Closed abhignay closed 1 year ago
Unplug the 1 watt units from your teensy immediately. These units can easily consume more power that the onboard regulator can supply. I've burned up some NANO's forgetting about power consumptions. I'm surprised the regulator survived this long.
These units really don't like 5v0 power supply. 3v3 is their recommend input voltage. I hope the voltage / heat issue did not damage the EBYTES
Do you have the antennas connected? The settings look like they match on both units. These should send/receive.
Hi Kris, what do you recommend I power the Ebytes with, should I get another one just to be safe?
I power all of mine with DC to DC buck converters there’s a wide variety of them on Amazon some have fixed output voltages some are variable. I’ve used several different types just as long as you’re out putting 3.3 V you should be good and at least one amp. Hard to see if your unit is fried. Do you have antennas installed?
Yep, I have antennas installed, What else could be causing the problems? I believe the current and voltage requirements are being fulfilled with my setup
Can you indicate exactly what you’re wearing connections are?
I seriously doubt it will matter between a 3.2 and a 4.0 since they’re both 32 bit but each my pack that struct differently Try sending just one bite
I’ll knock this up sometime this weekend and see if there are differences between how three point twos and 4.0’s interact
The connectors are SMA, here's a picture of them hooked up to the antenna.
I have a spare Teensy 3.2, I'll try using two 3.2's if I'm still not receiving data packets would it be safe to assume that one of my Ebyte's are fried?
Hi Kris. Looks like it's the same result with 2 Teensy 3.2's
Should I look into buying another Ebyte? On another note would it be OK to power said Ebyte (transmitter) with a 1s 3.6V LiPo?
I think I see what this issue is... I tried your exact code and mine did not work either. I noticed your receiver code does not have the Transceiver.SaveParameters(PERMANENT); Call
I just tried the following (with the Transceiver.SaveParameters(PERMANENT); call)
Teensy LC -> Teensy 4.0 EBYTE E51-TTL-500 (really should not matter).
Mine works, transmits and receives..
Try this...
in your receiver code just after //save the parameters to the unit,
add the line
Transceiver.SaveParameters(PERMANENT);
This should get things working.
hmm, seems like that didn't fix it, tried it with both these configurations:-
Teensy 3.2 -> Teensy 3.2 Teensy 4.0 -> Teensy 3.2
One thing I did do is uncomment the defines for the 1W modules in the header file, that didn't help either
You only need the library to program these units, let's skip the library completely and just use the serial port.
If this does not work, i'd say your units are defective.
// transmitter
//note serial1 is pin 0 and 1 in the MCU, EBYTE Rx connects to pin 1, EBYTE Tx connects to pin 0 // you could try Serial2 or Serial3 Teensy 4.0 pin 7, 8 are Serial2, Teensy 3.2 pin 7, 8 are Serial3
// create the transceiver object, passing in the serial and pins EBYTE Transceiver(&ESerial, PIN_M0, PIN_M1, PIN_AX);
void setup() { pinMode(PIN_MO, OUTPUT); pinModePIN_M1 OUTPUT);
digitalWrite(PIN_MO, LOW); digitalWrite(PIN_M1, LOW);
Serial.begin(9600);
while (!Serial) {}
ESerial.begin(9600);
Serial.println("Starting Sender");
}
void loop() {
ESerial.print(9);
Serial.print("Sending");
delay(1000);
}
//////////////////////////////////////// // receiver
//note serial1 is pin 0 and 1 in the MCU, EBYTE Rx connects to pin 1, EBYTE Tx connects to pin 0 // you could try Serial2 or Serial3 Teensy 4.0 pin 7, 8 are Serial2, Teensy 3.2 pin 7, 8 are Serial3
void setup() { pinMode(PIN_MO, OUTPUT); pinModePIN_M1 OUTPUT);
digitalWrite(PIN_MO, LOW); digitalWrite(PIN_M1, LOW); Serial.begin(9600);
while (!Serial) {}
ESerial.begin(9600);
Serial.println("Starting Reader");
}
void loop() {
if ESerial.available()) {
Serial.println(ESerial.read();
} else { // if the time checker is over some prescribed amount // let the user know there is no incoming data if ((millis() - Last) > 1000) { Serial.println("Searching: "); Last = millis(); }
}
}
Looks like the my transmitter is defective, also would it be ok to power said Ebyte (transmitter) with a 1s 3.6V LiPo?
I would check the data sheet for an acceptable range of supply voltages
one last thing, put a delay(10) in the receiver loop just before the available() call.
I noticed my Teensy 4.0 sometimes can't ready the serial port from my GPS sensor,
Alright Kris! I'll try that, would you mind if I close this issue once I get the new Ebyte (should be in 2 days) and test it?
Hi Kris, just wanted to end this issue with this comment. I managed to get everything working with a new Ebyte! Thanks for all your help and for making such an awesome library :)
edit - sorry about this, I didn't notice that you had already closed the issue
That is great news. I find these units very reliable even if loss of power, they start right up and continue working. I have these in a home automation setting (remote garage door and outside temp monitoring) and have been working for about 2 years with no issues.
If you find yourself wanting to communicate between 2 different MCU (a Teensy and NANO), the structure will probably pack differently and become garbled up on the receive, __attribute (packed)___ may help, or use the EasyTransfer library.
Ok Kris, will keep that in mind!
Hi Kris, one last thing, the datasheet recommends using this module with 5V, would it be ok to power it with 5V @ 3A (using an AP63300 to stepdown 7.4V to 5V, 7.4V comes from a 2s Li-Po).
If that’s what the data sheet says… I’ve always powered mine with 3.3 they seem to get warm at 5 V. However before you connect your RX and TX lines I would measure the output voltage and make sure there’s still 3.3 if you power from 5 volts TEensy 4 are not 5 V tolerant. I’m not sure about that specific buck converter but that’s all I use.
Thanks. Kris Kasprzak
On Oct 12, 2022, at 11:53 AM, moonman @.***> wrote:
Hi Kris, one last thing, the datasheet recommends using this module with 5V, would it be ok to power it with 5V @ 3A (using an AP63300 to stepdown 7.4V to 5V, 7.4V comes from a 2s Li-Po).
— Reply to this email directly, view it on GitHub, or unsubscribe. You are receiving this because you modified the open/close state.
Hi Kris, firstly thanks for making this library! I'm having some problems with receiving data packets.
I'm using the E32 433T30D 1W module and a Teensy 4.0 with the transmitter and Teensy 3.2 with the receiver. I tried powering the transmitter E32 with a Panasonic NCR18650PF 3c 2900mAh Li-Ion battery, but the E32 was got extremely hot, so I'm currently powering both with the onboard teensy vregs.
This is the Transmitter code:-
This is the receiver's code:
Here's all the transmitter's parameters: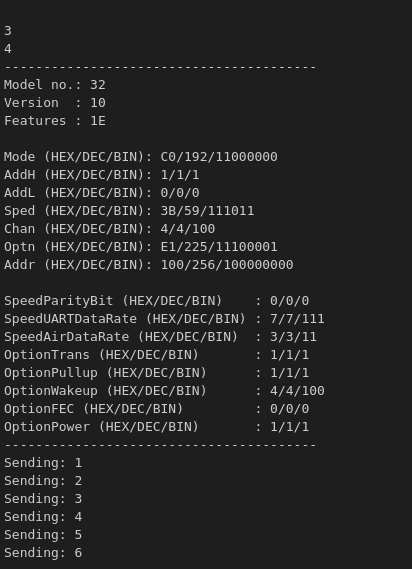
The receiver's parameters: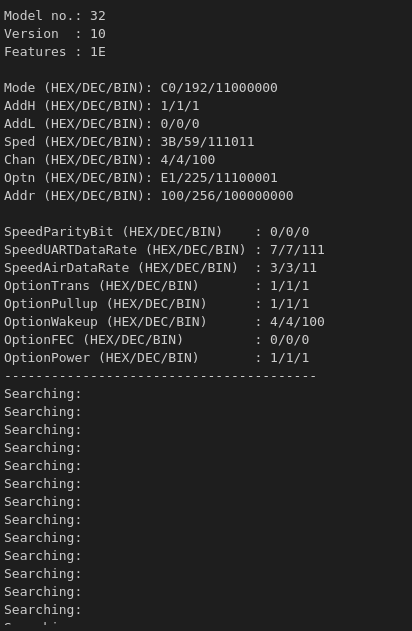
As you can see in the screenshots above, the transmitter has successfully sent data packets yet the receiver is still searching. Could you help me with this?
Thanks, moonman