Open harikanammi opened 5 years ago
have you solve above one. if yes then please share us.
Not solved!!!!
If you want a different output in your axis, you would give a different function for the formalLabel property.
What do you want to change them to?
To Change X and Y axis value use object to store the data:
import React from 'react'; import {SafeAreaView, StyleSheet, ScrollView, View} from 'react-native'; import {BarChart, Grid, XAxis, YAxis} from 'react-native-svg-charts'; import * as scale from 'd3-scale'; import {Text} from 'react-native-svg'; class App extends React.Component { render() { const data = [ { value: 50, labelX: 'A', labelY: 'One', }, { value: 10, labelX: 'B', labelY: 'Two', }, { value: 40, labelX: 'C', labelY: 'Three', }, { value: 95, labelX: 'D', labelY: 'Four', }, { value: 85, labelX: 'E', labelY: 'Five', }, ];
const CUT_OFF = 20;
const Labels = ({x, y, bandwidth, data}) =>
data.map((item, index) => (
<Text
key={index}
x={x(index) + bandwidth / 2}
y={item.value < CUT_OFF ? y(item.value) - 10 : y(item.value) + 15}
fontSize={14}
fill={item.value >= CUT_OFF ? 'white' : 'black'}
alignmentBaseline={'middle'}
textAnchor={'middle'}>
{item.value}
</Text>
));
return (
<>
<SafeAreaView>
<ScrollView
contentInsetAdjustmentBehavior="automatic"
style={styles.scrollView}>
<View style={{flexDirection: 'row', height: 200}}>
<View style={{width: 50, flexDirection: 'column'}}>
<YAxis
data={data}
scale={scale.scaleBand}
svg={{
fill: 'grey',
fontSize: 20,
}}
style={{flex: 1}}
min={0}
yAccessor={({index}) => index}
formatLabel={(_, index) => data[index].labelY}
/>
<View style={{flex: 0.1}} />
</View>
<View style={{flex: 1, flexDirection: 'column'}}>
<BarChart
style={{flex: 1}}
data={data}
gridMin={0}
yAccessor={({item}) => item.value}
svg={{fill: 'rgb(134, 65, 244)'}}>
<Labels />
<Grid direction={Grid.Direction.HORIZONTAL} />
</BarChart>
<XAxis
data={data}
scale={scale.scaleBand}
svg={{
fill: 'grey',
fontSize: 20,
}}
min={0}
style={{flex: 0.1}}
yAccessor={({index}) => index}
formatLabel={(_, index) => data[index].labelX}
/>
</View>
</View>
</ScrollView>
</SafeAreaView>
</>
);
} }
const styles = StyleSheet.create({ scrollView: { backgroundColor: '#f9f9f9', padding: 10, }, });
export default App;
To Change X and Y axis value use object to store the data:
import React from 'react'; import {SafeAreaView, StyleSheet, ScrollView, View} from 'react-native'; import {BarChart, Grid, XAxis, YAxis} from 'react-native-svg-charts'; import * as scale from 'd3-scale'; import {Text} from 'react-native-svg'; class App extends React.Component { render() { const data = [ { value: 50, labelX: 'A', labelY: 'One', }, { value: 10, labelX: 'B', labelY: 'Two', }, { value: 40, labelX: 'C', labelY: 'Three', }, { value: 95, labelX: 'D', labelY: 'Four', }, { value: 85, labelX: 'E', labelY: 'Five', }, ];
const CUT_OFF = 20; const Labels = ({x, y, bandwidth, data}) => data.map((item, index) => ( <Text key={index} x={x(index) + bandwidth / 2} y={item.value < CUT_OFF ? y(item.value) - 10 : y(item.value) + 15} fontSize={14} fill={item.value >= CUT_OFF ? 'white' : 'black'} alignmentBaseline={'middle'} textAnchor={'middle'}> {item.value} </Text> )); return ( <> <SafeAreaView> <ScrollView contentInsetAdjustmentBehavior="automatic" style={styles.scrollView}> <View style={{flexDirection: 'row', height: 200}}> <View style={{width: 50, flexDirection: 'column'}}> <YAxis data={data} scale={scale.scaleBand} svg={{ fill: 'grey', fontSize: 20, }} style={{flex: 1}} min={0} yAccessor={({index}) => index} formatLabel={(_, index) => data[index].labelY} /> <View style={{flex: 0.1}} /> </View> <View style={{flex: 1, flexDirection: 'column'}}> <BarChart style={{flex: 1}} data={data} gridMin={0} yAccessor={({item}) => item.value} svg={{fill: 'rgb(134, 65, 244)'}}> <Labels /> <Grid direction={Grid.Direction.HORIZONTAL} /> </BarChart> <XAxis data={data} scale={scale.scaleBand} svg={{ fill: 'grey', fontSize: 20, }} min={0} style={{flex: 0.1}} yAccessor={({index}) => index} formatLabel={(_, index) => data[index].labelX} /> </View> </View> </ScrollView> </SafeAreaView> </> );
} }
const styles = StyleSheet.create({ scrollView: { backgroundColor: '#f9f9f9', padding: 10, }, });
export default App;
TEXT IS CREATING ISSUE WITH NORMAL TEXT FROM REACT-NATIVE
can this is use for area chart also ????
What is the problem? Hi, i am using this module it's working fine. But my question is how to change the x-axis values and y-axis values in Bar-Chart. Initially takes x-axis values are
0,1,2,3,4------
Initially takes y-axis values are-80,-60,-40,-20,0,20,40-----
What platform?
Code to reproduce
Here is my screenshot:-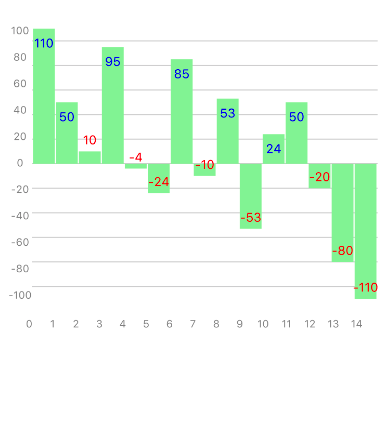