Closed ghost1372 closed 3 years ago
Did it happen due to lack of file versioning protection as described in #19 (did it fail to load becuase json was the older format?)
@Nucs No I created a example project please take a look at it By clicking on the button, the size of the columns is displayed correctly But the same sizes are stored differently in the settings file WpfApp3.zip
The idea you were trying to achieve is using a settings file as a view model or partially as a view model.
So I've decided to implement support for INotifyPropertyChanged
and INotifyCollectionChanged
.
Please have a look at this self explanatory example: https://github.com/Nucs/JsonSettings/blob/master/examples/INotifyPropertyChangedExample.cs
Also added IgnoreAutosaveAttribute
So now your settings file should look:
public class Configs : NotifiyingJsonSettings {
public override string FileName { get; set; } = "config.json";
private ObservableCollection<double> _columnWidth = new ObservableCollection<double>();
private ObservableCollection<DataGridLength> _columnWidth2 = new ObservableCollection<DataGridLength>();
private ObservableCollection<string> _columnWidth3 = new ObservableCollection<string>();
public virtual ObservableCollection<double> ColumnWidth {
get => _columnWidth;
set {
if (Equals(value, _columnWidth)) return;
_columnWidth = value;
OnPropertyChanged();
}
}
public virtual ObservableCollection<string> ColumnWidth3 {
get => _columnWidth3;
set {
if (Equals(value, _columnWidth3)) return;
_columnWidth3 = value;
OnPropertyChanged();
}
}
public virtual ObservableCollection<DataGridLength> ColumnWidth2 {
get => _columnWidth2;
set {
if (Equals(value, _columnWidth2)) return;
_columnWidth2 = value;
OnPropertyChanged();
}
}
}
This will trigger a save whenever the property (INotifyPropertyChanged) by calling OnPropertyChanged() from setter or one of the items inside the property (INotifyCollectionChanged) are changed.
Also only properties in the settings class are monitored, nested objects with INotifyPropertyChanged/INotifyCollectionChanged won't trigger save.
thank you i will test it when nuget package is ready
@Nucs i tested your sample code and column width is stored correctly but I see the 0s again. Is this normal?
I also noticed something strange If we run the example project I sent earlier with a change
private void Button_Click(object sender, RoutedEventArgs e)
{
Settings.MyProperty = "test";
}
All information is stored correctly in the database 🤔
but I see the 0s again. Is this normal?
I think Json.NET might be serializing the ObservableCollection
I also noticed something strange If we run the example project I sent earlier with a change
I think the binding for the column's widths is not working on the XAML level. So it triggers save only when change Settings.MyProperty. Could you drop .zip you ran? thanks.
Run the program Open the settings file in the text editor (vscode) Click on the button You can see the changes WpfApp3.zip
I initially assumed you would bind the column widths by templating the columns. Anyways, on the basic level - this is how it should be done without advanced binding. WpfApp3.zip
Key note, Collections have to be initialized empty, otherwise every load you'll end up with 5 more items (count: 5 in constructor). So you have to dynamically do it like in the example.
Arigato My problem was completely solved, I think this issue can be closed
I want to save the value of the datagrid column width in the setting file I used the following:
public Configs Settings = JsonSettings.Load<Configs>().EnableAutosave();
If I use the message box, I can see that all the columns have the correct value
MessageBox.Show(string.Join("; ", dataGrid.Columns.Select(column => column.ActualWidth)));
But the setting file shows the wrong information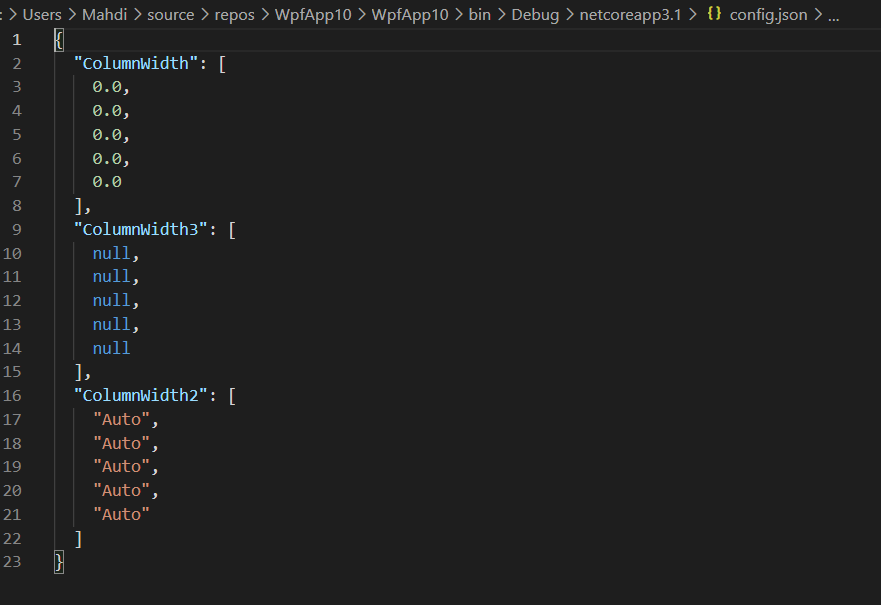