + width, y + height), (x, y + height)
which shows in middle of the screen shot. That may be the solid we are not expected.Now, back to DXF_Viewer's code, in the file src\Solid.cpp, there are some code like below:
https://github.com/JamesBremner/DXF_Viewer/blob/e476b55acaad3f1e0ac22ab04b2dfd1303d827eb/src/Solid.cpp#L43-L68
It only read the first point and the third point, which is not enough to reconstruct the rectangle.
solid.zip
I have upload the solid.dxf in the solid.zip file.
And here is the suggested code changes: 1, it looks like the whole 4 points are needed. 2, the points sequence should be considered, for example, in the first element, the dxf shows such text:
To draw a normal rectangle, at least we need two points, which is the first point
(150,250)
( (x, y)), and the fourth point(160, 270)
((x + width, y + height)).Here are some reference: Solid — ezdxf 0.11.1 documentation
Help: SOLID (DXF)