I think most of the issues are arising when you try to get elements in pages like in the image
Line 82 causes an issue as we can see
It is trying to get classes in the cart page when we are in the product page and trying to add items to the cart.
Proposed Solutions
Changing the cart behaviour to be something like a popup which will be something that is present on all pages and not a page on its own
3. This code will go into the `addItemToCart()` function
```js
// use localstorage to add the item to the cart
function addItemToCart(title, price, imageSrc, quantity = 1) {
var alreadyInCart = false;
//check if item is present in the cart
var cartItemsOld = JSON.parse(localStorage.getItem("cartItems"));
//console.log("cart items old: ", cartItemsOld);
if (cartItemsOld != null && cartItemsOld.length > 0) {
for (var i = 0; i < cartItemsOld.length; i++) {
if (cartItemsOld[i].title == title) {
alert("Item already in cart! Increasing quantity by one.");
cartItemsOld[i].quantity += 1;
localStorage.setItem("cartItems", JSON.stringify(cartItemsOld));
alreadyInCart = true;
return;
}
}
}
if (alreadyInCart == false) {
cartItems.push({ title, price, imageSrc, quantity });
localStorage.setItem("cartItems", JSON.stringify(cartItems));
console.log("Updated cart: ", cartItems);
//do the dom manipulation after this to add the item to the cart
}
}
Now adding global code to detect which page the browser is in
if (window.location.pathname == "/cart"){
//do stuff
console.log("in cart.html");
addCartItemsToDOM();
}
function addCartItemsToDOM() {
var cartItems = JSON.parse(localStorage.getItem("cartItems"));
// console.log("cart items: ", cartItems);
for (var i = 0; i < cartItems.length; i++) {
var title = cartItems[i].title;
var price = cartItems[i].price;
var imageSrc = cartItems[i].imageSrc;
var quantity = cartItems[i].quantity;
console.log(title, price, imageSrc, quantity);
addCartItemsDivs(title, price, imageSrc, quantity);
}
}
function addCartItemsDivs(title, price, imageSrc, quantity) {
var cartRow = document.createElement("div");
cartRow.innerText = title;
cartRow.classList.add("cart-row");
var cartItems = document.getElementsByClassName("cart-items")[0];
var cartItemNames = cartItems.getElementsByClassName("cart-item-title");
var cartRowContents = `
<div class="cart-item cart-column">
<img class="cart-item-image" src="${imageSrc}" width="100" height="100">
<span class="cart-item-title">"${title}"</span>
</div>
<span class="cart-price cart-column"> "${price}"</span>
<div class="cart-quantity cart-column">
<input class = "cart-item-quantity" type = "number" value = ${quantity}>
<button class="btn-remove" type = "button">REMOVE</button>
</div>`;
cartRow.innerHTML = cartRowContents;
cartItems.appendChild(cartRow);
cartRow.getElementsByClassName("btn-remove")[0].addEventListener("click", removeCartItem);
// cartRow.getElementsByClassName("cart-item-quantity")[0].addEventListener("change", quantityChanged);
}
Now changing the updateCartTotal function to work with the new code
function updateCartTotal() {
var total = 0;
var cartItems = JSON.parse(localStorage.getItem("cartItems"));
for (var i = 0; i < cartItems.length; i++) {
var price = cartItems[i].price;
price = price.replace("₹", "");
var quantity = cartItems[i].quantity;
total = total + price * quantity;
}
total = Math.round(total * 100) / 100;
console.log("total: ", total);
document.getElementsByClassName("cart-total-price")[0].innerText = "₹ " + total;
// cartNumbers();
}
Now to change the code based on
localStorage.setItem("cartItems", JSON.stringify(cartItemsOld));
alreadyInCart = true;
return;
}
}
}
if (alreadyInCart == false) {
cartItems.push({ title, price, imageSrc, quantity });
localStorage.setItem("cartItems", JSON.stringify(cartItems));
console.log("Updated cart: ", cartItems);
//do the dom manipulation after this to add the item to the cart
}
}
7. Now adding global code to detect which page the browser is in
```js
if (window.location.pathname == "/cart"){
//do stuff
console.log("in cart.html");
addCartItemsToDOM();
}
function addCartItemsToDOM() {
var cartItems = JSON.parse(localStorage.getItem("cartItems"));
// console.log("cart items: ", cartItems);
for (var i = 0; i < cartItems.length; i++) {
var title = cartItems[i].title;
var price = cartItems[i].price;
var imageSrc = cartItems[i].imageSrc;
var quantity = cartItems[i].quantity;
console.log(title, price, imageSrc, quantity);
addCartItemsDivs(title, price, imageSrc, quantity);
}
}
function addCartItemsDivs(title, price, imageSrc, quantity) {
var cartRow = document.createElement("div");
cartRow.innerText = title;
cartRow.classList.add("cart-row");
var cartItems = document.getElementsByClassName("cart-items")[0];
var cartItemNames = cartItems.getElementsByClassName("cart-item-title");
var cartRowContents = `
<div class="cart-item cart-column">
<img class="cart-item-image" src="${imageSrc}" width="100" height="100">
<span class="cart-item-title">"${title}"</span>
</div>
<span class="cart-price cart-column"> "${price}"</span>
<div class="cart-quantity cart-column">
<input class = "cart-item-quantity" type = "number" value = ${quantity}>
<button class="btn-remove" type = "button">REMOVE</button>
</div>`;
cartRow.innerHTML = cartRowContents;
cartItems.appendChild(cartRow);
cartRow.getElementsByClassName("btn-remove")[0].addEventListener("click", removeCartItem);
cartRow.getElementsByClassName("cart-item-quantity")[0].addEventListener("change", quantityChanged);
}
Now changing the updateCartTotal() function to work with the new code
function updateCartTotal() {
var total = 0;
var cartItems = JSON.parse(localStorage.getItem("cartItems"));
for (var i = 0; i < cartItems.length; i++) {
var price = cartItems[i].price;
price = price.replace("₹", "");
var quantity = cartItems[i].quantity;
total = total + price * quantity;
}
total = Math.round(total * 100) / 100;
console.log("total: ", total);
if(window.location.pathname == "/cart")
{
document.getElementsByClassName("cart-total-price")[0].innerText = "₹ " + total;
}
localStorage.setItem("total", total);
// cartNumbers();
}
Now to change the code based on LocalStorage for removeCartItem() function
function removeCartItem(event) {
var buttonClicked = event.target;
var cartItems = JSON.parse(localStorage.getItem("cartItems"));
var title = buttonClicked.parentElement.parentElement.getElementsByClassName("cart-item-title")[0].innerText;
title = title.replaceAll('"', "");
// console.log("parent title", title);
for (var i = 0; i < cartItems.length; i++) {
if (cartItems[i].title == title) {
cartItems.splice(i, 1);
localStorage.setItem("cartItems", JSON.stringify(cartItems));
console.log("Updated cart: (removed)", cartItems);
}
}
buttonClicked.parentElement.parentElement.remove();
updateCartTotal();
}
Now to change the code based on LocalStorage for quantityChanged() function
function quantityChanged(event) {
var input = event.target;
var cartItems = JSON.parse(localStorage.getItem("cartItems"));
var title = input.parentElement.parentElement.getElementsByClassName("cart-item-title")[0].innerText;
title = title.replaceAll('"', "");
// console.log("parent title", title);
for (var i = 0; i < cartItems.length; i++) {
if (cartItems[i].title == title) {
cartItems[i].quantity = input.value;
localStorage.setItem("cartItems", JSON.stringify(cartItems));
console.log("Updated cart (quantity changed) : ", cartItems);
}
}
if (isNaN(input.value) || input.value <= 0) {
input.value = 1;
}
updateCartTotal();
}
Finally modify on purchase to clear the items present in localStorage and update the div
function purchaseClicked(event) {
var cartItemsDiv = document.getElementsByClassName("cart-items")[0];
cartItems = JSON.parse(localStorage.getItem("cartItems"));
if (cartItems.length > 0) {
alert("Thank you for your purchase of " + cartItems.length + " items. Total amount: " + localStorage.getItem("total") + "");
cartItems = [];
localStorage.setItem("cartItems", JSON.stringify(cartItems));
cartItemsDiv.innerHTML = "";
updateCartTotal();
} else {
alert("⚠️ Please choose items to purchase!");
}
}
Issue at hand
I think most of the issues are arising when you try to get elements in pages like in the image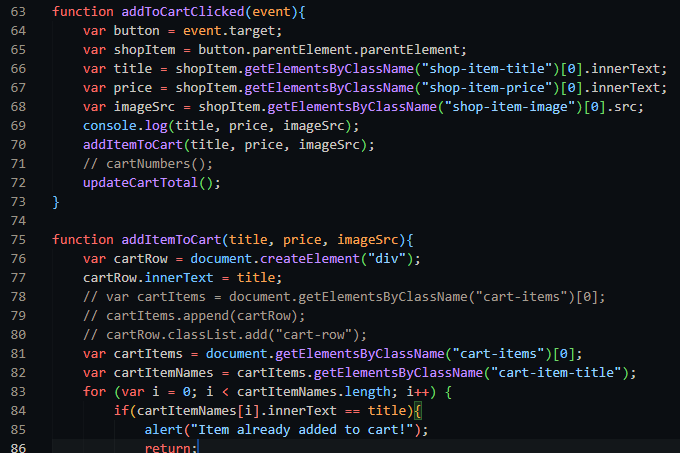
Line 82 causes an issue as we can see
It is trying to get classes in the cart page when we are in the product page and trying to add items to the cart.
Proposed Solutions
Implementation of LocalStorage Concept Code
I will focus on the LocalStorage Implementation
Steps :
ready()
functioncartItems = localStorage.getItem("cartItems", JSON.stringify(cartItems)); if (cartItems == null) { cartItems = []; localStorage.setItem("cartItems", JSON.stringify(cartItems)); } console.log("Initial Cart on Load: ", cartItems);
Now adding global code to detect which page the browser is in
Now changing the
updateCartTotal
function to work with the new codeNow to change the code based on
Now changing the
updateCartTotal()
function to work with the new codeNow to change the code based on LocalStorage for
removeCartItem()
functionNow to change the code based on LocalStorage for
quantityChanged()
functionFinally modify on purchase to clear the items present in localStorage and update the div