Open benjinne opened 1 year ago
Here's a fully working example from this branch:
#include "Arduino.h"
#include <SPI.h>
#include <SPIMemory.h>
#define MISO PB4
#define MOSI PB5
#define SCK PB3
#define CS PB0
SPIClass spiclass(MOSI, MISO, SCK);
SPIFlash flash(CS, &spiclass);
void setup() {
SerialUSB.begin(115200);
flash.setClock(20000000);
flash.begin(MB(2));
}
void loop() {
uint16_t manID = flash.getJEDECID();
SerialUSB.printf("manID %i \n\r", manID);
flash.eraseSector(0x03);
if(flash.writeByte(0x03, 20)){
SerialUSB.printf("write success!\n\r");
}
else
SerialUSB.printf("Error.\n\r");
uint8_t abyte = flash.readByte(0x03);
SerialUSB.printf("abyte: %i\n\r", abyte);
delay(2000);
}
output is:
manID 16405
write success!
abyte: 20
chip included on the dev board is: https://stm32-base.org/assets/pdf/devices/W25Q16JV.pdf
in my arduino IDE (SPIFlash.h) can't work. and can't compile. I added comment on SPIFlash.h file
//#if defined (ARDUINO_ARCH_SAMD) || defined(ARCH_STM32) || defined(ARDUINO_ARCH_ESP32)
// SPIFlash(uint8_t cs = CS, SPIClass spiinterface=&SPI);
// #elif defined (BOARD_RTL8195A)
// SPIFlash(PinName cs = CS);
// #else
// SPIFlash(uint8_t cs = CS);
// SPIFlash(int8_t SPIPinsArray);
// #endif
and add
SPIFlash(uint8_t cs = CS, SPIClass *spiinterface=&SPI);
and work, the skecth can upload to board.
Pull request details
What kind of change does this PR introduce? (Bug fix, feature, docs update, ...)
[ x] Bug fix
[ x] Added feature
[ x] Documentation update
What is the current behavior? Using this development board (W25Q16JV included): https://stm32-base.org/boards/STM32F407VET6-STM32-F4VE-V2.0.html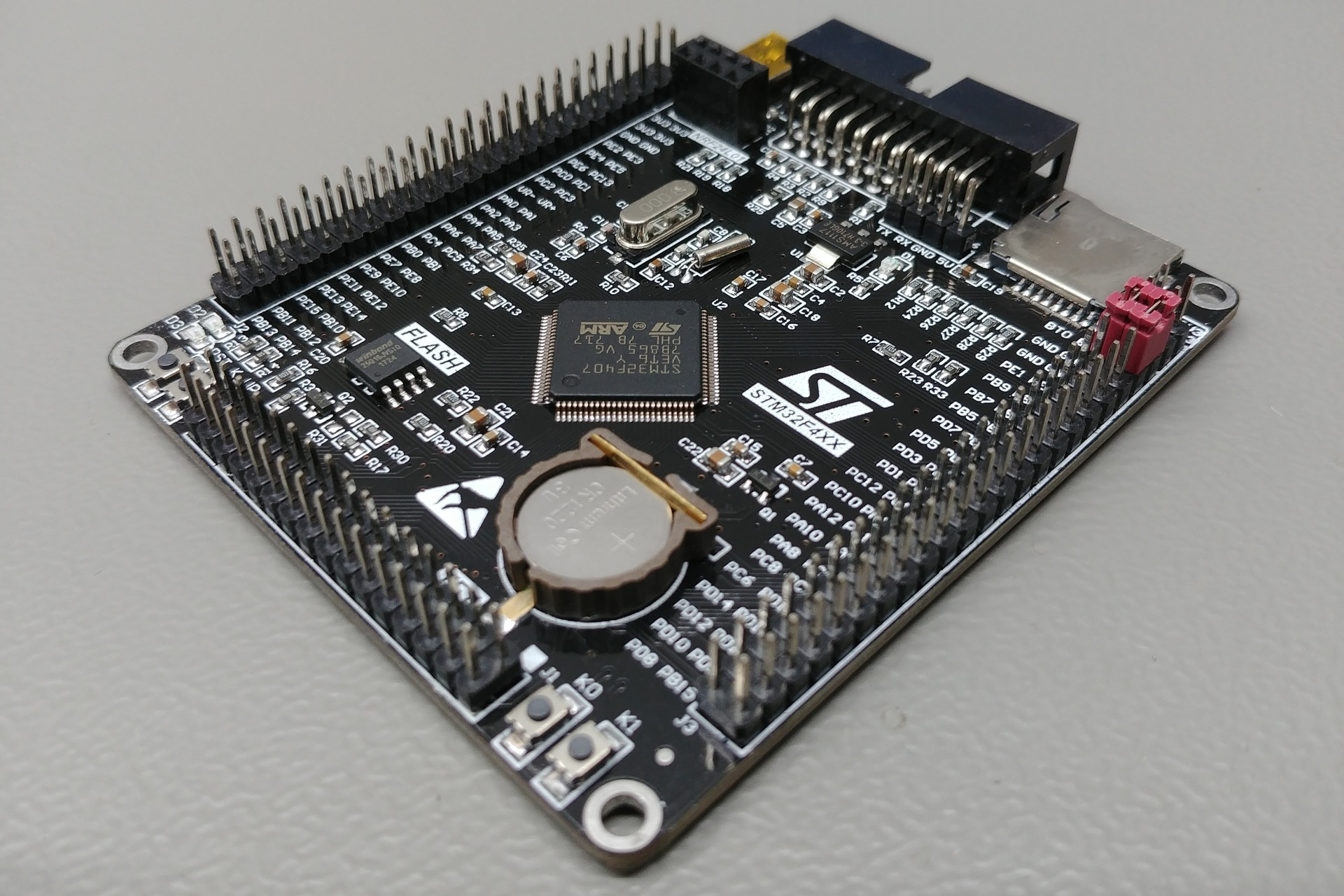
The stm32 defines needed updated.
This example code wouldn't compile:
Due to SPIFlash.h being included before AARCH_STM32 was defined the code wouldn't compile:
Also since this example is using a non-standard SPIClass, more ARCH_STM32 were needed so that the SPIFlash _spi object would be used instead of the generic SPI object
What is the new behavior? (if this is a feature change) Adds support for more STM32 boards like the one mentioned above.
Other information:
DO NOT DELETE OR EDIT anything below this
Note 1: Make sure to add all the information needed to understand the bug so that someone can help. If any essential information is missing we'll add the 'Needs more information' label and close the issue until there is enough information.
Note 2: For support questions (for example, tutorials on how to use the library), please use the Arduino Forums. This repository's issues are reserved for feature requests and bug reports.