Closed friuns2 closed 2 years ago
Dissonance isn't really designed to make this easy. Most of the complex things that Dissonance does for you are in the networking system, so I would really strongly advise to use that if at all possible!
If you do want to do this anyway, you can still build a custom network system for Dissonance but instead of using the base classes (BaseServer/BaseClient etc) you can instead implement ICommsNetwork
. You can implement this however you like and the rest of Dissonance will work as normal (e.g. you don't need to replace the microphone or playback systems). This isn't a use case I can provide much support for though.
It's been a while so I'll close this discussion, feel free to re-open it if you have more questions :)
Dissonance.zip Is it possible to use Dissonance without its networking? Just for encoding and decoding i want to send packets directly without additional Dissonance networking layer
I tried to make some loopback test, but get errors packet arrived is very late, Can you help me?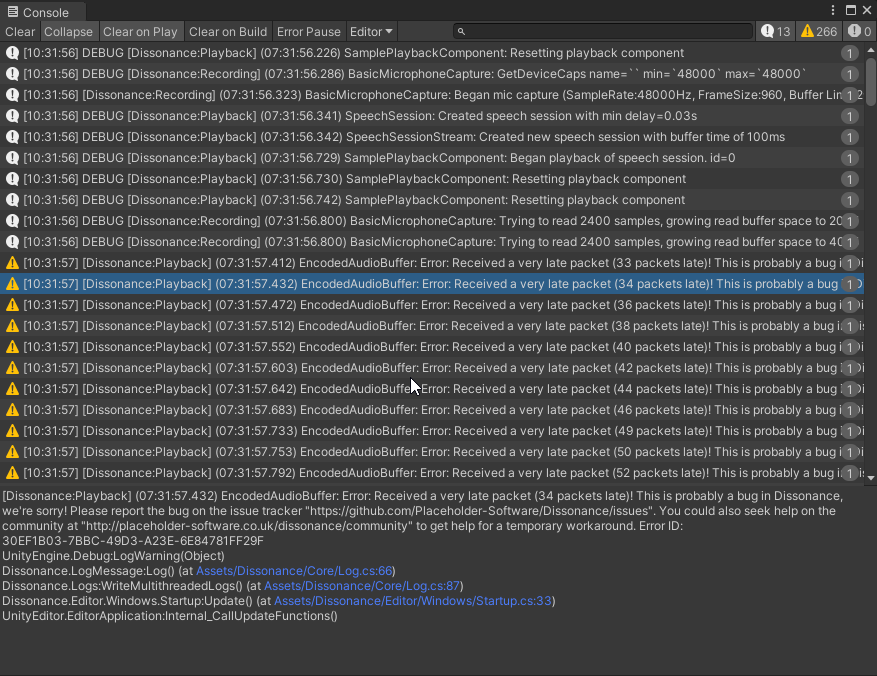