Open Pin-Jiun opened 1 year ago
STL是運用template進行開發的函式庫,C++11引入
template
STL包含三大部分 : Container Algorithm Iterator
Container
Algorithm
Iterator
vector, list, stack, queue, map,… 一種儲存資料的結構
find, sort, count,… 各種function的集合 基本容器都含有 begin(), end(), size(), max_size(), empty(), swap() 等用法
begin()
end()
size()
max_size()
empty()
swap()
C::iterator it
對於今天假設要使用sort,對於不同容器vector和list一定有不同的演算法 此時就又要分別對vector和list使用不同的演算法 假設有m跟演算法和n個容器,此時如果沒有Iterator則需要m*n個function 但今天有Iterator則只需要m+n個function
Vector 是C++標準程式庫中的一個類,可視為會自動擴展容量的陣列,以循序(Sequential)的方式維護變數集合
#include <iostream> #include <algorithm> #include <vector> using namespace std; int main() { //宣告vector v1, 其資料型別為int vector<int> v1; //指派元素 v1.push_back(3); v1.push_back(8); v1.push_back(2); v1.push_back(6); //宣告iterator vector<int>::iterator itrBegin = v1.begin(); vector<int>::iterator itrEnd = v1.end(); //algorithm利用iterator去操作function //可以使用 auto修飾字 //for(auto itr = itrBegin; itr != itrEnd; itr++) for(vector<int>::iterator itr = itrBegin; itr != itrEnd; itr++){ cout << itr << endl; //error 不可直接print iterator cout << *itr << endl; cout << &itr << endl; cout << &(*itr) << endl; } }
STL(standard template library)標準模板庫
STL是運用
template
進行開發的函式庫,C++11引入STL包含三大部分 :
Container
Algorithm
Iterator
Container (容器)
vector, list, stack, queue, map,… 一種儲存資料的結構
Algorithm (演算法)
find, sort, count,… 各種function的集合 基本容器都含有
begin()
,end()
,size()
,max_size()
,empty()
,swap()
等用法Iterator (抽象指標)
C::iterator it
的方式來宣告 iterator ,以取得容器內元素的位址對於今天假設要使用sort,對於不同容器vector和list一定有不同的演算法 此時就又要分別對vector和list使用不同的演算法 假設有m跟演算法和n個容器,此時如果沒有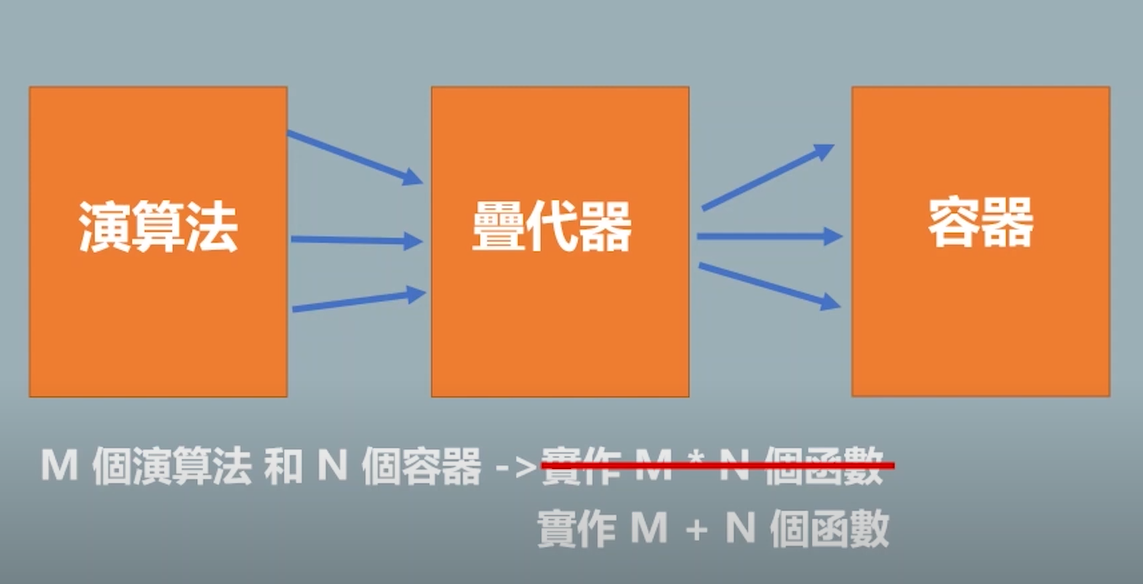
Iterator
則需要m*n個function 但今天有Iterator
則只需要m+n個functionSTL初探-Vector
Vector 是C++標準程式庫中的一個類,可視為會自動擴展容量的陣列,以循序(Sequential)的方式維護變數集合