Open ssfrr opened 5 years ago
update - rewriting the broadcast in terms of map
seems to work:
function err(D)
errs = map(X1, X2, ω) do x1, x2, om
abs2(x2 - x1 * exp(-im*om*D))
end
sum(errs)
end
So it seems the problem is with the broadcasting
(Oops, wrong button sorry.)
It works for me:
julia> err(D) = sum(@.(abs2(X2 - X1 * exp(-im*ω*D))))
err (generic function with 1 method)
julia> @time minimise(err, -5..5)
0.034151 seconds (3.65 k allocations: 1.380 MiB)
([5366.36, 5405.64], Interval{Float64}[[0.679316, 0.679927], [0.679926, 0.680537], [0.678715, 0.679317], [0.680536, 0.681157], [0.678105, 0.678716], [0.677504, 0.678106], [0.681156, 0.681748], [0.681747, 0.682349], [0.676904, 0.677505], [0.682348, 0.682949], [0.676313, 0.676905], [0.682948, 0.683559], [0.675702, 0.676314], [0.683558, 0.68416], [0.675102, 0.675703]])
What version of IntervalArithmetic.jl
et al are you using?
I just re-tried this with IntervalOptimisation#master and IntervalArithmetic#master and got the same error. I tried on both Julia 1.1 and Julia 1.2.
Also, there's a much more minimal MWE:
using IntervalArithmetic
randn(10) .+ (-2..2)
So this is actually an IntervalArithmetic problem, not IntervalOptimization. I can move the issue over there.
Oh, actually this is a dup of https://github.com/JuliaIntervals/IntervalArithmetic.jl/issues/255
Closing since needs to be fixed in IntervalArithmetic.jl
.
Reopening since it's not fixed yet...
I'm having some trouble minimizing a function that includes complex numbers and broadcasting. I'm not sure which is causing the problem. On slack @dpsanders mentioned being a little concerned by complex numbers, but looking at the stack trace I'm actually wondering if its a broadcasting problem (it seems to be trying to assign an interval into the result array from a broadcasting operation, and for some reason the result array has float elements.
I'm coming from a DSP background, and the application is delay estimation between two signals where the delay is not an integer number of samples. The main insight here is that a delay in the time domain is the same as multiplying by a complex exponential in the frequency domain. The frequency of the exponential determines the delay, so we can estimate the delay by finding the frequency that minimizes the distance between the two spectra. Here's a MWE:
Which gives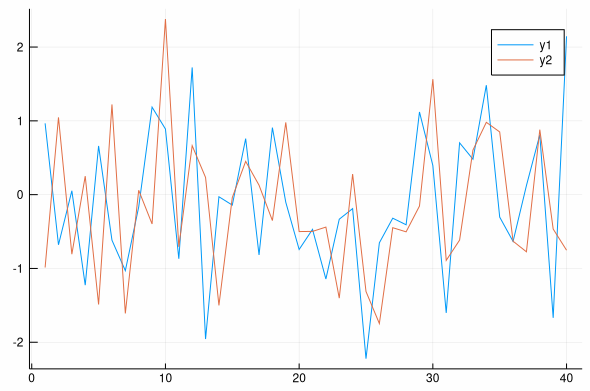
We're trying to estimate
D
. Here's our error function (which we see has a minimum at 0.68):Trying to minimise it gives: