Open tommedema opened 7 years ago
Hi! It would be interesting to discover why it didn't worked on elm and Chrizmas. I think it may be a timing problem, but i'm not sure.
Did you tried navigating them without headless mode on to see what happens?
Cheers! Lucho
Hi! Any news about this?
Thanks! Luciano
@LucianoGanga yes, I tried that, and it is indeed a timing issue. But the question is why await mainTab.waitForPageToLoad();
does not seem to resolve this timing issue. What do you think?
Hi @tommedema !
It may be because of how waitForPageToLoad() works. It waits for the browser event of "page loaded", and in the case that is failing, maybe the page tells the browser that it already loaded all but do some stuff asynchronously. What I think is that you can try using the .waitForSelectorToLoad()
and wait for a specific selector you need to be loaded.
Thanks! Lucho
First let me say that I very much appreciate your work. Am planning to contribute in the future, perhaps this is a good start. I could write a test for
waitForPageToLoad
with different single page applications that load data from a backend.Before writing the test I would like to actually understand how I can make this work. So far it seems to work for react+redux, react+mobx, angular1, and angular4. However, it does not seem to work with elm and a CMS called Chrizmas.
I've been trying this out as follows:
Results are promising but not perfect.
react-redux: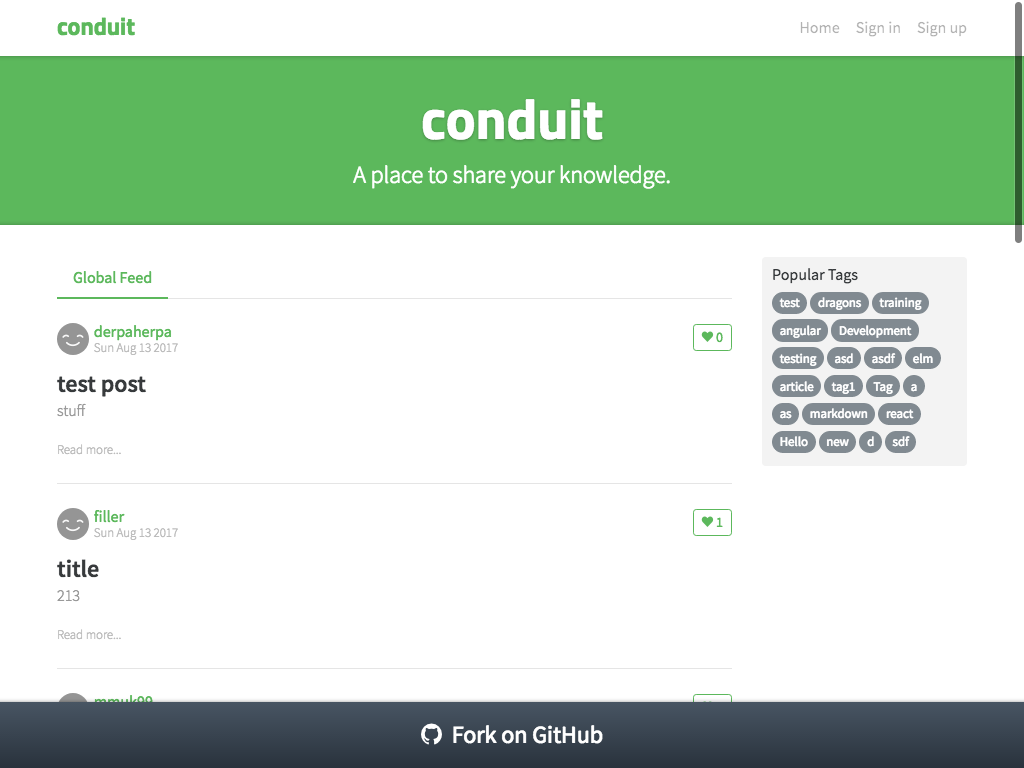
react-mobx: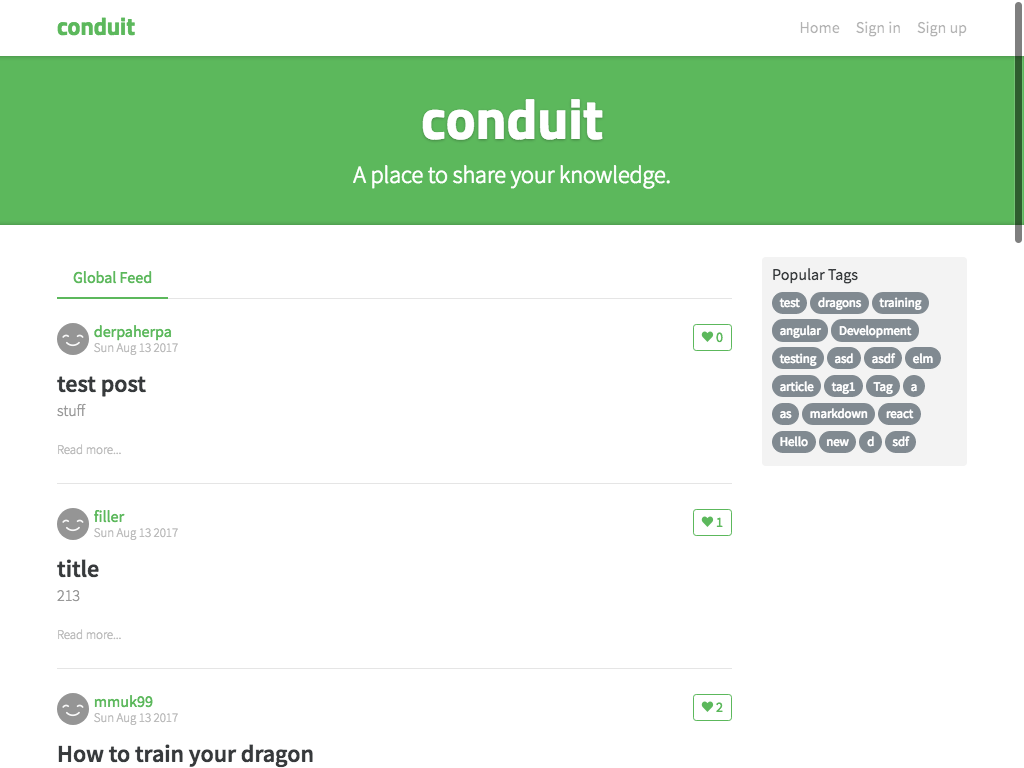
angular1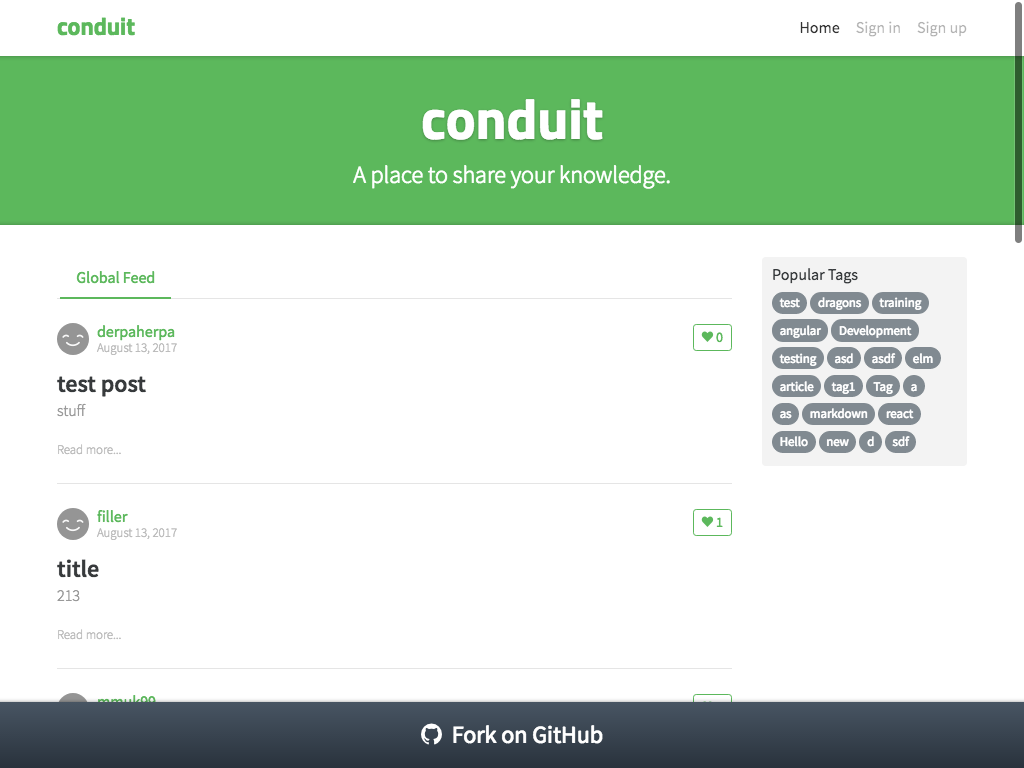
angular4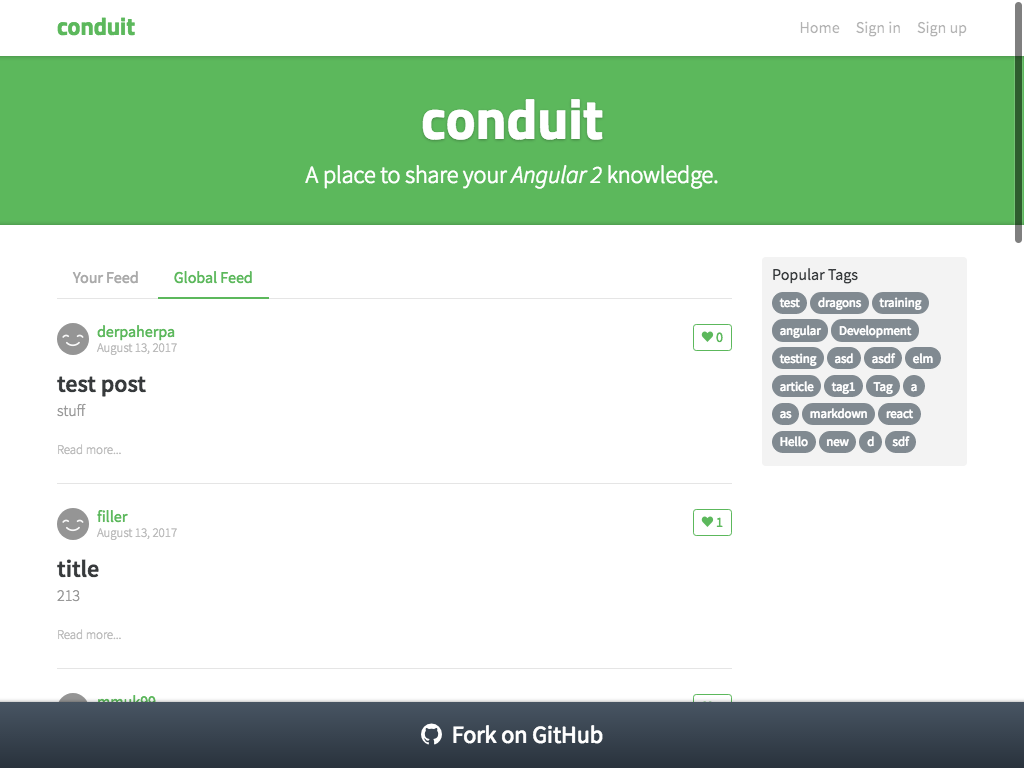
elm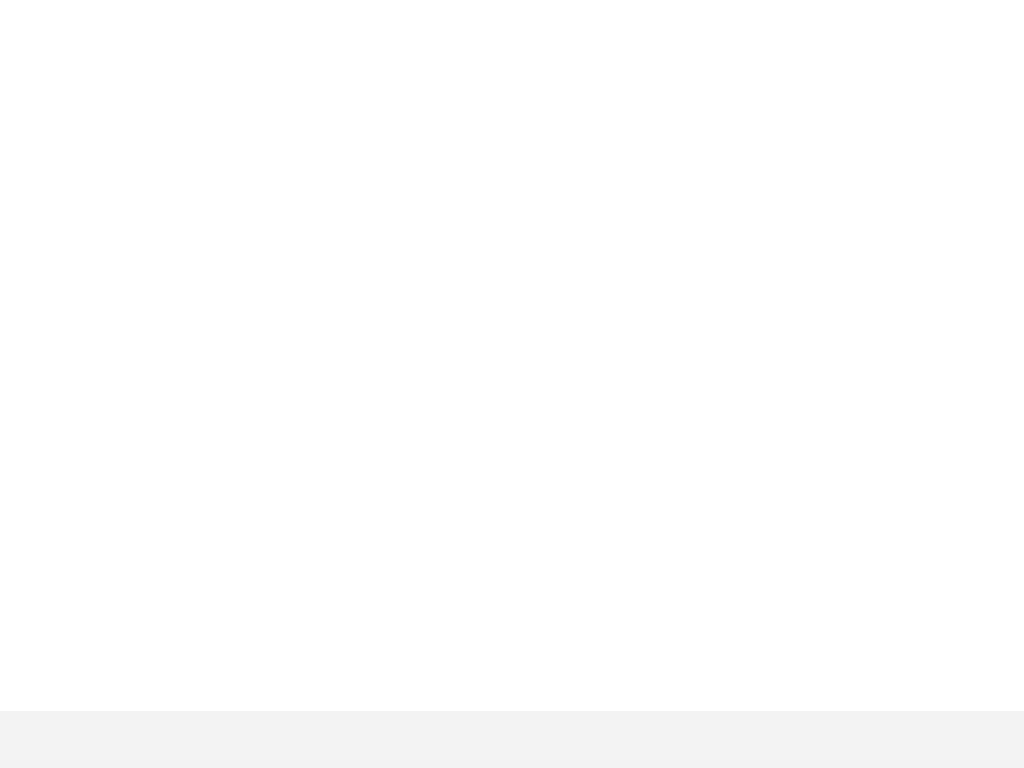
chrizmas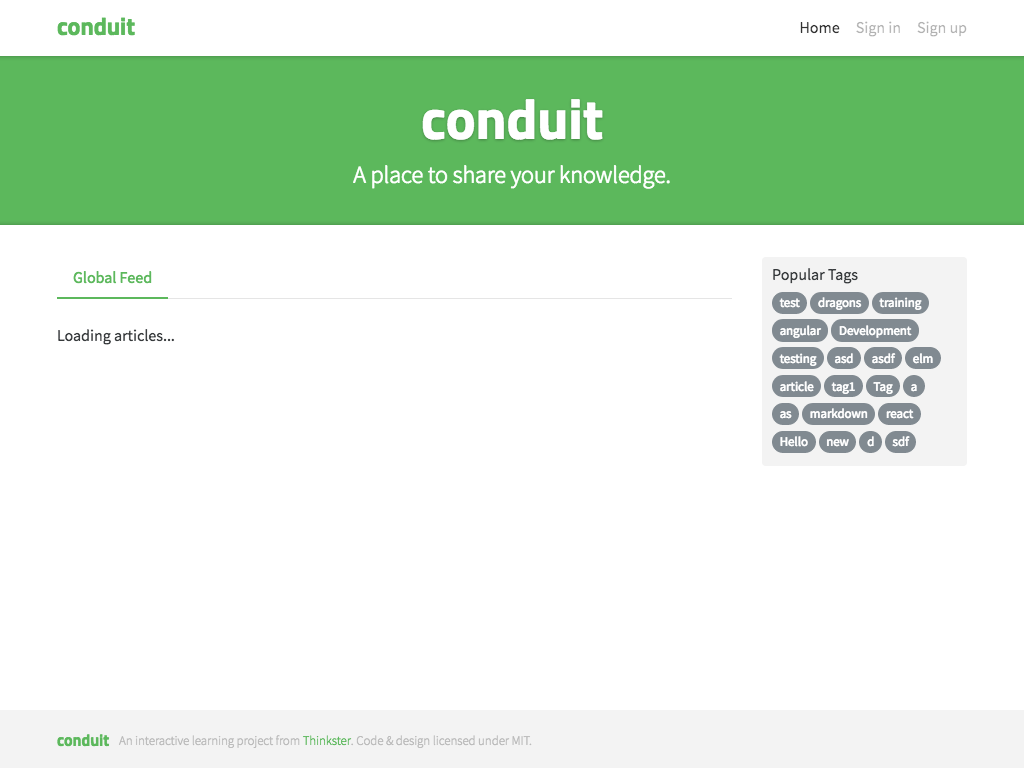
The URLs come from the realworld project. My plan is to make these work first, then write a test case for it. If you have other SPA example urls, that would be great.
Also, are you ok with tests (integration tests I guess) that depend on these realworld.io projects and backends? They will probably stay alive but this is not guaranteed.