Open jmeyers91 opened 4 years ago
I have the same problem tell me if you found a solution !
I also got into similar issue for couple of minutes. But later figured out that i am only sending wrong value of selected array. Make sure you are sending values with false etc in it. It select Select All option based on following:
length of option === length of selected item
I've got a form with several multi-select components on it and the options for some of them are dependent on which options are selected in others. The problem I'm running into is when I check and uncheck options in the select that is depended on, the other select seems to end up in a state where some but not all options are selected, but it shows all options as selected.
Here's a screenshot of what I mean: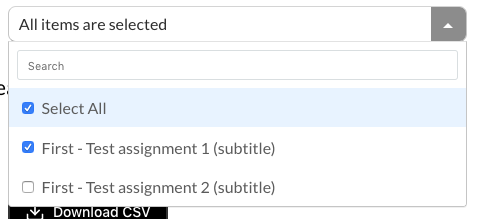
And the code for the form: