Closed UCDFiddes closed 2 years ago
const p1 = memoryjs.readMemory(processObject.handle, process.modBaseAddr + 0x41F8798, memoryjs.PTR);
// p1 = 0x1DBCCBCD730
const p2 = memoryjs.readMemory(processObject.handle, p1 + 0x0, memoryjs.PTR);
// p2 = 0x1DBCCB70C80
const p3 = memoryjs.readMemory(processObject.handle, p2 + 0x20, memoryjs.PTR);
// p3 = 0x1DC070CF3B8
// ...etc
It would be a good idea to not use process
as the name of your memoryjs.openProcess
variable as it is a reserved global variable in Node.js
Closing, comment if you still need help :)
Opening because others may find this stuff below helpful. If you're looking to recreate the Cheat Engine pointer experience then you can reduce down an array of offsets instead of potentially writing a ton of lines and getting lost.
Is this something that could fit the examples @Rob-- ?
const getValueWithOffsets = (address, handle, offsets, type) => {
return offsets.reduce((a, b, index, array) => {
const value = memoryjs.readMemory(handle, a + b, memoryjs.PTR)
if (index === array.length - 1) {
return memoryjs.readMemory(handle, a + b, type) // If we're at the last offset, we don't want to read it's value as a pointer, but rather the type it should be, instead. If you want the end address instead of the value (then return a + b)
}
return value
}, address)
}
Using the above Minecraft example, it'd look something like this.
const processName = 'Minecraft.Windows.exe'
const game = memoryjs.openProcess(processName)
const OFFSETS = [0x0, 0x20, 0x8, 0x10, 0x268, 0x128, 0x9A4] // Your array of offsets. (0x... notates that we're writing hexadecimal. Start from the bottom of the offset in Cheat Engine)
let result = getValueWithOffsets(
game.modBaseAddr + 0x41F8798, // Minecraft.Windows.exe + 0x41F8798
game.handle,
OFFSETS,
memoryjs.BYTE, // The end type of the value needed.
)
console.log(result) // 0. The value of the end address, as a byte.
I hope this helps!
Hi Rob,
I apologize in advance for commenting on an already closed issue, but I've been at this for hours and have hit a hard wall. I was able to read from cheat engine the values I want at a particular address just fine, but I'm having issues with the pointers in my code. As you can see in the GE screenshot, I am able to get float I want, but in my console screenshot from node, I am getting something totally different. pointerChain is the method in question that is being called from another file. Any help would be greatly appreciated :)
Please see below for reference:
Hi Rob,
I apologize in advance for commenting on an already closed issue, but I've been at this for hours and have hit a hard wall. I was able to read from cheat engine the values I want at a particular address just fine, but I'm having issues with the pointers in my code. As you can see in the GE screenshot, I am able to get float I want, but in my console screenshot from node, I am getting something totally different. pointerChain is the method in question that is being called from another file. Any help would be greatly appreciated :)
Please see below for reference:
![]()
![]()
![]()
Try setting the type of p8 to float instead of ptr See my example above, where the last offset isn't declared as a pointer, but rather the type we're looking for.
Opening because others may find this stuff below helpful. If you're looking to recreate the Cheat Engine pointer experience then you can reduce down an array of offsets instead of potentially writing a ton of lines and getting lost.
Is this something that could fit the examples @Rob-- ?
const getValueWithOffsets = (address, handle, offsets, type) => { return offsets.reduce((a, b, index, array) => { const value = memoryjs.readMemory(handle, a + b, memoryjs.PTR) if (index === array.length - 1) { return memoryjs.readMemory(handle, a + b, type) // If we're at the last offset, we don't want to read it's value as a pointer, but rather the type it should be, instead. If you want the end address instead of the value (then return a + b) } return value }, address) }
Using the above Minecraft example, it'd look something like this.
const processName = 'Minecraft.Windows.exe' const game = memoryjs.openProcess(processName) const OFFSETS = [0x0, 0x20, 0x8, 0x10, 0x268, 0x128, 0x9A4] // Your array of offsets. (0x... notates that we're writing hexadecimal. Start from the bottom of the offset in Cheat Engine) let result = getValueWithOffsets( game.modBaseAddr + 0x41F8798, // Minecraft.Windows.exe + 0x41F8798 game.handle, OFFSETS, memoryjs.BYTE, // The end type of the value needed. ) console.log(result) // 0. The value of the end address, as a byte.
I hope this helps!
This didn't work but this refactor did.
const getValueWithOffsets = (handle, offsets, resultType) => {
let pointer = null;
for (let i = 0; i < offsets.length; i++) {
let isLast = i === offsets.length - 1,
offset = offsets[i];
if (isLast) {
return memoryjs.readMemory(handle, Number(pointer) + offset, resultType);
} else {
if (pointer === null) {
pointer = memoryjs.readMemory(handle, offset, memoryjs.PTR);
} else {
pointer = memoryjs.readMemory(handle, Number(pointer) + offset, memoryjs.PTR);
}
}
}
}
const hp = getValueWithOffsets(
trose.handle,
[trose.modBaseAddr + 0x0154EE60, 0x3B90],
memoryjs.INT32, // The end type of the value needed.
)
May someone teach me how to read this pointer using memoryjs. Ive tried things but it just wont work.
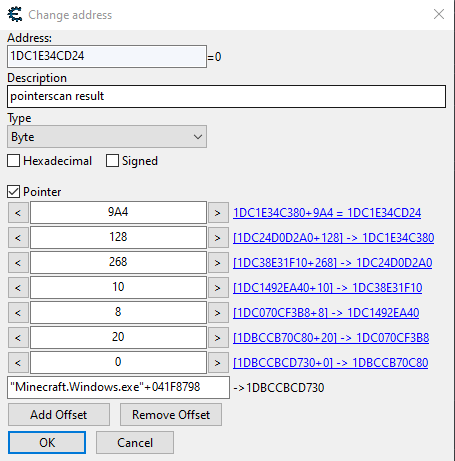