Closed tilmantroester closed 3 years ago
@tilmantroester in this case the mask (and the fields) you're using are complete non-band-limited (i.e. the power spectrum of the mask is constant at high ell), so the fact that namaster only computes mode-coupling up to a given ell_max coupled with the numerical errors of healpix means that the resulting MCM is probably inaccurate.
Maybe try using a nicer mask (e.g. a smoothed version of this one)?
@damonge is correct here that the mask is pathological, and reducing the power at small scales will make mode-coupling accurate again. This is the usual signal processing situation: input signals which are not bandlimited suffer from aliasing, so you have to apply a low-pass filter.
Here's a row of the mode-coupling matrix comparing a non-band-limited mask, and a nice smoothed mask, plotted in log-log. This describes the contributions to the pseudo-spectrum at ell=200 from the underlying spectrum. The mode-coupling matrix elements for the white noise mask are increasing with ell, because the power spectrum of the mask is constant.
Simply scaling the harmonic coefficients of the mask by sqrt(ell) past some high multipole is sufficient for me to recover unbiased spectra again.
The issue persists when using a real weight map. It's a shear weight map, so it's not clear to me how that can be smoothed while conserving the shear weight information appropriately. Not using nmt_workspace.couple_cell
but instead scaling the theoretical noise spectrum by the mean of the square of the weight map solved the problem, however:
This can be closed I think. A heuristic that checks if a mask is problematic and warns the user might be useful though.
Yes, this is due to the finite ell_max up to which the MCM is computed, whereas the input power spectrum has power up to ell=infinity in principle. Multiplying by <mask^2> here is the exact effect of a mask on a flat power spectrum, so that's bound to do better (this is incidentally what we did in https://arxiv.org/abs/2010.09717).
In practice we have never seen this be a problem for shear-like signal power spectra, where the signal decreases with scale relatively fast, but this is something we may need to test again for LSST when the error bars are smaller.
I'll close this for now then, but please feel free to reopen in any related issues appear.
I have an issue where namaster seems to fail to return unbiased estimates of the power spectrum if a weight map is being used as the mask. I don't know if that's a fundamental issue (doubtful, since support for weight maps is stated in a couple of places) or I messed up somewhere (entirely possible but the same code works for binary masks). Apodisation doesn't seem to help.
The setup is as follows (full code at the end):
nmt_workspace.decouple_cell(nmt_workspace.couple_cell(Cl_theory))
For full-sky (top panel) and binary mask (bottom panel, just the circular footprint), this works as expected: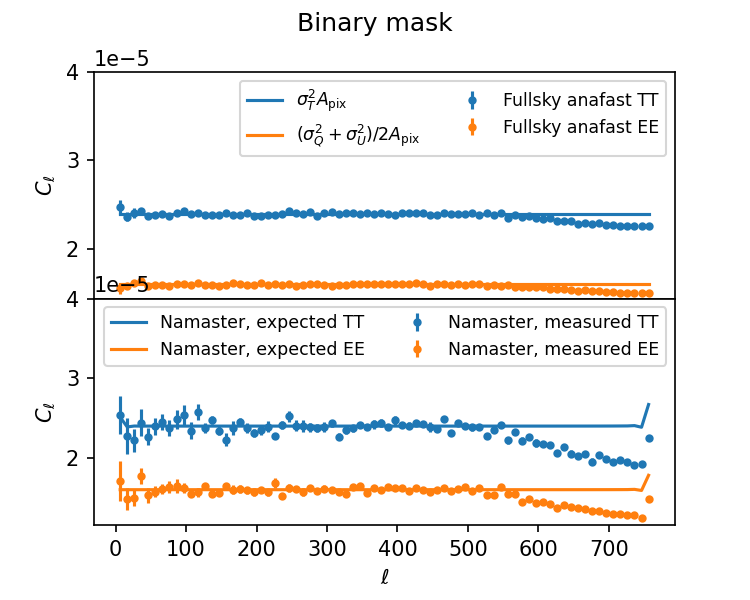
When using the weight map, the measured, decoupled Cls don't agree with the analytic values anymore: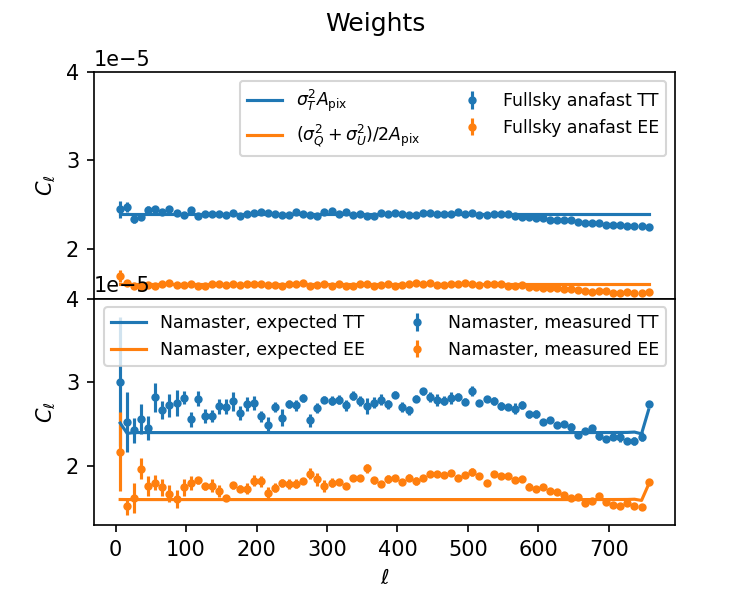
Code: