Open Serock3 opened 3 years ago
CommutativeCancellation was designed to be used in a fixed gate set [H, X, Y, Z, CX, CY, CZ]. It's indeed a bug. Its behavior should be either (1) flag that the above circuit is not supported or (2) add support to the above circuit. Will try to fix it.
Information
What is the current behavior?
The CommutativeCancellation pass modifies some circuits in an incorrect way, which can change both state vector snapshots and measurement outcomes. This happens also when using the transpiler with
optimization_level=2
or above, as the pass is then applied.Steps to reproduce the problem
This circuit seems a bit arbitrary, but it the smallest one that I have found that reproduces the bug.
Output: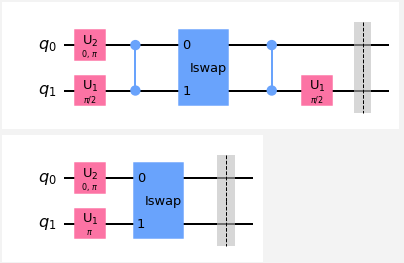
The pass gives an unwanted phase in the |10> state. Note that the iSWAP and CZ gates commute, and so the CZs really cancel. If you remove them (or any other gate), the bug stops occurring.
The effect is more pronounced if you add the inverse of the above circuit and measurements. The opposite bug happens to the inverse circuit which causes the state to become |01> instead of |00>. If you replace
circuit.snapshot('middle', snapshot_type=snapshot_type)
withAnd print the states and measurement counts, you get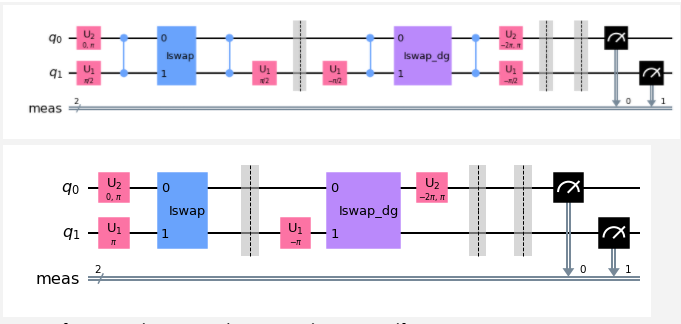
Note how this now even affects the measurement outcomes.
Note also that the CommutativeCancellation pass requires CommutationAnalysis, so the bug may lie in either one. CommutationAnalysis "determines commutativity through matrix multiplication", so if this happens for the iSWAP gate it can probably happen on other ones too.
What is the expected behavior?
Transpilation should not change the behavior of the circuit (except for perhaps commuting the qubit ordering, but that's not relevant here), especially not measurements.
Suggested solutions
I don't know enough about the operation of these passes to have found a fix.