Closed sarjon closed 6 years ago
ping @mickaelandrieu
I've already done it => it's amazing how similar are our ideas, you're like a copy of me, but younger and with more muscles xD
Edit: can I adapt your suggestion ? I think adding a module loader can be a good addition too :)
/c @Quetzacoalt91
@sarjon this is the tree of my poc module
Did you have installed a bot to steal my work on my computer? xD
nice! :smile:
it would also be great if module controllers could use both annotations & yml for defining routes. :)
You don't even let me time to play LoL, I'll finish my PR right now.
It's really funny ^^ /c @toutantic
I'll finish my PR right now.
i cant wait for this feature to be available in core. :)
@sarjon I've ended up improving your proposal instead of working on annotations :trollface:
I have found a weird issue with annotations, and I need more time to work on supporting them in this context)
The support of annotations is not a blocking issue and can be introduced later => closed!
Thanks for your contribution @sarjon :)
@toutantic support of "modern/Symfony" controllers is now merged in develop
branch and will be shipped in 1.7.5.
Symfony is here, so why not allow module developers to make use of it and introduce symfony controllers for modules? I've been playing around with PrestaShop modules and i came up with this. Maybe it will give some ideas for future development. :)
First some changes to core
Create custom routes loader for modules and register it
I've implmented
PrestaShopBundle\Routing\ModuleRouteLoader
whichload()
methods looks like this, but could be improved by loading only installed modules.Register routes loader:
Finally, add new entry point for module routes:
Configure new path entry for twig templates so module templates can be rendered
Thats it for core changes.
A demo module
Here's how module directory structure looks like: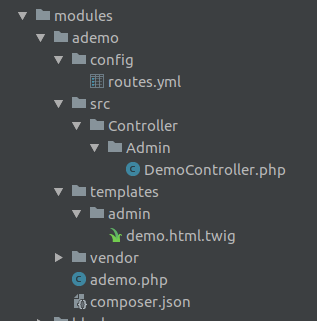
Routes file in module:
Module template file:
And finally a controller:
Now access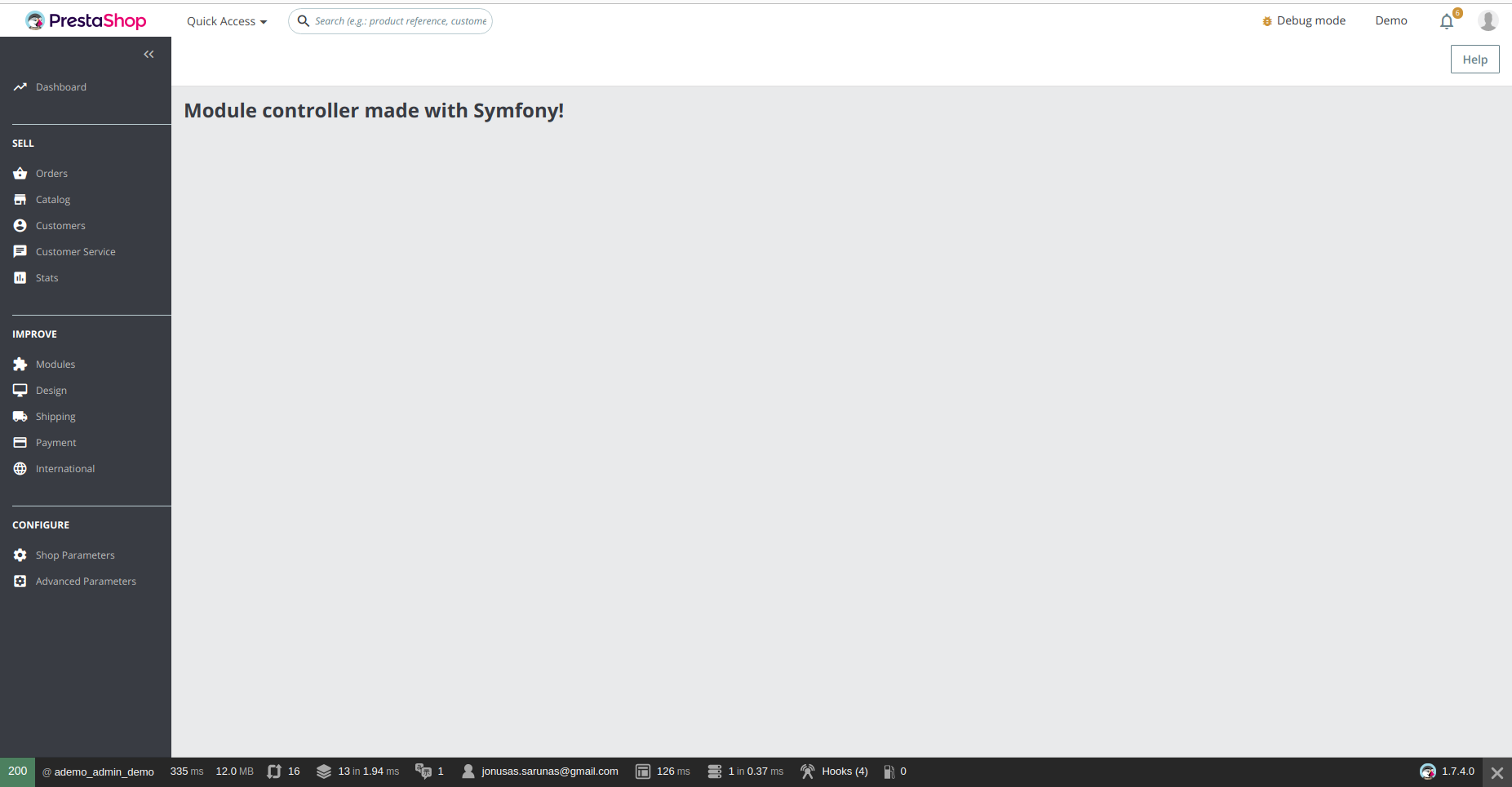
http://prestashop.local/admin-dev/index.php/modules/ademo/demo
and here we have page rendered with module using symfony controller!