S No. | Widget | Description
-- | -- | --
1 | pack() | This geometry manager organizes widgets in blocks before placing them in the parent widget.
2 | grid() | This geometry manager organizes widgets in a table-like structure in the parent widget.
3 | place() | This geometry manager organizes widgets by placing them in a specific position in the parent widget.
place()
The Place geometry manager is the simplest of the three general geometry managers provided in Tkinter.
It allows you explicitly set the position and size of a window, either in absolute terms, or relative to another window.
https://www.geeksforgeeks.org/python-place-method-in-tkinter/
# Importing tkinter module
from tkinter import *
from tkinter.ttk import *
# creating Tk window
master = Tk()
# setting geometry of tk window
master.geometry("200x200")
# button widget
b2 = Button(master, text = "GFG")
b2.pack(fill = X, expand = True, ipady = 10)
# button widget
b1 = Button(master, text = "Click me !")
# This is where b1 is placed inside b2 with in_ option
b1.place(in_= b2, relx = 0.5, rely = 0.5, anchor = CENTER)
# label widget
l = Label(master, text = "I'm a Label")
l.place(anchor = NW)
# infinite loop which is required to
# run tkinter program infinitely
# until an interrupt occurs
mainloop()
grid()
The Grid geometry manager puts the widgets in a 2-dimensional table.
place()
The Place geometry manager is the simplest of the three general geometry managers provided in Tkinter. It allows you explicitly set the position and size of a window, either in absolute terms, or relative to another window. https://www.geeksforgeeks.org/python-place-method-in-tkinter/
grid()
The Grid geometry manager puts the widgets in a 2-dimensional table.
https://www.geeksforgeeks.org/python-grid-method-in-tkinter/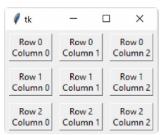
Pack()
The Pack geometry manager packs widgets relative to the earlier widget. https://www.geeksforgeeks.org/python-pack-method-in-tkinter/