Describe the bug
I first downsample a point cloud with RGB feature, and then use ME.MinkowskiInterpolation to upsample the point cloud back to the original resolution. However, the upsampled RGB is obviously wrong in some regions.
import open3d as o3d
import MinkowskiEngine as ME
from MinkowskiEngine.MinkowskiPooling import MinkowskiAvgPooling
import numpy as np
import torch
def load_file(file_name):
pcd = o3d.io.read_point_cloud(file_name)
coords = np.array(pcd.points)
colors = np.array(pcd.colors)
return coords, colors, pcd
# load point cloud
coords, colors, _ = load_file('./scene0000_00_vh_clean_2.ply')
coords_qv, unique_map, inverse_map = ME.utils.sparse_quantize(
coordinates=coords,
quantization_size=0.05,
return_index=True,
return_inverse=True)
feats_qv = torch.from_numpy(colors[unique_map])
# We first downsample the original data
pooling = MinkowskiAvgPooling(kernel_size=2, stride=2, dimension=3)
ori_data = ME.SparseTensor(
coordinates=ME.utils.batched_coordinates([coords_qv]),
features=feats_qv.float()
)
downsampled_data = pooling(ori_data)
# Save for visualization
downsampled_pcd = o3d.geometry.PointCloud()
downsampled_pcd.points = o3d.utility.Vector3dVector(downsampled_data.C[:,1:].numpy())
downsampled_pcd.colors = o3d.utility.Vector3dVector(downsampled_data.F.numpy())
o3d.io.write_point_cloud("./downsampled_pcd.ply", downsampled_pcd)
# Then we upsample the downsampled_data
upInt = ME.MinkowskiInterpolation()
upsampled_data = upInt(downsampled_data, ME.utils.batched_coordinates([coords_qv]).float())
# Save for visualization
upsampled_pcd = o3d.geometry.PointCloud()
upsampled_pcd.points = o3d.utility.Vector3dVector(coords_qv.numpy())
upsampled_pcd.colors = o3d.utility.Vector3dVector(upsampled_data.numpy())
o3d.io.write_point_cloud("./upsampled_pcd.ply", upsampled_pcd)
Results:
Expected behavior
It is expected that the upsampled_pcd.ply should have similar color with downsampled_pcd.ply. However, it is obvious that some regions of upsampled_pcd.ply have strange color.
Desktop (please complete the following information):
OS: CentOS Linux release 7.9.2009 (Core)
Python version: 3.8.13
Pytorch version: 1.7.1
CUDA version: 11.1
NVIDIA Driver version: 515.86.01
Minkowski Engine version: 0.5.4
Output of the following command. (If you installed the latest MinkowskiEngine, paste the output of python -c "import MinkowskiEngine as ME; ME.print_diagnostics()".
==========System==========
Linux-3.10.0-1160.80.1.el7.x86_64-x86_64-with-glibc2.17
cat: /etc/lsb-release: No such file or directory
3.8.13 (default, Mar 28 2022, 11:38:47)
[GCC 7.5.0]
==========Pytorch==========
1.7.1
torch.cuda.is_available(): True
==========NVIDIA-SMI==========
/usr/bin/nvidia-smi
Driver Version 515.86.01
CUDA Version 11.7
VBIOS Version 90.02.42.00.0F
Image Version G001.0000.02.04
GSP Firmware Version N/A
==========NVCC==========
/cluster/apps/gcc-6.3.0/cuda-11.1.1-s2fmzfqahrfvezvmg4tslqqedhl3bggv/bin/nvcc
nvcc: NVIDIA (R) Cuda compiler driver
Copyright (c) 2005-2020 NVIDIA Corporation
Built on Mon_Oct_12_20:09:46_PDT_2020
Cuda compilation tools, release 11.1, V11.1.105
Build cuda_11.1.TC455_06.29190527_0
==========CC==========
CC=/cluster/spack/apps/linux-centos7-x86_64/gcc-4.8.5/gcc-6.3.0-sqhtfh32p5gerbkvi5hih7cfvcpmewvj/bin/g++
/cluster/spack/apps/linux-centos7-x86_64/gcc-4.8.5/gcc-6.3.0-sqhtfh32p5gerbkvi5hih7cfvcpmewvj/bin/g++
g++ (GCC) 6.3.0
Copyright (C) 2016 Free Software Foundation, Inc.
This is free software; see the source for copying conditions. There is NO
warranty; not even for MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
==========MinkowskiEngine==========
0.5.4
MinkowskiEngine compiled with CUDA Support: True
NVCC version MinkowskiEngine is compiled: 11010
CUDART version MinkowskiEngine is compiled: 11010
Additional context
Add any other context about the problem here.
Describe the bug I first downsample a point cloud with RGB feature, and then use ME.MinkowskiInterpolation to upsample the point cloud back to the original resolution. However, the upsampled RGB is obviously wrong in some regions.
To Reproduce
The used sample data can be downloaded here: https://drive.google.com/file/d/1PGXXxtfUs0Ji8hKE-jnb7LbsUCTMgm2C/view?usp=sharing
Results: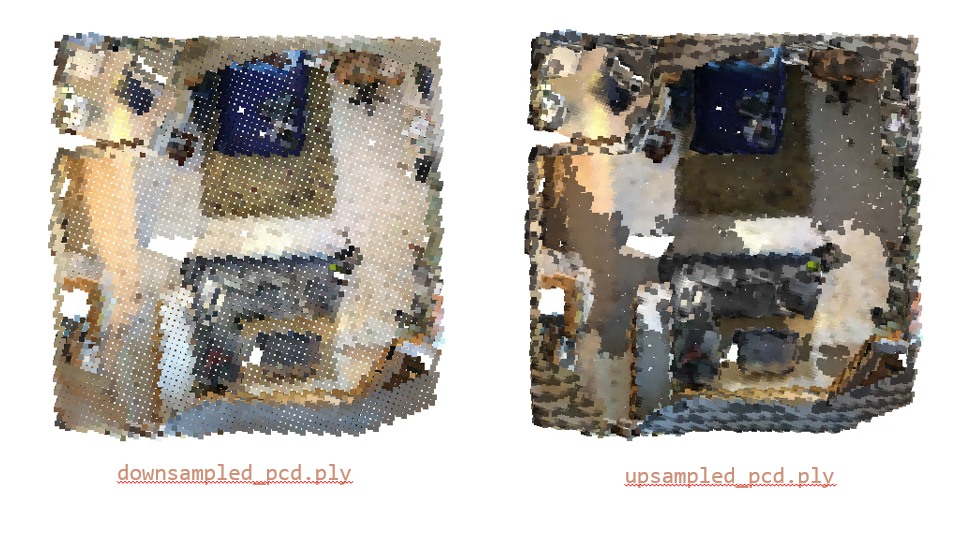
Expected behavior
It is expected that the upsampled_pcd.ply should have similar color with downsampled_pcd.ply. However, it is obvious that some regions of upsampled_pcd.ply have strange color.
Desktop (please complete the following information):
python -c "import MinkowskiEngine as ME; ME.print_diagnostics()"
.==========System========== Linux-3.10.0-1160.80.1.el7.x86_64-x86_64-with-glibc2.17 cat: /etc/lsb-release: No such file or directory 3.8.13 (default, Mar 28 2022, 11:38:47) [GCC 7.5.0] ==========Pytorch========== 1.7.1 torch.cuda.is_available(): True ==========NVIDIA-SMI========== /usr/bin/nvidia-smi Driver Version 515.86.01 CUDA Version 11.7 VBIOS Version 90.02.42.00.0F Image Version G001.0000.02.04 GSP Firmware Version N/A ==========NVCC========== /cluster/apps/gcc-6.3.0/cuda-11.1.1-s2fmzfqahrfvezvmg4tslqqedhl3bggv/bin/nvcc nvcc: NVIDIA (R) Cuda compiler driver Copyright (c) 2005-2020 NVIDIA Corporation Built on Mon_Oct_12_20:09:46_PDT_2020 Cuda compilation tools, release 11.1, V11.1.105 Build cuda_11.1.TC455_06.29190527_0 ==========CC========== CC=/cluster/spack/apps/linux-centos7-x86_64/gcc-4.8.5/gcc-6.3.0-sqhtfh32p5gerbkvi5hih7cfvcpmewvj/bin/g++ /cluster/spack/apps/linux-centos7-x86_64/gcc-4.8.5/gcc-6.3.0-sqhtfh32p5gerbkvi5hih7cfvcpmewvj/bin/g++ g++ (GCC) 6.3.0 Copyright (C) 2016 Free Software Foundation, Inc. This is free software; see the source for copying conditions. There is NO warranty; not even for MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
==========MinkowskiEngine========== 0.5.4 MinkowskiEngine compiled with CUDA Support: True NVCC version MinkowskiEngine is compiled: 11010 CUDART version MinkowskiEngine is compiled: 11010
Additional context Add any other context about the problem here.