Open bmwertman opened 5 years ago
Hi, i had the same issue too. OnChangeDate first setDate with empty array ([]) then set your data with time out. It fixed my problem.
const onSwitchChange = (index) => {
setData([]);
setTimeout(() => {
setData([50, 10, 40, 95, 85, 95, 35, 53, 24, 50])
}, 100)
}
What is the problem?
I have an array of objects with 3 properties on each; time, salinity and volume.
When I switch from rendering time and salinity to rendering time and volume, or vice versa, the labels on the Y-axis do not change as expected. The new Y-axis labels get bunched up together on top of each other. The old Y-axis labels from the initial render remain on the chart
Charted data and X-axis(time) change as expected.
This sounds very similar to this issue, Labels not re-rendering correctly when data changes #135
When does it happen?
Toggle the component's
dataView
state, which is used in both theyAccessor
andformatLabel
functions, from 'salinity' to 'volume' or from 'volume' to 'salinity'What platform?
Versions
React Native version: 0.58.6 react-native-svg: 9.3.3 react-native-svg-charts-version: 5.2.0 and JesperLekland/react-native-svg-charts#c9f5c39c35230c75dcd040f2ace80b6362a80470
Code to reproduce
Screenshots
Initial Render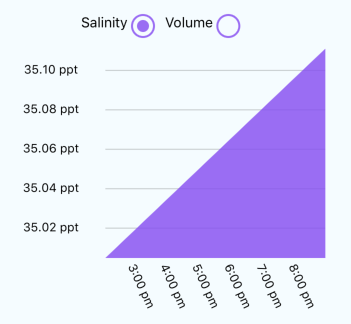
Rerender after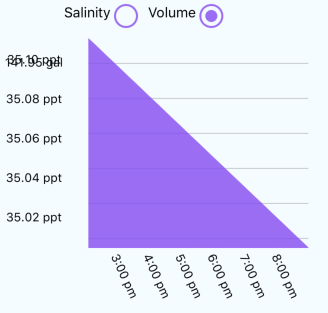
dataView
state's value is changed