Open HackyDev opened 1 year ago
That looks promising! They make smaller relays, but the mechanical output will be weaker. You might get a stronger output by finding a low voltage relay, say 3V (I don't know if these exist, I've only seen 5V), then drive them at a higher voltage. If you're driving them directly and not using the contacts, you can try a mechanical buzzer, which is essentially a relay wired to oscillate, connected to a large diaphragm. Those might have even more powerful coils, I'm excited to hear how these work.
Hello, sorry for intruding but this is an area of deep interest for me - my wife has early onset PD. I am an SDE and EE. Very familiar with design and manufacture of electronic circuits. I have a small business designing and selling circuit boards for the CNC marketplace.
The first thing that struck me is the use of relay coils to make the actuators. Coil winding is not hard, no need to crack open cheap relays. Plus the coil can be made to meet the exact needs - though, the hard part is knowing exactly what the needs are. That's not my area of expertise unfortunately. The relay coils look pretty big, I would think lower profile ones would fit better in the gloves. A coil winding machine is pretty easy to build, ham radio people have been doing it for about 100 years now. Also, the relay coils probably draw a lot of current (likely 70 mA for the 5V ones), especially when multiplied by 8. I assume the gloves are battery powered so power consumption is probably a big deal. Not sure if much lower current draw is even possible, though. I suspect that's why there seems to be a lot interest in piezo actuators.
For driving the coils, the uln2803 is ok but you might want to consider using mosfet H-bridges. The uln2803 is pretty old and the new mosfet drivers are much more efficient, important for battery power. An example is the DMHC3025, it comes in a small 8 pin surface mount package and could be mounted right at the coil. It costs about $0.70 each so <$5.00. I'm sure there are even smaller packages.
Anyway, just wanted to chime in to say I'm following and am interested in building/testing/experimenting on this front.
Phil Barrett
By the way, have you thought about turning on the discussion feature? Typically on github, issues are where people report bugs. The discussions section is where a lot of people do brainstorming.
@phil-barrett, Hey! Thank you for the tips. Additionally, there are these tiny audio exciters available, such as the Tectonic TEAX09C005-8. Although I've never worked with them, I believe they would be perfect for this application and could save a lot of time for DIYers. Anyway, I've managed to assemble a working prototype using this approach. It was a real pain to put everything together though. If you're curious here's the repo
I looked at the TEAX09C005-8 and it appears to be driven like a speaker. If it works well, then great! But, after reading a bit more, I wonder if a tiny solenoid is a good fit for this application. I'm using quotes because I think my terminology is wrong for the application space.
I'm very interested to see how your proto works. Basically, it uses the solenoid approach. While I get it's a pain to use the coils, I'd like to try winding my own. I need to bone up on solenoid construction though.
Hey!
I'm here to report some findings on the use of electromagnets. I was able to achieve 250Hz frequency and a rise time of 10 - 20ms using an electromagnet from a simple arduino relay and a neodimium magnet. I measured the frequency by pressing the contactor against the microphone and using this service to detect the pitch. The vibrations are very mild, but noticeable. The exact amplitude is not known, because I have no idea how to measure it yet. I'm sure the rise time can be improved using other electromagnets/code/etc.
Here's the representation of the concept.
Here's a relay.
Here's a relay without latching mechanism and the casing.
Here's new casing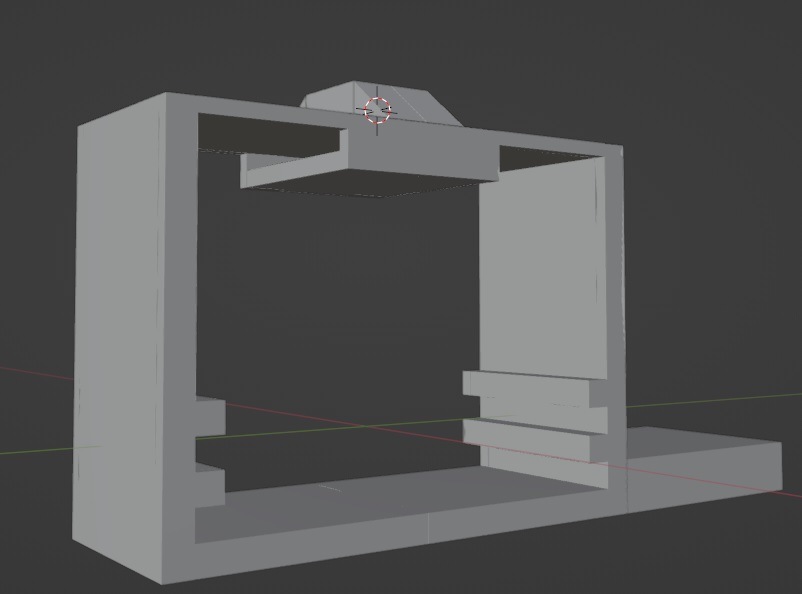
Here's its all together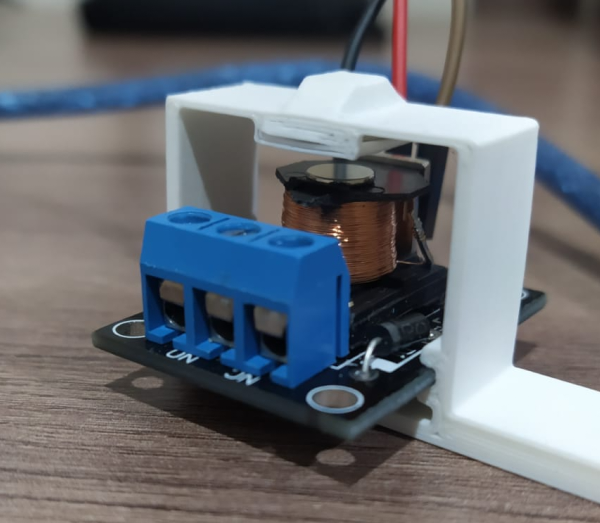
Here's Arduino code:
Here's the rise time (screenshot from audio editor)