Closed Thomas-de-Bock closed 1 year ago
The purpose of the collision plane (the manifold) is to define a plane of contact to push the shapes apart along its normal vector. This normal describes the axis of minimum translation to separate the shapes.
We could represent the contact by points along the intersection of edges, but this wouldn’t provide the best results for a collision solver.
We can calculate the depth of the collision by calculating the distance of each point along the normal vector until separation. The depth is already given to you in the manifold struct.
If you’d like to read more about this kind of algorithm there are comments near the bottom of cute_c2.h describing the algorithm and reading materials.
I’m going to close this issue, but you can of course comment here further if you have more questions or want to discuss more.
Sorry for responding so late, I read it and I think I understand the point of the returned values now, thanks. Though I am curious to know if there is any way of getting the points of intersection in the c2 header as I need it for calculating certain forces in my engine.
I’m quite suspicious that you don’t need these intersection points, and would like to learn more about your use case to try and help verify what you need and don’t need. The most difficult part of game physics is understanding the problem context and then selecting good algorithms.
Sutherland hodgman clipping would be the algorithm to collect your intersection points. If you like I can provide you with a 2D implementation in C.
Here you go: https://gist.github.com/RandyGaul/8b9c3f3724ea34959586205220be1da3
Though note, if you want edge-edge intersections you'll have to deal with cases like this:
As not all the cases have a nice and simple 2 collision points like this case:
That's perfect, thanks so much!
So I'm using this code to get the result between two square polies (I know I shouldnt be using polytopoly for squares but this is for testing).
and print out the points of intersection and vertices, what this prints out in my case is:
these values are all in line with what they should be in my program, but the contact points seem weird to me. I'm using my own rendering code which is still very barebones so to make it clear I plotted the points here: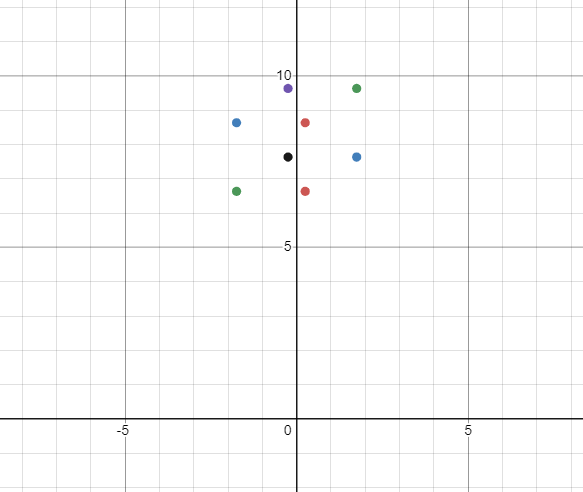
these are the points of the polygons, but when I also plot the contact points, specified with the arrows (one of the points is on the same position as one of the vertices of the right square):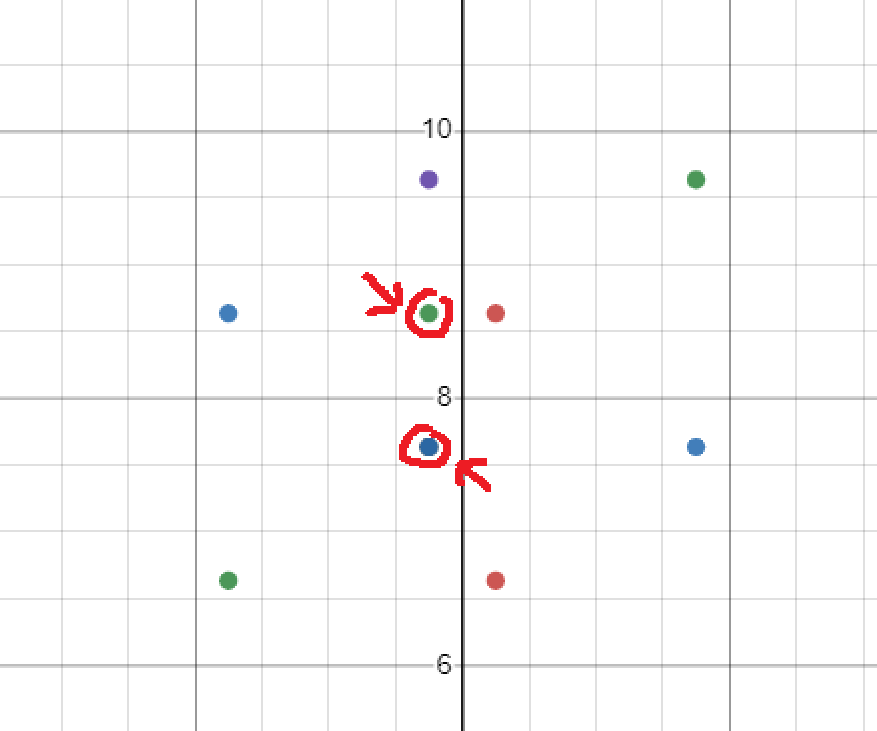
If I'm not mistaken about the definition of contact points in this case shouldn't they be placed here?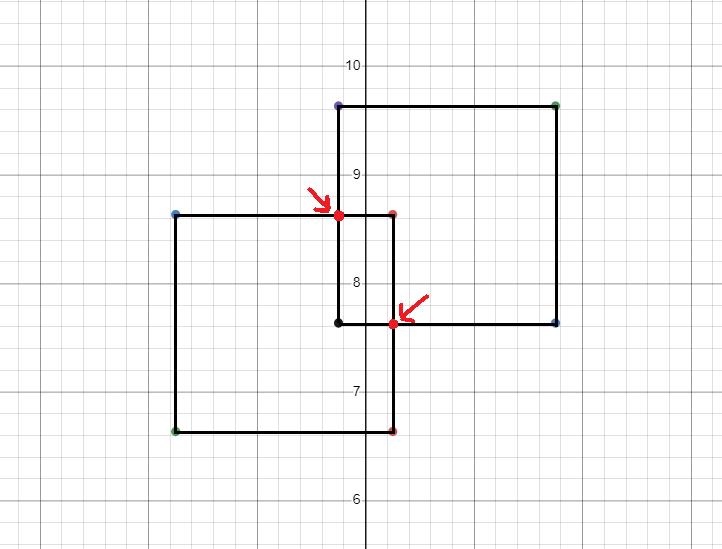
as that is where the lines intersect?
I create the polygons with:
(WorldParticles just contains the world positions of the vertices of the current shape)